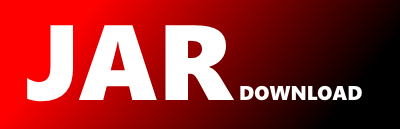
org.hyperic.sigar.ResourceLimit Maven / Gradle / Ivy
/*****************************************************
* WARNING: this file was generated by -e
* on Mon May 24 16:26:06 2010.
* Any changes made here will be LOST.
*****************************************************/
package org.hyperic.sigar;
import java.util.HashMap;
import java.util.Map;
/**
* ResourceLimit sigar class.
*/
public class ResourceLimit implements java.io.Serializable {
private static final long serialVersionUID = 32184L;
public ResourceLimit() { }
public native void gather(Sigar sigar) throws SigarException;
/**
* This method is not intended to be called directly.
* use Sigar.getResourceLimit() instead.
* @exception SigarException on failure.
* @see org.hyperic.sigar.Sigar#getResourceLimit
*/
static ResourceLimit fetch(Sigar sigar) throws SigarException {
ResourceLimit resourceLimit = new ResourceLimit();
resourceLimit.gather(sigar);
return resourceLimit;
}
long cpuCur = 0;
/**
* Get the cpu_cur.
* Supported Platforms: Undocumented.
*
* @return cpu_cur
*/
public long getCpuCur() { return cpuCur; }
long cpuMax = 0;
/**
* Get the cpu_max.
* Supported Platforms: Undocumented.
*
* @return cpu_max
*/
public long getCpuMax() { return cpuMax; }
long fileSizeCur = 0;
/**
* Get the file_size_cur.
* Supported Platforms: Undocumented.
*
* @return file_size_cur
*/
public long getFileSizeCur() { return fileSizeCur; }
long fileSizeMax = 0;
/**
* Get the file_size_max.
* Supported Platforms: Undocumented.
*
* @return file_size_max
*/
public long getFileSizeMax() { return fileSizeMax; }
long pipeSizeMax = 0;
/**
* Get the pipe_size_max.
* Supported Platforms: Undocumented.
*
* @return pipe_size_max
*/
public long getPipeSizeMax() { return pipeSizeMax; }
long pipeSizeCur = 0;
/**
* Get the pipe_size_cur.
* Supported Platforms: Undocumented.
*
* @return pipe_size_cur
*/
public long getPipeSizeCur() { return pipeSizeCur; }
long dataCur = 0;
/**
* Get the data_cur.
* Supported Platforms: Undocumented.
*
* @return data_cur
*/
public long getDataCur() { return dataCur; }
long dataMax = 0;
/**
* Get the data_max.
* Supported Platforms: Undocumented.
*
* @return data_max
*/
public long getDataMax() { return dataMax; }
long stackCur = 0;
/**
* Get the stack_cur.
* Supported Platforms: Undocumented.
*
* @return stack_cur
*/
public long getStackCur() { return stackCur; }
long stackMax = 0;
/**
* Get the stack_max.
* Supported Platforms: Undocumented.
*
* @return stack_max
*/
public long getStackMax() { return stackMax; }
long coreCur = 0;
/**
* Get the core_cur.
* Supported Platforms: Undocumented.
*
* @return core_cur
*/
public long getCoreCur() { return coreCur; }
long coreMax = 0;
/**
* Get the core_max.
* Supported Platforms: Undocumented.
*
* @return core_max
*/
public long getCoreMax() { return coreMax; }
long memoryCur = 0;
/**
* Get the memory_cur.
* Supported Platforms: Undocumented.
*
* @return memory_cur
*/
public long getMemoryCur() { return memoryCur; }
long memoryMax = 0;
/**
* Get the memory_max.
* Supported Platforms: Undocumented.
*
* @return memory_max
*/
public long getMemoryMax() { return memoryMax; }
long processesCur = 0;
/**
* Get the processes_cur.
* Supported Platforms: Undocumented.
*
* @return processes_cur
*/
public long getProcessesCur() { return processesCur; }
long processesMax = 0;
/**
* Get the processes_max.
* Supported Platforms: Undocumented.
*
* @return processes_max
*/
public long getProcessesMax() { return processesMax; }
long openFilesCur = 0;
/**
* Get the open_files_cur.
* Supported Platforms: Undocumented.
*
* @return open_files_cur
*/
public long getOpenFilesCur() { return openFilesCur; }
long openFilesMax = 0;
/**
* Get the open_files_max.
* Supported Platforms: Undocumented.
*
* @return open_files_max
*/
public long getOpenFilesMax() { return openFilesMax; }
long virtualMemoryCur = 0;
/**
* Get the virtual_memory_cur.
* Supported Platforms: Undocumented.
*
* @return virtual_memory_cur
*/
public long getVirtualMemoryCur() { return virtualMemoryCur; }
long virtualMemoryMax = 0;
/**
* Get the virtual_memory_max.
* Supported Platforms: Undocumented.
*
* @return virtual_memory_max
*/
public long getVirtualMemoryMax() { return virtualMemoryMax; }
void copyTo(ResourceLimit copy) {
copy.cpuCur = this.cpuCur;
copy.cpuMax = this.cpuMax;
copy.fileSizeCur = this.fileSizeCur;
copy.fileSizeMax = this.fileSizeMax;
copy.pipeSizeMax = this.pipeSizeMax;
copy.pipeSizeCur = this.pipeSizeCur;
copy.dataCur = this.dataCur;
copy.dataMax = this.dataMax;
copy.stackCur = this.stackCur;
copy.stackMax = this.stackMax;
copy.coreCur = this.coreCur;
copy.coreMax = this.coreMax;
copy.memoryCur = this.memoryCur;
copy.memoryMax = this.memoryMax;
copy.processesCur = this.processesCur;
copy.processesMax = this.processesMax;
copy.openFilesCur = this.openFilesCur;
copy.openFilesMax = this.openFilesMax;
copy.virtualMemoryCur = this.virtualMemoryCur;
copy.virtualMemoryMax = this.virtualMemoryMax;
}
public static native long INFINITY();
public Map toMap() {
Map map = new HashMap();
String strcpuCur =
String.valueOf(this.cpuCur);
if (!"-1".equals(strcpuCur))
map.put("CpuCur", strcpuCur);
String strcpuMax =
String.valueOf(this.cpuMax);
if (!"-1".equals(strcpuMax))
map.put("CpuMax", strcpuMax);
String strfileSizeCur =
String.valueOf(this.fileSizeCur);
if (!"-1".equals(strfileSizeCur))
map.put("FileSizeCur", strfileSizeCur);
String strfileSizeMax =
String.valueOf(this.fileSizeMax);
if (!"-1".equals(strfileSizeMax))
map.put("FileSizeMax", strfileSizeMax);
String strpipeSizeMax =
String.valueOf(this.pipeSizeMax);
if (!"-1".equals(strpipeSizeMax))
map.put("PipeSizeMax", strpipeSizeMax);
String strpipeSizeCur =
String.valueOf(this.pipeSizeCur);
if (!"-1".equals(strpipeSizeCur))
map.put("PipeSizeCur", strpipeSizeCur);
String strdataCur =
String.valueOf(this.dataCur);
if (!"-1".equals(strdataCur))
map.put("DataCur", strdataCur);
String strdataMax =
String.valueOf(this.dataMax);
if (!"-1".equals(strdataMax))
map.put("DataMax", strdataMax);
String strstackCur =
String.valueOf(this.stackCur);
if (!"-1".equals(strstackCur))
map.put("StackCur", strstackCur);
String strstackMax =
String.valueOf(this.stackMax);
if (!"-1".equals(strstackMax))
map.put("StackMax", strstackMax);
String strcoreCur =
String.valueOf(this.coreCur);
if (!"-1".equals(strcoreCur))
map.put("CoreCur", strcoreCur);
String strcoreMax =
String.valueOf(this.coreMax);
if (!"-1".equals(strcoreMax))
map.put("CoreMax", strcoreMax);
String strmemoryCur =
String.valueOf(this.memoryCur);
if (!"-1".equals(strmemoryCur))
map.put("MemoryCur", strmemoryCur);
String strmemoryMax =
String.valueOf(this.memoryMax);
if (!"-1".equals(strmemoryMax))
map.put("MemoryMax", strmemoryMax);
String strprocessesCur =
String.valueOf(this.processesCur);
if (!"-1".equals(strprocessesCur))
map.put("ProcessesCur", strprocessesCur);
String strprocessesMax =
String.valueOf(this.processesMax);
if (!"-1".equals(strprocessesMax))
map.put("ProcessesMax", strprocessesMax);
String stropenFilesCur =
String.valueOf(this.openFilesCur);
if (!"-1".equals(stropenFilesCur))
map.put("OpenFilesCur", stropenFilesCur);
String stropenFilesMax =
String.valueOf(this.openFilesMax);
if (!"-1".equals(stropenFilesMax))
map.put("OpenFilesMax", stropenFilesMax);
String strvirtualMemoryCur =
String.valueOf(this.virtualMemoryCur);
if (!"-1".equals(strvirtualMemoryCur))
map.put("VirtualMemoryCur", strvirtualMemoryCur);
String strvirtualMemoryMax =
String.valueOf(this.virtualMemoryMax);
if (!"-1".equals(strvirtualMemoryMax))
map.put("VirtualMemoryMax", strvirtualMemoryMax);
return map;
}
public String toString() {
return toMap().toString();
}
}