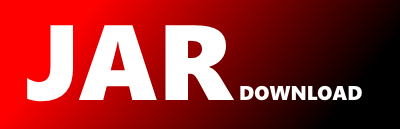
io.katharsis.jpa.internal.query.backend.criteria.CriteriaTupleImpl Maven / Gradle / Ivy
package io.katharsis.jpa.internal.query.backend.criteria;
import java.util.Arrays;
import java.util.List;
import java.util.Map;
import javax.persistence.Tuple;
import javax.persistence.TupleElement;
public class CriteriaTupleImpl implements Tuple, io.katharsis.jpa.query.Tuple {
private Object[] data;
private Map selectionBindings;
private int numEntriesToIgnore = 0;
protected CriteriaTupleImpl(Object[] data, Map selectionBindings) {
this.data = data;
this.selectionBindings = selectionBindings;
}
@Override
public X get(TupleElement tupleElement) {
throw new UnsupportedOperationException("not implemented");
}
@SuppressWarnings("unchecked")
@Override
public X get(String alias, Class type) {
return (X) get(alias);
}
@Override
public Object get(String alias) {
Integer index = selectionBindings.get(alias);
if (index == null) {
throw new IllegalArgumentException("selection " + alias + " not found");
}
return get(index.intValue());
}
@SuppressWarnings("unchecked")
@Override
public X get(int i, Class type) {
return (X) data[i + numEntriesToIgnore];
}
@Override
public Object get(int i) {
return data[i + numEntriesToIgnore];
}
@Override
public Object[] toArray() {
if (numEntriesToIgnore > 0) {
return Arrays.copyOfRange(data, numEntriesToIgnore, data.length - numEntriesToIgnore);
}
else {
return data;
}
}
@Override
public List> getElements() {
throw new UnsupportedOperationException("not implemented");
}
@Override
public void reduce(int numEntriesToIgnore) {
this.numEntriesToIgnore = numEntriesToIgnore;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy