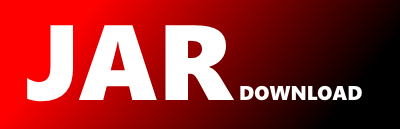
org.hyperic.sigar.SysInfo Maven / Gradle / Ivy
/*****************************************************
* WARNING: this file was generated by -e
* on Mon May 24 16:26:06 2010.
* Any changes made here will be LOST.
*****************************************************/
package org.hyperic.sigar;
import java.util.HashMap;
import java.util.Map;
/**
* SysInfo sigar class.
*/
public class SysInfo implements java.io.Serializable {
private static final long serialVersionUID = 16002L;
public SysInfo() { }
public native void gather(Sigar sigar) throws SigarException;
/**
* This method is not intended to be called directly.
* use Sigar.getSysInfo() instead.
* @exception SigarException on failure.
* @see org.hyperic.sigar.Sigar#getSysInfo
*/
static SysInfo fetch(Sigar sigar) throws SigarException {
SysInfo sysInfo = new SysInfo();
sysInfo.gather(sigar);
return sysInfo;
}
String name = null;
/**
* Get the name.
* Supported Platforms: All.
*
* @return name
*/
public String getName() { return name; }
String version = null;
/**
* Get the version.
* Supported Platforms: All.
*
* @return version
*/
public String getVersion() { return version; }
String arch = null;
/**
* Get the arch.
* Supported Platforms: All.
*
* @return arch
*/
public String getArch() { return arch; }
String machine = null;
/**
* Get the machine.
* Supported Platforms: All.
*
* @return machine
*/
public String getMachine() { return machine; }
String description = null;
/**
* Get the description.
* Supported Platforms: All.
*
* @return description
*/
public String getDescription() { return description; }
String patchLevel = null;
/**
* Get the patch_level.
* Supported Platforms: Win32.
*
* @return patch_level
*/
public String getPatchLevel() { return patchLevel; }
String vendor = null;
/**
* Get the vendor.
* Supported Platforms: All.
*
* @return vendor
*/
public String getVendor() { return vendor; }
String vendorVersion = null;
/**
* Get the vendor_version.
* Supported Platforms: All.
*
* @return vendor_version
*/
public String getVendorVersion() { return vendorVersion; }
String vendorName = null;
/**
* Get the vendor_name.
* Supported Platforms: All.
*
* @return vendor_name
*/
public String getVendorName() { return vendorName; }
String vendorCodeName = null;
/**
* Get the vendor_code_name.
* Supported Platforms: All.
*
* @return vendor_code_name
*/
public String getVendorCodeName() { return vendorCodeName; }
void copyTo(SysInfo copy) {
copy.name = this.name;
copy.version = this.version;
copy.arch = this.arch;
copy.machine = this.machine;
copy.description = this.description;
copy.patchLevel = this.patchLevel;
copy.vendor = this.vendor;
copy.vendorVersion = this.vendorVersion;
copy.vendorName = this.vendorName;
copy.vendorCodeName = this.vendorCodeName;
}
public Map toMap() {
Map map = new HashMap();
String strname =
String.valueOf(this.name);
if (!"-1".equals(strname))
map.put("Name", strname);
String strversion =
String.valueOf(this.version);
if (!"-1".equals(strversion))
map.put("Version", strversion);
String strarch =
String.valueOf(this.arch);
if (!"-1".equals(strarch))
map.put("Arch", strarch);
String strmachine =
String.valueOf(this.machine);
if (!"-1".equals(strmachine))
map.put("Machine", strmachine);
String strdescription =
String.valueOf(this.description);
if (!"-1".equals(strdescription))
map.put("Description", strdescription);
String strpatchLevel =
String.valueOf(this.patchLevel);
if (!"-1".equals(strpatchLevel))
map.put("PatchLevel", strpatchLevel);
String strvendor =
String.valueOf(this.vendor);
if (!"-1".equals(strvendor))
map.put("Vendor", strvendor);
String strvendorVersion =
String.valueOf(this.vendorVersion);
if (!"-1".equals(strvendorVersion))
map.put("VendorVersion", strvendorVersion);
String strvendorName =
String.valueOf(this.vendorName);
if (!"-1".equals(strvendorName))
map.put("VendorName", strvendorName);
String strvendorCodeName =
String.valueOf(this.vendorCodeName);
if (!"-1".equals(strvendorCodeName))
map.put("VendorCodeName", strvendorCodeName);
return map;
}
public String toString() {
return toMap().toString();
}
}