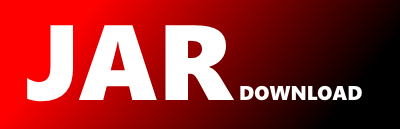
org.hyperic.sigar.win32.Service Maven / Gradle / Ivy
/*
* Copyright (c) 2006-2008 Hyperic, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.hyperic.sigar.win32;
import java.io.PrintStream;
import java.util.Arrays;
import java.util.ArrayList;
import java.util.List;
import org.hyperic.sigar.Sigar;
public class Service extends Win32 {
// Service State
public static final int SERVICE_STOPPED = 0x00000001;
public static final int SERVICE_START_PENDING = 0x00000002;
public static final int SERVICE_STOP_PENDING = 0x00000003;
public static final int SERVICE_RUNNING = 0x00000004;
public static final int SERVICE_CONTINUE_PENDING = 0x00000005;
public static final int SERVICE_PAUSE_PENDING = 0x00000006;
public static final int SERVICE_PAUSED = 0x00000007;
private static final String[] STATUS = {
"Unknown",
"Stopped",
"Start Pending",
"Stop Pending",
"Running",
"Continue Pending",
"Pause Pending",
"Paused"
};
// Service Controls
private static final int CONTROL_START = 0x00000000;
private static final int CONTROL_STOP = 0x00000001;
private static final int CONTROL_PAUSE = 0x00000002;
private static final int CONTROL_RESUME = 0x00000003;
// Service Control Manager object specific access types
private static final int STANDARD_RIGHTS_REQUIRED = (int)0x000F0000L;
private static final int SC_MANAGER_CONNECT = 0x0001;
private static final int SC_MANAGER_CREATE_SERVICE = 0x0002;
private static final int SC_MANAGER_ENUMERATE_SERVICE = 0x0004;
private static final int SC_MANAGER_LOCK = 0x0008;
private static final int SC_MANAGER_QUERY_LOCK_STATUS = 0x0010;
private static final int SC_MANAGER_MODIFY_BOOT_CONFIG = 0x0020;
private static final int SC_MANAGER_ALL_ACCESS =
(STANDARD_RIGHTS_REQUIRED |
SC_MANAGER_CONNECT |
SC_MANAGER_CREATE_SERVICE |
SC_MANAGER_ENUMERATE_SERVICE |
SC_MANAGER_LOCK |
SC_MANAGER_QUERY_LOCK_STATUS |
SC_MANAGER_MODIFY_BOOT_CONFIG);
// Service object specific access type
private static final int SERVICE_QUERY_CONFIG = 0x0001;
private static final int SERVICE_CHANGE_CONFIG = 0x0002;
private static final int SERVICE_QUERY_STATUS = 0x0004;
private static final int SERVICE_ENUMERATE_DEPENDENTS = 0x0008;
private static final int SERVICE_START = 0x0010;
private static final int SERVICE_STOP = 0x0020;
private static final int SERVICE_PAUSE_CONTINUE = 0x0040;
private static final int SERVICE_INTERROGATE = 0x0080;
private static final int SERVICE_USER_DEFINED_CONTROL = 0x0100;
private static final int SERVICE_ALL_ACCESS =
(STANDARD_RIGHTS_REQUIRED |
SERVICE_QUERY_CONFIG |
SERVICE_CHANGE_CONFIG |
SERVICE_QUERY_STATUS |
SERVICE_ENUMERATE_DEPENDENTS |
SERVICE_START |
SERVICE_STOP |
SERVICE_PAUSE_CONTINUE |
SERVICE_INTERROGATE |
SERVICE_USER_DEFINED_CONTROL);
private long manager;
private long service;
private String name;
private Service() throws Win32Exception
{
this.manager = OpenSCManager("", SC_MANAGER_ALL_ACCESS);
}
public static native List getServiceNames(Sigar sigar, String ptql)
throws Win32Exception;
public static List getServiceNames() throws Win32Exception {
return getServiceNames(null, null);
}
public static List getServiceConfigs(Sigar sigar, String exe)
throws Win32Exception {
List services = new ArrayList();
List names = Service.getServiceNames(sigar,
"Service.Exe.Ieq=" + exe);
for (int i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy