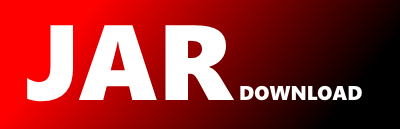
io.katharsis.jpa.internal.query.AbstractQueryExecutorImpl Maven / Gradle / Ivy
package io.katharsis.jpa.internal.query;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import javax.persistence.EntityGraph;
import javax.persistence.EntityManager;
import javax.persistence.Query;
import javax.persistence.Subgraph;
import io.katharsis.jpa.internal.meta.MetaAttributePath;
import io.katharsis.jpa.internal.meta.MetaDataObject;
import io.katharsis.jpa.query.JpaQueryExecutor;
public abstract class AbstractQueryExecutorImpl implements JpaQueryExecutor {
protected int offset = 0;
protected int limit = -1;
protected boolean cached = false;
protected EntityManager em;
protected int numAutoSelections;
protected Set fetchPaths = new HashSet<>();
protected MetaDataObject meta;
protected Map selectionBindings;
public AbstractQueryExecutorImpl(EntityManager em, MetaDataObject meta, int numAutoSelections, Map selectionBindings) {
this.em = em;
this.meta = meta;
this.numAutoSelections = numAutoSelections;
this.selectionBindings = selectionBindings;
}
@Override
public JpaQueryExecutor fetch(List attrPath) {
// include path an all prefix paths
MetaAttributePath path = meta.resolvePath(attrPath);
for (int i = 1; i <= path.length(); i++) {
fetchPaths.add(path.subPath(0, i));
}
return this;
}
@Override
public JpaQueryExecutor setCached(boolean cached) {
this.cached = cached;
return this;
}
@Override
public JpaQueryExecutor setOffset(int offset) {
this.offset = offset;
return this;
}
@Override
public JpaQueryExecutor setLimit(int limit) {
this.limit = limit;
return this;
}
@Override
public JpaQueryExecutor setWindow(int offset, int limit) {
this.offset = offset;
this.limit = limit;
return this;
}
@SuppressWarnings("unchecked")
@Override
public List getResultList() {
List> list = executeQuery();
// due to sorting & distinct we have a multiselect even
// if we are only interested in the entites.
List resultList;
if (isCompoundSelection()) {
List entityList = new ArrayList<>();
for (Object obj : list) {
Object[] values = (Object[]) obj;
entityList.add((T) values[0]);
}
resultList = entityList;
} else {
resultList = (List) list;
}
return resultList;
}
protected abstract boolean isCompoundSelection();
protected abstract List> executeQuery(); // NOSONAR
@Override
public T getUniqueResult(boolean nullable) {
List list = getResultList();
if (list.size() > 1)
throw new IllegalStateException("query does not return unique value, " + list.size() + " results returned");
if (!list.isEmpty())
return list.get(0);
else if (nullable)
return null;
else
throw new IllegalStateException("no result found");
}
protected List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy