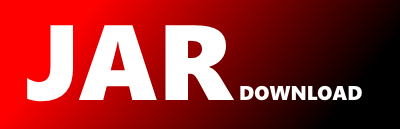
io.kestra.plugin.jdbc.JdbcConnectionInterface Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of plugin-jdbc Show documentation
Show all versions of plugin-jdbc Show documentation
Abstract JDBC plugin for Kestra
package io.kestra.plugin.jdbc;
import io.kestra.core.models.annotations.PluginProperty;
import io.kestra.core.runners.RunContext;
import io.swagger.v3.oas.annotations.media.Schema;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
import java.util.Properties;
import javax.validation.constraints.NotNull;
public interface JdbcConnectionInterface {
@Schema(
title = "The jdbc url to connect to the database"
)
@PluginProperty(dynamic = true)
@NotNull
String getUrl();
@Schema(
title = "The database user"
)
@PluginProperty(dynamic = true)
String getUsername();
@Schema(
title = "The database user's password"
)
@PluginProperty(dynamic = true)
String getPassword();
/**
* JDBC driver may be auto-registered. See DriverManager
*
* @throws SQLException registerDrivers failed
*/
void registerDriver() throws SQLException;
default Properties connectionProperties(RunContext runContext) throws Exception {
Properties props = new Properties();
props.put("jdbc.url", runContext.render(this.getUrl()));
if (this.getUsername() != null) {
props.put("user", runContext.render(this.getUsername()));
}
if (this.getPassword() != null) {
props.put("password", runContext.render(this.getPassword()));
}
return props;
}
default Connection connection(RunContext runContext) throws Exception {
registerDriver();
Properties props = this.connectionProperties(runContext);
String jdbcUrl = props.getProperty("jdbc.url");
props.remove("jdbc.url");
return DriverManager.getConnection(jdbcUrl, props);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy