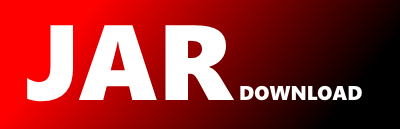
io.kestra.plugin.kubernetes.services.InstanceService Maven / Gradle / Ivy
package io.kestra.plugin.kubernetes.services;
import com.fasterxml.jackson.databind.ObjectMapper;
import io.kestra.core.exceptions.IllegalVariableEvaluationException;
import io.kestra.core.runners.RunContext;
import io.kestra.core.serializers.JacksonMapper;
import io.kestra.core.utils.MapUtils;
import java.io.IOException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
abstract public class InstanceService {
private static final ObjectMapper mapper = JacksonMapper.ofYaml();
@SuppressWarnings({"unchecked", "rawtypes"})
public static T fromMap(Class cls, RunContext runContext, Map map) throws IOException, IllegalVariableEvaluationException {
Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy