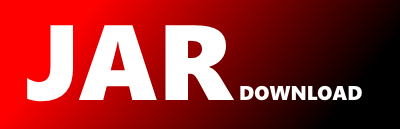
io.kestra.plugin.mqtt.Publish Maven / Gradle / Ivy
package io.kestra.plugin.mqtt;
import com.fasterxml.jackson.core.JsonProcessingException;
import io.kestra.core.models.annotations.Example;
import io.kestra.core.models.annotations.Plugin;
import io.kestra.core.models.annotations.PluginProperty;
import io.kestra.core.models.executions.metrics.Counter;
import io.kestra.core.models.tasks.RunnableTask;
import io.kestra.core.runners.RunContext;
import io.kestra.core.serializers.FileSerde;
import io.kestra.core.serializers.JacksonMapper;
import io.kestra.plugin.mqtt.services.MqttFactory;
import io.kestra.plugin.mqtt.services.MqttInterface;
import io.kestra.plugin.mqtt.services.SerdeType;
import io.swagger.v3.oas.annotations.media.Schema;
import lombok.*;
import lombok.experimental.SuperBuilder;
import jakarta.validation.constraints.NotNull;
import reactor.core.publisher.Flux;
import reactor.core.publisher.FluxSink;
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.URI;
import java.nio.charset.StandardCharsets;
import java.util.List;
import java.util.Map;
import static io.kestra.core.utils.Rethrow.throwFunction;
@SuperBuilder
@ToString
@EqualsAndHashCode
@Getter
@NoArgsConstructor
@Schema(
title = "Produce message in a MQTT topic"
)
@Plugin(
examples = {
@Example(
code = {
"server: tcp://localhost:1883",
"clientId: kestraProducer",
"topic: kestra/sensors/cpu",
"serdeType: JSON",
"retain: true",
"from: ",
" type: \"sensors\"",
" value: 1.23",
}
),
@Example(
code = {
"server: ssl://localhost:8883",
"clientId: kestraProducer",
"topic: kestra/sensors/cpu",
"crt: /home/path/to/ca.crt",
"serdeType: JSON",
"retain: true",
"from: ",
" type: \"sensors\"",
" value: 1.23",
}
)
}
)
public class Publish extends AbstractMqttConnection implements RunnableTask, MqttPropertiesInterface {
@Schema(
title = "Topic where to send message"
)
@NotNull
@PluginProperty(dynamic = true)
private String topic;
@Schema(
title = "Source of message send",
description = "Can be an internal storage uri, a map or a list.",
anyOf = {String.class, Object[].class, Map.class }
)
@NotNull
@PluginProperty(dynamic = true)
private Object from;
@Schema(
title = "Whether or not the publish message should be retained by the messaging engine. ",
description = "Sending a message with retained set to true and with an empty byte array as the " +
"payload e.g. `null` will clear the retained message from the server."
)
@NotNull
@PluginProperty
@Builder.Default
private Boolean retain = false;
private SerdeType serdeType;
@Builder.Default
private Integer qos = 1;
@SuppressWarnings("unchecked")
@Override
public Publish.Output run(RunContext runContext) throws Exception {
MqttInterface connection = MqttFactory.create(runContext, this);
Integer count = 1;
String topic = runContext.render(this.topic);
if (this.from instanceof String || this.from instanceof List) {
Flux
© 2015 - 2024 Weber Informatics LLC | Privacy Policy