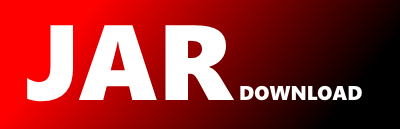
commonMain.io.ktor.server.html.Template.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ktor-server-html-builder-jvm Show documentation
Show all versions of ktor-server-html-builder-jvm Show documentation
Ktor is a framework for quickly creating web applications in Kotlin with minimal effort.
The newest version!
/*
* Copyright 2014-2021 JetBrains s.r.o and contributors. Use of this source code is governed by the Apache 2.0 license.
*/
package io.ktor.server.html
/**
* A template that expands inside [TOuter].
* @see [respondHtmlTemplate]
*/
public interface Template {
public fun TOuter.apply()
}
/**
* A placeholder that is inserted inside [TOuter].
* @see [respondHtmlTemplate]
*/
public open class Placeholder {
private var content: TOuter.(Placeholder) -> Unit = { }
public var meta: String = ""
public operator fun invoke(meta: String = "", content: TOuter.(Placeholder) -> Unit) {
this.content = content
this.meta = meta
}
public fun apply(destination: TOuter) {
destination.content(this)
}
}
/**
* A placeholder that can be used to insert the content that appears multiple times (for example, list items).
* @see [respondHtmlTemplate]
*/
public open class PlaceholderList {
private var items = ArrayList>()
public val size: Int
get() = items.size
public operator fun invoke(meta: String = "", content: TInner.(Placeholder) -> Unit = {}) {
val placeholder = PlaceholderItem(items.size, items)
placeholder(meta, content)
items.add(placeholder)
}
public fun isEmpty(): Boolean = items.size == 0
public fun isNotEmpty(): Boolean = isEmpty().not()
public fun apply(destination: TOuter, render: TOuter.(PlaceholderItem) -> Unit) {
for (item in items) {
destination.render(item)
}
}
}
/**
* An item of a [PlaceholderList] when it is expanded.
*/
public class PlaceholderItem(public val index: Int, public val collection: List>) :
Placeholder() {
public val first: Boolean get() = index == 0
public val last: Boolean get() = index == collection.lastIndex
}
/**
* Inserts every element of [PlaceholderList].
*/
public fun TOuter.each(
items: PlaceholderList,
itemTemplate: TOuter.(PlaceholderItem) -> Unit
) {
items.apply(this, itemTemplate)
}
/**
* Inserts [Placeholder].
*/
public fun TOuter.insert(placeholder: Placeholder): Unit = placeholder.apply(this)
/**
* A placeholder that is also a [Template].
* It can be used to insert child templates and create nested layouts.
*/
public open class TemplatePlaceholder {
private var content: TTemplate.() -> Unit = { }
public operator fun invoke(content: TTemplate.() -> Unit) {
this.content = content
}
public fun apply(template: TTemplate) {
template.content()
}
}
public fun , TOuter> TOuter.insert(
template: TTemplate,
placeholder: TemplatePlaceholder
) {
placeholder.apply(template)
with(template) { apply() }
}
public fun > TOuter.insert(template: TTemplate, build: TTemplate.() -> Unit) {
template.build()
with(template) { apply() }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy