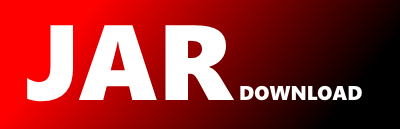
commonMain.io.ktor.util.Attributes.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ktor-utils-watchosarm64 Show documentation
Show all versions of ktor-utils-watchosarm64 Show documentation
Ktor is a framework for quickly creating web applications in Kotlin with minimal effort.
The newest version!
/*
* Copyright 2014-2024 JetBrains s.r.o and contributors. Use of this source code is governed by the Apache 2.0 license.
*/
package io.ktor.util
import io.ktor.util.reflect.*
import kotlin.jvm.*
import kotlin.reflect.*
/**
* Specifies a key for an attribute in [Attributes]
* @param T is a type of the value stored in the attribute
* @param name is a name of the attribute for diagnostic purposes. Can't be blank
*/
@JvmSynthetic
public inline fun AttributeKey(name: String): AttributeKey =
AttributeKey(name, typeInfo())
/**
* Specifies a key for an attribute in [Attributes]
* @param T is a type of the value stored in the attribute
* @property name is a name of the attribute for diagnostic purposes. Can't be blank
* @property type the recorded kotlin type of T
*/
public data class AttributeKey @JvmOverloads constructor(
public val name: String,
private val type: TypeInfo = typeInfo(),
) {
init {
require(name.isNotBlank()) { "Name can't be blank" }
}
override fun toString(): String = "AttributeKey: $name"
}
/**
* A version of [AttributeKey] that overrides [equals] and [hashCode] using [name]
* @param T is a type of the value stored in the attribute
* @param name is a name of the attribute
*/
@Deprecated(
"Please use `AttributeKey` class instead",
replaceWith = ReplaceWith("AttributeKey"),
level = DeprecationLevel.ERROR
)
public typealias EquatableAttributeKey = AttributeKey
/**
* Creates an attributes instance suitable for the particular platform
*/
public expect fun Attributes(concurrent: Boolean = false): Attributes
/**
* Map of attributes accessible by [AttributeKey] in a typed manner
*/
public interface Attributes {
/**
* Gets a value of the attribute for the specified [key], or throws an exception if an attribute doesn't exist
*/
public operator fun get(key: AttributeKey): T =
getOrNull(key) ?: throw IllegalStateException("No instance for key $key")
/**
* Gets a value of the attribute for the specified [key], or return `null` if an attribute doesn't exist
*/
public fun getOrNull(key: AttributeKey): T?
/**
* Checks if an attribute with the specified [key] exists
*/
public operator fun contains(key: AttributeKey<*>): Boolean
/**
* Creates or changes an attribute with the specified [key] using [value]
*/
public fun put(key: AttributeKey, value: T)
/**
* Removes an attribute with the specified [key]
*/
public fun remove(key: AttributeKey)
/**
* Removes an attribute with the specified [key] and returns its current value, throws an exception if an attribute doesn't exist
*/
public fun take(key: AttributeKey): T = get(key).also { remove(key) }
/**
* Removes an attribute with the specified [key] and returns its current value, returns `null` if an attribute doesn't exist
*/
public fun takeOrNull(key: AttributeKey): T? = getOrNull(key).also { remove(key) }
/**
* Gets a value of the attribute for the specified [key], or calls supplied [block] to compute its value
*/
public fun computeIfAbsent(key: AttributeKey, block: () -> T): T
/**
* Returns [List] of all [AttributeKey] instances in this map
*/
public val allKeys: List>
}
/**
* Adds all attributes from another collection, replacing original values if any.
*/
public fun Attributes.putAll(other: Attributes) {
other.allKeys.forEach {
@Suppress("UNCHECKED_CAST")
put(it as AttributeKey, other[it])
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy