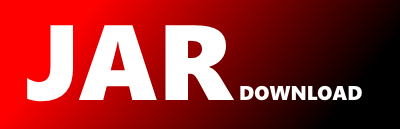
org.hyperic.sigar.ProcState Maven / Gradle / Ivy
/*****************************************************
* WARNING: this file was generated by -e
* on Mon May 24 16:26:06 2010.
* Any changes made here will be LOST.
*****************************************************/
package org.hyperic.sigar;
import java.util.HashMap;
import java.util.Map;
/**
* ProcState sigar class.
*/
public class ProcState implements java.io.Serializable {
private static final long serialVersionUID = 7805L;
public ProcState() { }
public native void gather(Sigar sigar, long pid) throws SigarException;
/**
* This method is not intended to be called directly.
* use Sigar.getProcState() instead.
* @exception SigarException on failure.
* @see org.hyperic.sigar.Sigar#getProcState
*/
static ProcState fetch(Sigar sigar, long pid) throws SigarException {
ProcState procState = new ProcState();
procState.gather(sigar, pid);
return procState;
}
char state = 0;
/**
* Get the Process state (Running, Zombie, etc.).
* Supported Platforms: All.
*
* System equivalent commands:
* - AIX:
top, ps
* - Darwin:
top, ps
* - FreeBSD:
top, ps
* - HPUX:
top, ps
* - Linux:
top, ps
* - Solaris:
top, ps
* - Win32:
taskman
*
* @return Process state (Running, Zombie, etc.)
*/
public char getState() { return state; }
String name = null;
/**
* Get the Name of the process program.
* Supported Platforms: All.
*
* System equivalent commands:
* - AIX:
top, ps
* - Darwin:
top, ps
* - FreeBSD:
top, ps
* - HPUX:
top, ps
* - Linux:
top, ps
* - Solaris:
top, ps
* - Win32:
taskman
*
* @return Name of the process program
*/
public String getName() { return name; }
long ppid = 0;
/**
* Get the Process parent process id.
* Supported Platforms: All.
*
* System equivalent commands:
* - AIX:
top, ps
* - Darwin:
top, ps
* - FreeBSD:
top, ps
* - HPUX:
top, ps
* - Linux:
top, ps
* - Solaris:
top, ps
* - Win32:
taskman
*
* @return Process parent process id
*/
public long getPpid() { return ppid; }
int tty = 0;
/**
* Get the Device number of rocess controling terminal.
* Supported Platforms: AIX, HPUX, Linux, Solaris.
*
* System equivalent commands:
* - AIX:
top, ps
* - Darwin:
top, ps
* - FreeBSD:
top, ps
* - HPUX:
top, ps
* - Linux:
top, ps
* - Solaris:
top, ps
* - Win32:
taskman
*
* @return Device number of rocess controling terminal
*/
public int getTty() { return tty; }
int nice = 0;
/**
* Get the Nice value of process.
* Supported Platforms: AIX, Darwin, FreeBSD, HPUX, Linux, Solaris.
*
* System equivalent commands:
* - AIX:
top, ps
* - Darwin:
top, ps
* - FreeBSD:
top, ps
* - HPUX:
top, ps
* - Linux:
top, ps
* - Solaris:
top, ps
* - Win32:
taskman
*
* @return Nice value of process
*/
public int getNice() { return nice; }
int priority = 0;
/**
* Get the Kernel scheduling priority of process.
* Supported Platforms: Darwin, FreeBSD, HPUX, Linux, Solaris, Win32.
*
* System equivalent commands:
* - AIX:
top, ps
* - Darwin:
top, ps
* - FreeBSD:
top, ps
* - HPUX:
top, ps
* - Linux:
top, ps
* - Solaris:
top, ps
* - Win32:
taskman
*
* @return Kernel scheduling priority of process
*/
public int getPriority() { return priority; }
long threads = 0;
/**
* Get the Number of active threads.
* Supported Platforms: AIX, Darwin, HPUX, Linux, Solaris, Win32.
*
* System equivalent commands:
* - AIX:
top, ps
* - Darwin:
top, ps
* - FreeBSD:
top, ps
* - HPUX:
top, ps
* - Linux:
top, ps
* - Solaris:
top, ps
* - Win32:
taskman
*
* @return Number of active threads
*/
public long getThreads() { return threads; }
int processor = 0;
/**
* Get the Processor number last run on.
* Supported Platforms: AIX, HPUX, Linux, Solaris.
*
* System equivalent commands:
* - AIX:
top, ps
* - Darwin:
top, ps
* - FreeBSD:
top, ps
* - HPUX:
top, ps
* - Linux:
top, ps
* - Solaris:
top, ps
* - Win32:
taskman
*
* @return Processor number last run on
*/
public int getProcessor() { return processor; }
void copyTo(ProcState copy) {
copy.state = this.state;
copy.name = this.name;
copy.ppid = this.ppid;
copy.tty = this.tty;
copy.nice = this.nice;
copy.priority = this.priority;
copy.threads = this.threads;
copy.processor = this.processor;
}
public static final char SLEEP = 'S';
public static final char RUN = 'R';
public static final char STOP = 'T';
public static final char ZOMBIE = 'Z';
public static final char IDLE = 'D';
public Map toMap() {
Map map = new HashMap();
String strstate =
String.valueOf(this.state);
if (!"-1".equals(strstate))
map.put("State", strstate);
String strname =
String.valueOf(this.name);
if (!"-1".equals(strname))
map.put("Name", strname);
String strppid =
String.valueOf(this.ppid);
if (!"-1".equals(strppid))
map.put("Ppid", strppid);
String strtty =
String.valueOf(this.tty);
if (!"-1".equals(strtty))
map.put("Tty", strtty);
String strnice =
String.valueOf(this.nice);
if (!"-1".equals(strnice))
map.put("Nice", strnice);
String strpriority =
String.valueOf(this.priority);
if (!"-1".equals(strpriority))
map.put("Priority", strpriority);
String strthreads =
String.valueOf(this.threads);
if (!"-1".equals(strthreads))
map.put("Threads", strthreads);
String strprocessor =
String.valueOf(this.processor);
if (!"-1".equals(strprocessor))
map.put("Processor", strprocessor);
return map;
}
public String toString() {
return toMap().toString();
}
}