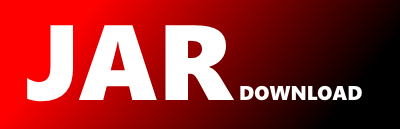
org.hyperic.sigar.Sigar Maven / Gradle / Ivy
/*
* Copyright (c) 2006-2008 Hyperic, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.hyperic.sigar;
import java.io.BufferedReader;
import java.io.File;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.List;
import java.util.Map;
import java.util.StringTokenizer;
import org.hyperic.jni.ArchLoaderException;
import org.hyperic.jni.ArchNotSupportedException;
import org.hyperic.sigar.ptql.ProcessFinder;
/**
* The Sigar class provides access to the sigar objects containing
* system information. The Sigar object itself maintains internal
* state specific to each platform. It also implements the SigarProxy
* interface which provides caching at the Java level.
*/
public class Sigar implements SigarProxy {
private static String loadError = null;
public static final long FIELD_NOTIMPL = -1;
/**
* The Sigar java version.
*/
public static final String VERSION_STRING =
SigarVersion.VERSION_STRING;
/**
* The Sigar native version.
*/
public static final String NATIVE_VERSION_STRING;
/**
* The scm (svn) revision from which sigar.jar was built.
*/
public static final String SCM_REVISION =
SigarVersion.SCM_REVISION;
/**
* The scm (svn) revision from which the sigar native binary was built.
*/
public static final String NATIVE_SCM_REVISION;
/**
* The date on which sigar.jar was built.
*/
public static final String BUILD_DATE =
SigarVersion.BUILD_DATE;
/**
* The date on which the sigar native binary was built.
*/
public static final String NATIVE_BUILD_DATE;
private static boolean enableLogging =
"true".equals(System.getProperty("sigar.nativeLogging"));
private static SigarLoader loader = new SigarLoader(Sigar.class);
private FileSystemMap mounts = null;
private boolean open = false;
int sigarWrapper = 0; //holds the sigar_t *
long longSigarWrapper = 0; //same, but where sizeof(void*) > sizeof(int)
// lastCpu is used to calculate the cpuPerc;
private Cpu lastCpu;
private Cpu[] lastCpuList;
private ProcessFinder processFinder = null;
static {
String nativeVersion = "unknown";
String nativeBuildDate = "unknown";
String nativeScmRevision = "unknown";
try {
loadLibrary();
nativeVersion = getNativeVersion();
nativeBuildDate = getNativeBuildDate();
nativeScmRevision = getNativeScmRevision();
checkVersion(nativeVersion);
} catch (SigarException e) {
loadError = e.getMessage();
try {
SigarLog.debug(loadError, e);
} catch (NoClassDefFoundError ne) {
//no log4j.jar
System.err.println(loadError);
e.printStackTrace();
}
}
NATIVE_VERSION_STRING = nativeVersion;
NATIVE_BUILD_DATE = nativeBuildDate;
NATIVE_SCM_REVISION = nativeScmRevision;
}
private static void checkVersion(String nativeVersionString)
throws SigarException {
StringTokenizer javaVersion =
new StringTokenizer(VERSION_STRING, ".");
StringTokenizer nativeVersion =
new StringTokenizer(nativeVersionString, ".");
String[] desc = { "major", "minor" };
for (int i=0; i
* Supported Platforms: Linux, Solaris and Windows.
* @param pid The process id.
* @return List of loaded modules.
* @exception SigarException on failure.
*/
private native List getProcModulesNative(long pid) throws SigarException;
public List getProcModules(long pid) throws SigarException {
return getProcModulesNative(pid);
}
public List getProcModules(String pid) throws SigarException {
return getProcModules(convertPid(pid));
}
/**
* Find the pid of the process which is listening on the given port.
* Supported Platforms: Linux, Windows 2003, Windows XP, AIX.
* @param protocol NetFlags.CONN_TCP or NetFlags.CONN_UDP.
* @param port The port number.
* @return pid of the process.
* @exception SigarException on failure.
*/
public native long getProcPort(int protocol, long port)
throws SigarException;
/**
* @param protocol "tcp" or "udp".
* @param port The port number.
* @return pid of the process.
* @exception SigarException on failure.
*/
public long getProcPort(String protocol, String port)
throws SigarException {
return getProcPort(NetFlags.getConnectionProtocol(protocol),
Integer.parseInt(port));
}
/**
* Get the cumulative cpu time for the calling thread.
*/
public ThreadCpu getThreadCpu() throws SigarException {
return ThreadCpu.fetch(this, 0);
}
private native FileSystem[] getFileSystemListNative() throws SigarException;
/**
* Get list of file systems.
* @exception SigarException on failure.
*/
public FileSystem[] getFileSystemList() throws SigarException {
FileSystem[] fslist = getFileSystemListNative();
if (this.mounts != null) {
this.mounts.init(fslist);
}
return fslist;
}
/**
* Get file system usage.
* @param name Name of the directory on which filesystem is mounted.
* @exception SigarException on failure.
*/
public FileSystemUsage getFileSystemUsage(String name)
throws SigarException {
if (name == null) {
throw new SigarException("name cannot be null");
}
return FileSystemUsage.fetch(this, name);
}
/**
* Get disk usage.
* @param name Name of disk
* @exception SigarException on failure.
*/
public DiskUsage getDiskUsage(String name)
throws SigarException {
if (name == null) {
throw new SigarException("name cannot be null");
}
return DiskUsage.fetch(this, name);
}
/**
* Get file system usage of a mounted directory.
* This method checks that the given directory is mounted.
* Unlike getFileSystemUsage() which only requires that the
* directory exists within a mounted file system.
* This method will also check that NFS servers are reachable via RPC
* before attempting to get the file system stats to prevent application
* hang when an NFS server is down.
* @param name Name of the directory on which filesystem is mounted.
* @exception SigarException If given directory is not mounted.
* @exception NfsUnreachableException If NFS server is unreachable.
* @see org.hyperic.sigar.Sigar#getFileSystemUsage
*/
public FileSystemUsage getMountedFileSystemUsage(String name)
throws SigarException, NfsUnreachableException {
FileSystem fs = getFileSystemMap().getFileSystem(name);
if (fs == null) {
throw new SigarException(name + " is not a mounted filesystem");
}
if (fs instanceof NfsFileSystem) {
NfsFileSystem nfs = (NfsFileSystem)fs;
if (!nfs.ping()) {
throw nfs.getUnreachableException();
}
}
return FileSystemUsage.fetch(this, name);
}
public FileSystemMap getFileSystemMap()
throws SigarException {
if (this.mounts == null) {
this.mounts = new FileSystemMap();
}
getFileSystemList(); //this.mounts.init()
return this.mounts;
}
public FileInfo getFileInfo(String name)
throws SigarException {
return FileInfo.fetchFileInfo(this, name);
}
public FileInfo getLinkInfo(String name)
throws SigarException {
return FileInfo.fetchLinkInfo(this, name);
}
public DirStat getDirStat(String name)
throws SigarException {
return DirStat.fetch(this, name);
}
public DirUsage getDirUsage(String name)
throws SigarException {
return DirUsage.fetch(this, name);
}
/**
* Get list of cpu infomation.
* @exception SigarException on failure.
*/
public native CpuInfo[] getCpuInfoList() throws SigarException;
private native Cpu[] getCpuListNative() throws SigarException;
/**
* Get list of per-cpu metrics.
* @exception SigarException on failure.
*/
public Cpu[] getCpuList() throws SigarException {
return getCpuListNative();
}
/**
* Get list of network routes.
* @exception SigarException on failure.
*/
public native NetRoute[] getNetRouteList() throws SigarException;
/**
* Get list of network connections.
* @exception SigarException on failure.
*/
public native NetConnection[] getNetConnectionList(int flags)
throws SigarException;
/**
* Get the TCP listen address for the given port.
* If there is not a listener on the given port, null will be returned.
*/
public native String getNetListenAddress(long port)
throws SigarException;
public String getNetListenAddress(String port)
throws SigarException {
return getNetListenAddress(Long.parseLong(port));
}
public native String getNetServicesName(int protocol, long port);
public NetStat getNetStat()
throws SigarException {
NetStat netstat = new NetStat();
netstat.stat(this);
return netstat;
}
public NetStat getNetStat(byte[] address, long port)
throws SigarException {
NetStat netstat = new NetStat();
netstat.stat(this, address, port);
return netstat;
}
public native Who[] getWhoList()
throws SigarException;
/**
* TCP-MIB stats
* @exception SigarException on failure.
*/
public Tcp getTcp()
throws SigarException {
return Tcp.fetch(this);
}
public NfsClientV2 getNfsClientV2()
throws SigarException {
return NfsClientV2.fetch(this);
}
public NfsServerV2 getNfsServerV2()
throws SigarException {
return NfsServerV2.fetch(this);
}
public NfsClientV3 getNfsClientV3()
throws SigarException {
return NfsClientV3.fetch(this);
}
public NfsServerV3 getNfsServerV3()
throws SigarException {
return NfsServerV3.fetch(this);
}
/**
* Get general network info.
* @exception SigarException on failure.
*/
public NetInfo getNetInfo()
throws SigarException {
return NetInfo.fetch(this);
}
/**
* Get network interface configuration info.
* @exception SigarException on failure.
*/
public NetInterfaceConfig getNetInterfaceConfig(String name)
throws SigarException {
return NetInterfaceConfig.fetch(this, name);
}
/**
* Get default network interface configuration info.
* Iterates getNetInterfaceList(), returning the first
* available ethernet interface.
* @exception SigarException on failure.
*/
public NetInterfaceConfig getNetInterfaceConfig()
throws SigarException {
String[] interfaces = getNetInterfaceList();
for (int i=0; i 0) {
continue;
}
if ((flags & NetFlags.IFF_LOOPBACK) > 0) {
continue;
}
return ifconfig;
}
String msg =
"No ethernet interface available";
throw new SigarException(msg);
}
/**
* Get network interface stats.
* @exception SigarException on failure.
*/
public NetInterfaceStat getNetInterfaceStat(String name)
throws SigarException {
return NetInterfaceStat.fetch(this, name);
}
/**
* Get the list of configured network interface names.
* @exception SigarException on failure.
*/
public native String[] getNetInterfaceList() throws SigarException;
/**
* Prompt for a password, disabling terminal echo
* during user input.
* @param prompt Text printed before disabling echo
* @return Text entered by the user.
* @throws IOException If input could not be read.
* @throws SigarNotImplementedException If the native method
* is not implemented on the current platform.
*/
native static String getPasswordNative(String prompt)
throws IOException, SigarNotImplementedException;
/**
* Prompt for a password, disabling terminal echo
* during user input if possible.
* @param prompt Text printed before disabling echo
* @return Text entered by the user.
* @throws IOException If input could not be read.
*/
public static String getPassword(String prompt)
throws IOException
{
try {
return getPasswordNative(prompt);
} catch (IOException e) {
throw e;
} catch (SigarNotImplementedException e) {
//fallthrough
}
//fallback if native .so was not loaded or not supported
System.out.print(prompt);
return (new BufferedReader(new InputStreamReader(System.in))).
readLine();
}
/**
* Reliably retrieve the FQDN for a machine
*
* @return The fully qualified domain name of the machine.
* @exception SigarException on failure.
*/
public native String getFQDN() throws SigarException;
/**
* Enabling logging in the native Sigar code.
* This method will hook log4j into the Sigar
* native logging methods. Note that the majority
* of logging in the native code is only at the DEBUG
* level.
*/
public void enableLogging(boolean value) {
if (value) {
SigarLog.enable(this);
}
else {
SigarLog.disable(this);
}
}
}