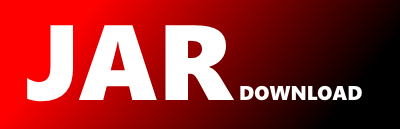
io.keen.client.java.Query Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of keen-client-api-query Show documentation
Show all versions of keen-client-api-query Show documentation
Java Query Client API for Keen IO
package io.keen.client.java;
import java.net.URL;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import io.keen.client.java.exceptions.KeenQueryClientException;
/**
* Query represents all the details of the query to be run, including required
* and optional parameters.
*
* Created by claireyoung on 5/18/15.
* @author claireyoung, baumatron, masojus
* @since 1.0.0
*/
public class Query extends KeenQueryRequest {
// required
private final QueryType queryType;
private final String eventCollection;
private final Timeframe timeframe;
// sometimes optional
private final String targetProperty;
// optional
private final RequestParameterCollection filters;
private final String interval; // requires timeframe to be set
private final String timezone;
private final List groupBy;
private final Integer maxAge; // integer greater than 30 seconds: https://keen.io/docs/data-analysis/caching/
// required by the Percentile query
private final Double percentile; // 0-100, two decimal places of precision, e.g. 99.99
/**
* Constructs the map to pass to the JSON handler, so that the proper required
* and optional Query arguments can be sent out to the server.
*
* @return The JSON object map.
*/
@Override
Map constructRequestArgs() {
Map queryArgs = new HashMap();
if (eventCollection != null) {
queryArgs.put(KeenQueryConstants.EVENT_COLLECTION, eventCollection);
}
if (interval != null) {
queryArgs.put(KeenQueryConstants.INTERVAL, interval);
}
// This will be overwritten by the timeframe if provided. Timezone is only applicable for
// a relative timeframe, and so this property will go away in the future.
if (timezone != null) {
queryArgs.put(KeenQueryConstants.TIMEZONE, timezone);
}
if (groupBy != null) {
queryArgs.put(KeenQueryConstants.GROUP_BY, groupBy);
}
if (maxAge != null) {
queryArgs.put(KeenQueryConstants.MAX_AGE, maxAge);
}
if (targetProperty != null) {
queryArgs.put(KeenQueryConstants.TARGET_PROPERTY, targetProperty);
}
if (percentile != null) {
queryArgs.put(KeenQueryConstants.PERCENTILE, percentile);
}
if (filters != null) {
queryArgs.put(KeenQueryConstants.FILTERS, filters.constructParameterRequestArgs());
}
if (timeframe != null) {
queryArgs.putAll(timeframe.constructTimeframeArgs());
}
return queryArgs;
}
/**
* @return the query type
*/
public QueryType getQueryType() { return queryType; }
/**
* @return whether this query has a GroupBy specified.
*/
public boolean hasGroupBy() { return groupBy != null; }
/**
* @return whether this query has an Interval specified.
*/
public boolean hasInterval() { return interval != null; }
/**
* Verifies whether the parameters are valid, based on the input query name.
*
* @return whether the parameters are valid.
*/
public boolean areParamsValid() {
if (queryType == QueryType.COUNT) {
if (eventCollection == null || eventCollection.isEmpty()) {
return false;
}
}
if (queryType == QueryType.COUNT_UNIQUE
|| queryType == QueryType.MINIMUM || queryType == QueryType.MAXIMUM
|| queryType == QueryType.AVERAGE || queryType == QueryType.MEDIAN
|| queryType == QueryType.PERCENTILE || queryType == QueryType.SUM
|| queryType == QueryType.SELECT_UNIQUE) {
if (eventCollection == null || eventCollection.isEmpty() || targetProperty == null || targetProperty.isEmpty()) {
return false;
}
}
if (queryType == QueryType.PERCENTILE) {
if (percentile == null) {
return false;
}
}
return true;
}
/**
* Constructs a Keen Query using a Builder.
*
* @param builder The builder from which to retrieve this client's interfaces and settings.
*/
protected Query(Builder builder) {
this.eventCollection = builder.eventCollection;
this.targetProperty = builder.targetProperty;
this.interval = builder.interval;
this.timezone = builder.timezone;
this.groupBy = builder.groupBy;
this.maxAge = builder.maxAge;
this.percentile = builder.percentile;
this.queryType = builder.queryType;
this.timeframe = builder.timeframe;
if (null != builder.filters && !builder.filters.isEmpty()) {
this.filters = new RequestParameterCollection(builder.filters);
} else {
this.filters = null;
}
}
@Override
URL getRequestURL(RequestUrlBuilder urlBuilder,
String projectId) throws KeenQueryClientException {
return urlBuilder.getAnalysisUrl(projectId, getAnalysisType());
}
@Override
String getAnalysisType() {
return queryType.toString();
}
@Override
boolean groupedResponseExpected() {
return this.hasGroupBy();
}
@Override
boolean intervalResponseExpected() {
return this.hasInterval();
}
@Override
Collection getGroupByParams() {
return this.groupBy;
}
/**
* Builder to construct a query with required and optional arguments.
* Note that the only required argument in this builder is QueryType, although
* individual queries may require additional arguments.
*/
public static class Builder {
// required
private QueryType queryType;
private String eventCollection;
private Timeframe timeframe;
// sometimes optional
private String targetProperty;
// required by the Percentile query
private Double percentile; // 0-100, two decimal places of precision, e.g. 99.99
// optional
private Collection filters;
private String interval;
private String timezone;
private List groupBy;
private Integer maxAge;
public Builder(QueryType queryType) {
this.queryType = queryType;
}
/**
* Get filters
*
* @return a collection of filters.
*/
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy