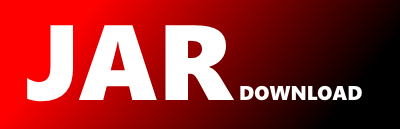
io.konik.zugferd.entity.CommonDelivery Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of konik Show documentation
Show all versions of konik Show documentation
Konik the ZUGFeRD processing library
The newest version!
/* Copyright (C) 2014 konik.io
*
* This file is part of the Konik library.
*
* The Konik library is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* The Konik library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with the Konik library. If not, see .
*/
package io.konik.zugferd.entity;
import java.io.Serializable;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlTransient;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import io.konik.jaxb.bindable.entity.SupplyChainEventAdapter;
import io.konik.validator.annotation.Basic;
import io.konik.zugferd.entity.trade.item.ReferencedDocumentItem;
import io.konik.zugferd.unqualified.ZfDate;
/**
* = The Common Delivery class.
*
* @param The {@link ReferencedDocument} or the {@link ReferencedDocumentItem} that each Delivery might contain.
*/
@XmlTransient
@XmlType(name = "SupplyChainTradeDeliveryType")
public abstract class CommonDelivery implements Serializable {
@XmlElement(name = "ShipToTradeParty")
private TradeParty shipTo;
@XmlElement(name = "UltimateShipToTradeParty")
private TradeParty ultimateShipTo;
@XmlElement(name = "ActualDeliverySupplyChainEvent")
@XmlJavaTypeAdapter(value = SupplyChainEventAdapter.class)
private ZfDate actualDelivery;
protected CommonDelivery() {
super();
}
/**
* Gets the ship to.
*
* @return the ship to
*/
public TradeParty getShipTo() {
return shipTo;
}
/**
* Sets the ship to.
*
* @param shipTo the ship to
* @return the delivery
*/
public CommonDelivery setShipTo(TradeParty shipTo) {
this.shipTo = shipTo;
return this;
}
/**
* Gets the ultimate ship to.
*
* @return the ultimate ship to
*/
public TradeParty getUltimateShipTo() {
return ultimateShipTo;
}
/**
* Sets the ultimate ship to.
*
* @param ultimateShipTo the ultimate ship to
* @return the delivery
*/
public CommonDelivery setUltimateShipTo(TradeParty ultimateShipTo) {
this.ultimateShipTo = ultimateShipTo;
return this;
}
/**
* Gets the actual delivery event.
*
* Delivery and performance date in sales tax purposes
*
* @return the actual event
*/
@Basic
public ZfDate getActualDelivery() {
return actualDelivery;
}
/**
* Sets the actual delivery event.
*
* Delivery and performance date in sales tax purposes
*
* @param actualDelivery the actual delivery
* @return the delivery
*/
public CommonDelivery setActualDelivery(ZfDate actualDelivery) {
this.actualDelivery = actualDelivery;
return this;
}
/**
* Gets the despatch advice.
*
* @return the despatch advice
*/
public abstract R getDespatchAdvice();
/**
* Sets the despatch advice.
*
* @param despatchAdvice the despatch advice
* @return the common delivery
*/
public abstract CommonDelivery setDespatchAdvice(R despatchAdvice);
/**
* Gets the delivery note.
*
* @return the note
*/
public abstract R getDeliveryNote();
/**
* Sets the delivery note.
*
* @param deliveryNote the delivery note
* @return the delivery
*/
public abstract CommonDelivery setDeliveryNote(R deliveryNote);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy