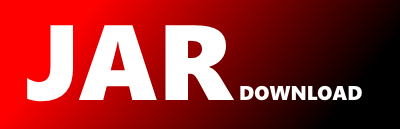
io.keen.client.java.RequestParameterCollection Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of keen-client-api-query Show documentation
Show all versions of keen-client-api-query Show documentation
Java Query Client API for Keen IO
package io.keen.client.java;
import java.util.Collection;
import java.util.Collections;
import java.util.Iterator;
import java.util.LinkedList;
/**
* A helper class for dealing with collections of jsonifiable parameters.
* Constructs a jsonifiable collection of the jsonifiable parameters for each sub-parameter.
*
* @author baumatron, masojus
* @param The type of RequestParameter
*/
class RequestParameterCollection>
extends RequestParameter>
implements Iterable {
private final Collection extends RequestParameterT> parameters;
RequestParameterCollection(Collection extends RequestParameterT> parameters) {
if (null == parameters || parameters.isEmpty()) {
throw new IllegalArgumentException("'parameters' is a required parameter and must not be empty.");
}
this.parameters = parameters;
}
@Override
Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy