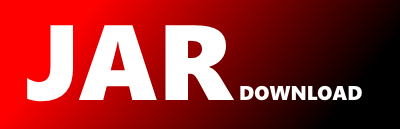
org.hyperic.sigar.Who Maven / Gradle / Ivy
/*****************************************************
* WARNING: this file was generated by -e
* on Mon May 24 16:26:06 2010.
* Any changes made here will be LOST.
*****************************************************/
package org.hyperic.sigar;
import java.util.HashMap;
import java.util.Map;
/**
* Who sigar class.
*/
public class Who implements java.io.Serializable {
private static final long serialVersionUID = 4241L;
public Who() { }
public native void gather(Sigar sigar) throws SigarException;
/**
* This method is not intended to be called directly.
* use Sigar.getWho() instead.
* @exception SigarException on failure.
* @see org.hyperic.sigar.Sigar#getWho
*/
static Who fetch(Sigar sigar) throws SigarException {
Who who = new Who();
who.gather(sigar);
return who;
}
String user = null;
/**
* Get the user.
* Supported Platforms: Undocumented.
*
* @return user
*/
public String getUser() { return user; }
String device = null;
/**
* Get the device.
* Supported Platforms: Undocumented.
*
* @return device
*/
public String getDevice() { return device; }
String host = null;
/**
* Get the host.
* Supported Platforms: Undocumented.
*
* @return host
*/
public String getHost() { return host; }
long time = 0;
/**
* Get the time.
* Supported Platforms: Undocumented.
*
* @return time
*/
public long getTime() { return time; }
void copyTo(Who copy) {
copy.user = this.user;
copy.device = this.device;
copy.host = this.host;
copy.time = this.time;
}
public Map toMap() {
Map map = new HashMap();
String struser =
String.valueOf(this.user);
if (!"-1".equals(struser))
map.put("User", struser);
String strdevice =
String.valueOf(this.device);
if (!"-1".equals(strdevice))
map.put("Device", strdevice);
String strhost =
String.valueOf(this.host);
if (!"-1".equals(strhost))
map.put("Host", strhost);
String strtime =
String.valueOf(this.time);
if (!"-1".equals(strtime))
map.put("Time", strtime);
return map;
}
public String toString() {
return toMap().toString();
}
}