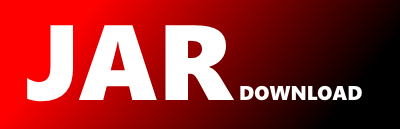
io.kubernetes.client.openapi.models.V1beta1PodSecurityPolicySpecFluent Maven / Gradle / Ivy
/*
Copyright 2022 The Kubernetes Authors.
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package io.kubernetes.client.openapi.models;
import io.kubernetes.client.fluent.Fluent;
import io.kubernetes.client.fluent.Nested;
import java.util.Collection;
import java.util.List;
import java.util.function.Predicate;
/** Generated */
public interface V1beta1PodSecurityPolicySpecFluent>
extends Fluent {
public Boolean getAllowPrivilegeEscalation();
public A withAllowPrivilegeEscalation(java.lang.Boolean allowPrivilegeEscalation);
public java.lang.Boolean hasAllowPrivilegeEscalation();
public A addToAllowedCSIDrivers(Integer index, V1beta1AllowedCSIDriver item);
public A setToAllowedCSIDrivers(
java.lang.Integer index, io.kubernetes.client.openapi.models.V1beta1AllowedCSIDriver item);
public A addToAllowedCSIDrivers(
io.kubernetes.client.openapi.models.V1beta1AllowedCSIDriver... items);
public A addAllToAllowedCSIDrivers(
Collection items);
public A removeFromAllowedCSIDrivers(
io.kubernetes.client.openapi.models.V1beta1AllowedCSIDriver... items);
public A removeAllFromAllowedCSIDrivers(
java.util.Collection items);
public A removeMatchingFromAllowedCSIDrivers(Predicate predicate);
/**
* This method has been deprecated, please use method buildAllowedCSIDrivers instead.
*
* @return The buildable object.
*/
@Deprecated
public List getAllowedCSIDrivers();
public java.util.List
buildAllowedCSIDrivers();
public io.kubernetes.client.openapi.models.V1beta1AllowedCSIDriver buildAllowedCSIDriver(
java.lang.Integer index);
public io.kubernetes.client.openapi.models.V1beta1AllowedCSIDriver buildFirstAllowedCSIDriver();
public io.kubernetes.client.openapi.models.V1beta1AllowedCSIDriver buildLastAllowedCSIDriver();
public io.kubernetes.client.openapi.models.V1beta1AllowedCSIDriver buildMatchingAllowedCSIDriver(
java.util.function.Predicate<
io.kubernetes.client.openapi.models.V1beta1AllowedCSIDriverBuilder>
predicate);
public java.lang.Boolean hasMatchingAllowedCSIDriver(
java.util.function.Predicate<
io.kubernetes.client.openapi.models.V1beta1AllowedCSIDriverBuilder>
predicate);
public A withAllowedCSIDrivers(
java.util.List
allowedCSIDrivers);
public A withAllowedCSIDrivers(
io.kubernetes.client.openapi.models.V1beta1AllowedCSIDriver... allowedCSIDrivers);
public java.lang.Boolean hasAllowedCSIDrivers();
public V1beta1PodSecurityPolicySpecFluent.AllowedCSIDriversNested addNewAllowedCSIDriver();
public io.kubernetes.client.openapi.models.V1beta1PodSecurityPolicySpecFluent
.AllowedCSIDriversNested<
A>
addNewAllowedCSIDriverLike(io.kubernetes.client.openapi.models.V1beta1AllowedCSIDriver item);
public io.kubernetes.client.openapi.models.V1beta1PodSecurityPolicySpecFluent
.AllowedCSIDriversNested<
A>
setNewAllowedCSIDriverLike(
java.lang.Integer index,
io.kubernetes.client.openapi.models.V1beta1AllowedCSIDriver item);
public io.kubernetes.client.openapi.models.V1beta1PodSecurityPolicySpecFluent
.AllowedCSIDriversNested<
A>
editAllowedCSIDriver(java.lang.Integer index);
public io.kubernetes.client.openapi.models.V1beta1PodSecurityPolicySpecFluent
.AllowedCSIDriversNested<
A>
editFirstAllowedCSIDriver();
public io.kubernetes.client.openapi.models.V1beta1PodSecurityPolicySpecFluent
.AllowedCSIDriversNested<
A>
editLastAllowedCSIDriver();
public io.kubernetes.client.openapi.models.V1beta1PodSecurityPolicySpecFluent
.AllowedCSIDriversNested<
A>
editMatchingAllowedCSIDriver(
java.util.function.Predicate<
io.kubernetes.client.openapi.models.V1beta1AllowedCSIDriverBuilder>
predicate);
public A addToAllowedCapabilities(java.lang.Integer index, String item);
public A setToAllowedCapabilities(java.lang.Integer index, java.lang.String item);
public A addToAllowedCapabilities(java.lang.String... items);
public A addAllToAllowedCapabilities(java.util.Collection items);
public A removeFromAllowedCapabilities(java.lang.String... items);
public A removeAllFromAllowedCapabilities(java.util.Collection items);
public java.util.List getAllowedCapabilities();
public java.lang.String getAllowedCapability(java.lang.Integer index);
public java.lang.String getFirstAllowedCapability();
public java.lang.String getLastAllowedCapability();
public java.lang.String getMatchingAllowedCapability(
java.util.function.Predicate predicate);
public java.lang.Boolean hasMatchingAllowedCapability(
java.util.function.Predicate predicate);
public A withAllowedCapabilities(java.util.List allowedCapabilities);
public A withAllowedCapabilities(java.lang.String... allowedCapabilities);
public java.lang.Boolean hasAllowedCapabilities();
public A addToAllowedFlexVolumes(java.lang.Integer index, V1beta1AllowedFlexVolume item);
public A setToAllowedFlexVolumes(
java.lang.Integer index, io.kubernetes.client.openapi.models.V1beta1AllowedFlexVolume item);
public A addToAllowedFlexVolumes(
io.kubernetes.client.openapi.models.V1beta1AllowedFlexVolume... items);
public A addAllToAllowedFlexVolumes(
java.util.Collection items);
public A removeFromAllowedFlexVolumes(
io.kubernetes.client.openapi.models.V1beta1AllowedFlexVolume... items);
public A removeAllFromAllowedFlexVolumes(
java.util.Collection items);
public A removeMatchingFromAllowedFlexVolumes(
java.util.function.Predicate predicate);
/**
* This method has been deprecated, please use method buildAllowedFlexVolumes instead.
*
* @return The buildable object.
*/
@java.lang.Deprecated
public java.util.List
getAllowedFlexVolumes();
public java.util.List
buildAllowedFlexVolumes();
public io.kubernetes.client.openapi.models.V1beta1AllowedFlexVolume buildAllowedFlexVolume(
java.lang.Integer index);
public io.kubernetes.client.openapi.models.V1beta1AllowedFlexVolume buildFirstAllowedFlexVolume();
public io.kubernetes.client.openapi.models.V1beta1AllowedFlexVolume buildLastAllowedFlexVolume();
public io.kubernetes.client.openapi.models.V1beta1AllowedFlexVolume
buildMatchingAllowedFlexVolume(
java.util.function.Predicate<
io.kubernetes.client.openapi.models.V1beta1AllowedFlexVolumeBuilder>
predicate);
public java.lang.Boolean hasMatchingAllowedFlexVolume(
java.util.function.Predicate<
io.kubernetes.client.openapi.models.V1beta1AllowedFlexVolumeBuilder>
predicate);
public A withAllowedFlexVolumes(
java.util.List
allowedFlexVolumes);
public A withAllowedFlexVolumes(
io.kubernetes.client.openapi.models.V1beta1AllowedFlexVolume... allowedFlexVolumes);
public java.lang.Boolean hasAllowedFlexVolumes();
public V1beta1PodSecurityPolicySpecFluent.AllowedFlexVolumesNested addNewAllowedFlexVolume();
public io.kubernetes.client.openapi.models.V1beta1PodSecurityPolicySpecFluent
.AllowedFlexVolumesNested<
A>
addNewAllowedFlexVolumeLike(
io.kubernetes.client.openapi.models.V1beta1AllowedFlexVolume item);
public io.kubernetes.client.openapi.models.V1beta1PodSecurityPolicySpecFluent
.AllowedFlexVolumesNested<
A>
setNewAllowedFlexVolumeLike(
java.lang.Integer index,
io.kubernetes.client.openapi.models.V1beta1AllowedFlexVolume item);
public io.kubernetes.client.openapi.models.V1beta1PodSecurityPolicySpecFluent
.AllowedFlexVolumesNested<
A>
editAllowedFlexVolume(java.lang.Integer index);
public io.kubernetes.client.openapi.models.V1beta1PodSecurityPolicySpecFluent
.AllowedFlexVolumesNested<
A>
editFirstAllowedFlexVolume();
public io.kubernetes.client.openapi.models.V1beta1PodSecurityPolicySpecFluent
.AllowedFlexVolumesNested<
A>
editLastAllowedFlexVolume();
public io.kubernetes.client.openapi.models.V1beta1PodSecurityPolicySpecFluent
.AllowedFlexVolumesNested<
A>
editMatchingAllowedFlexVolume(
java.util.function.Predicate<
io.kubernetes.client.openapi.models.V1beta1AllowedFlexVolumeBuilder>
predicate);
public A addToAllowedHostPaths(java.lang.Integer index, V1beta1AllowedHostPath item);
public A setToAllowedHostPaths(
java.lang.Integer index, io.kubernetes.client.openapi.models.V1beta1AllowedHostPath item);
public A addToAllowedHostPaths(
io.kubernetes.client.openapi.models.V1beta1AllowedHostPath... items);
public A addAllToAllowedHostPaths(
java.util.Collection items);
public A removeFromAllowedHostPaths(
io.kubernetes.client.openapi.models.V1beta1AllowedHostPath... items);
public A removeAllFromAllowedHostPaths(
java.util.Collection items);
public A removeMatchingFromAllowedHostPaths(
java.util.function.Predicate predicate);
/**
* This method has been deprecated, please use method buildAllowedHostPaths instead.
*
* @return The buildable object.
*/
@java.lang.Deprecated
public java.util.List
getAllowedHostPaths();
public java.util.List
buildAllowedHostPaths();
public io.kubernetes.client.openapi.models.V1beta1AllowedHostPath buildAllowedHostPath(
java.lang.Integer index);
public io.kubernetes.client.openapi.models.V1beta1AllowedHostPath buildFirstAllowedHostPath();
public io.kubernetes.client.openapi.models.V1beta1AllowedHostPath buildLastAllowedHostPath();
public io.kubernetes.client.openapi.models.V1beta1AllowedHostPath buildMatchingAllowedHostPath(
java.util.function.Predicate<
io.kubernetes.client.openapi.models.V1beta1AllowedHostPathBuilder>
predicate);
public java.lang.Boolean hasMatchingAllowedHostPath(
java.util.function.Predicate<
io.kubernetes.client.openapi.models.V1beta1AllowedHostPathBuilder>
predicate);
public A withAllowedHostPaths(
java.util.List allowedHostPaths);
public A withAllowedHostPaths(
io.kubernetes.client.openapi.models.V1beta1AllowedHostPath... allowedHostPaths);
public java.lang.Boolean hasAllowedHostPaths();
public V1beta1PodSecurityPolicySpecFluent.AllowedHostPathsNested addNewAllowedHostPath();
public io.kubernetes.client.openapi.models.V1beta1PodSecurityPolicySpecFluent
.AllowedHostPathsNested<
A>
addNewAllowedHostPathLike(io.kubernetes.client.openapi.models.V1beta1AllowedHostPath item);
public io.kubernetes.client.openapi.models.V1beta1PodSecurityPolicySpecFluent
.AllowedHostPathsNested<
A>
setNewAllowedHostPathLike(
java.lang.Integer index, io.kubernetes.client.openapi.models.V1beta1AllowedHostPath item);
public io.kubernetes.client.openapi.models.V1beta1PodSecurityPolicySpecFluent
.AllowedHostPathsNested<
A>
editAllowedHostPath(java.lang.Integer index);
public io.kubernetes.client.openapi.models.V1beta1PodSecurityPolicySpecFluent
.AllowedHostPathsNested<
A>
editFirstAllowedHostPath();
public io.kubernetes.client.openapi.models.V1beta1PodSecurityPolicySpecFluent
.AllowedHostPathsNested<
A>
editLastAllowedHostPath();
public io.kubernetes.client.openapi.models.V1beta1PodSecurityPolicySpecFluent
.AllowedHostPathsNested<
A>
editMatchingAllowedHostPath(
java.util.function.Predicate<
io.kubernetes.client.openapi.models.V1beta1AllowedHostPathBuilder>
predicate);
public A addToAllowedProcMountTypes(java.lang.Integer index, java.lang.String item);
public A setToAllowedProcMountTypes(java.lang.Integer index, java.lang.String item);
public A addToAllowedProcMountTypes(java.lang.String... items);
public A addAllToAllowedProcMountTypes(java.util.Collection items);
public A removeFromAllowedProcMountTypes(java.lang.String... items);
public A removeAllFromAllowedProcMountTypes(java.util.Collection items);
public java.util.List getAllowedProcMountTypes();
public java.lang.String getAllowedProcMountType(java.lang.Integer index);
public java.lang.String getFirstAllowedProcMountType();
public java.lang.String getLastAllowedProcMountType();
public java.lang.String getMatchingAllowedProcMountType(
java.util.function.Predicate predicate);
public java.lang.Boolean hasMatchingAllowedProcMountType(
java.util.function.Predicate predicate);
public A withAllowedProcMountTypes(java.util.List allowedProcMountTypes);
public A withAllowedProcMountTypes(java.lang.String... allowedProcMountTypes);
public java.lang.Boolean hasAllowedProcMountTypes();
public A addToAllowedUnsafeSysctls(java.lang.Integer index, java.lang.String item);
public A setToAllowedUnsafeSysctls(java.lang.Integer index, java.lang.String item);
public A addToAllowedUnsafeSysctls(java.lang.String... items);
public A addAllToAllowedUnsafeSysctls(java.util.Collection items);
public A removeFromAllowedUnsafeSysctls(java.lang.String... items);
public A removeAllFromAllowedUnsafeSysctls(java.util.Collection items);
public java.util.List getAllowedUnsafeSysctls();
public java.lang.String getAllowedUnsafeSysctl(java.lang.Integer index);
public java.lang.String getFirstAllowedUnsafeSysctl();
public java.lang.String getLastAllowedUnsafeSysctl();
public java.lang.String getMatchingAllowedUnsafeSysctl(
java.util.function.Predicate predicate);
public java.lang.Boolean hasMatchingAllowedUnsafeSysctl(
java.util.function.Predicate predicate);
public A withAllowedUnsafeSysctls(java.util.List allowedUnsafeSysctls);
public A withAllowedUnsafeSysctls(java.lang.String... allowedUnsafeSysctls);
public java.lang.Boolean hasAllowedUnsafeSysctls();
public A addToDefaultAddCapabilities(java.lang.Integer index, java.lang.String item);
public A setToDefaultAddCapabilities(java.lang.Integer index, java.lang.String item);
public A addToDefaultAddCapabilities(java.lang.String... items);
public A addAllToDefaultAddCapabilities(java.util.Collection items);
public A removeFromDefaultAddCapabilities(java.lang.String... items);
public A removeAllFromDefaultAddCapabilities(java.util.Collection items);
public java.util.List getDefaultAddCapabilities();
public java.lang.String getDefaultAddCapability(java.lang.Integer index);
public java.lang.String getFirstDefaultAddCapability();
public java.lang.String getLastDefaultAddCapability();
public java.lang.String getMatchingDefaultAddCapability(
java.util.function.Predicate predicate);
public java.lang.Boolean hasMatchingDefaultAddCapability(
java.util.function.Predicate predicate);
public A withDefaultAddCapabilities(java.util.List defaultAddCapabilities);
public A withDefaultAddCapabilities(java.lang.String... defaultAddCapabilities);
public java.lang.Boolean hasDefaultAddCapabilities();
public java.lang.Boolean getDefaultAllowPrivilegeEscalation();
public A withDefaultAllowPrivilegeEscalation(java.lang.Boolean defaultAllowPrivilegeEscalation);
public java.lang.Boolean hasDefaultAllowPrivilegeEscalation();
public A addToForbiddenSysctls(java.lang.Integer index, java.lang.String item);
public A setToForbiddenSysctls(java.lang.Integer index, java.lang.String item);
public A addToForbiddenSysctls(java.lang.String... items);
public A addAllToForbiddenSysctls(java.util.Collection items);
public A removeFromForbiddenSysctls(java.lang.String... items);
public A removeAllFromForbiddenSysctls(java.util.Collection items);
public java.util.List getForbiddenSysctls();
public java.lang.String getForbiddenSysctl(java.lang.Integer index);
public java.lang.String getFirstForbiddenSysctl();
public java.lang.String getLastForbiddenSysctl();
public java.lang.String getMatchingForbiddenSysctl(
java.util.function.Predicate predicate);
public java.lang.Boolean hasMatchingForbiddenSysctl(
java.util.function.Predicate predicate);
public A withForbiddenSysctls(java.util.List forbiddenSysctls);
public A withForbiddenSysctls(java.lang.String... forbiddenSysctls);
public java.lang.Boolean hasForbiddenSysctls();
/**
* This method has been deprecated, please use method buildFsGroup instead.
*
* @return The buildable object.
*/
@java.lang.Deprecated
public V1beta1FSGroupStrategyOptions getFsGroup();
public io.kubernetes.client.openapi.models.V1beta1FSGroupStrategyOptions buildFsGroup();
public A withFsGroup(io.kubernetes.client.openapi.models.V1beta1FSGroupStrategyOptions fsGroup);
public java.lang.Boolean hasFsGroup();
public V1beta1PodSecurityPolicySpecFluent.FsGroupNested withNewFsGroup();
public io.kubernetes.client.openapi.models.V1beta1PodSecurityPolicySpecFluent.FsGroupNested
withNewFsGroupLike(io.kubernetes.client.openapi.models.V1beta1FSGroupStrategyOptions item);
public io.kubernetes.client.openapi.models.V1beta1PodSecurityPolicySpecFluent.FsGroupNested
editFsGroup();
public io.kubernetes.client.openapi.models.V1beta1PodSecurityPolicySpecFluent.FsGroupNested
editOrNewFsGroup();
public io.kubernetes.client.openapi.models.V1beta1PodSecurityPolicySpecFluent.FsGroupNested
editOrNewFsGroupLike(io.kubernetes.client.openapi.models.V1beta1FSGroupStrategyOptions item);
public java.lang.Boolean getHostIPC();
public A withHostIPC(java.lang.Boolean hostIPC);
public java.lang.Boolean hasHostIPC();
public java.lang.Boolean getHostNetwork();
public A withHostNetwork(java.lang.Boolean hostNetwork);
public java.lang.Boolean hasHostNetwork();
public java.lang.Boolean getHostPID();
public A withHostPID(java.lang.Boolean hostPID);
public java.lang.Boolean hasHostPID();
public A addToHostPorts(java.lang.Integer index, V1beta1HostPortRange item);
public A setToHostPorts(
java.lang.Integer index, io.kubernetes.client.openapi.models.V1beta1HostPortRange item);
public A addToHostPorts(io.kubernetes.client.openapi.models.V1beta1HostPortRange... items);
public A addAllToHostPorts(
java.util.Collection items);
public A removeFromHostPorts(io.kubernetes.client.openapi.models.V1beta1HostPortRange... items);
public A removeAllFromHostPorts(
java.util.Collection items);
public A removeMatchingFromHostPorts(
java.util.function.Predicate predicate);
/**
* This method has been deprecated, please use method buildHostPorts instead.
*
* @return The buildable object.
*/
@java.lang.Deprecated
public java.util.List getHostPorts();
public java.util.List buildHostPorts();
public io.kubernetes.client.openapi.models.V1beta1HostPortRange buildHostPort(
java.lang.Integer index);
public io.kubernetes.client.openapi.models.V1beta1HostPortRange buildFirstHostPort();
public io.kubernetes.client.openapi.models.V1beta1HostPortRange buildLastHostPort();
public io.kubernetes.client.openapi.models.V1beta1HostPortRange buildMatchingHostPort(
java.util.function.Predicate
predicate);
public java.lang.Boolean hasMatchingHostPort(
java.util.function.Predicate
predicate);
public A withHostPorts(
java.util.List hostPorts);
public A withHostPorts(io.kubernetes.client.openapi.models.V1beta1HostPortRange... hostPorts);
public java.lang.Boolean hasHostPorts();
public V1beta1PodSecurityPolicySpecFluent.HostPortsNested addNewHostPort();
public io.kubernetes.client.openapi.models.V1beta1PodSecurityPolicySpecFluent.HostPortsNested
addNewHostPortLike(io.kubernetes.client.openapi.models.V1beta1HostPortRange item);
public io.kubernetes.client.openapi.models.V1beta1PodSecurityPolicySpecFluent.HostPortsNested
setNewHostPortLike(
java.lang.Integer index, io.kubernetes.client.openapi.models.V1beta1HostPortRange item);
public io.kubernetes.client.openapi.models.V1beta1PodSecurityPolicySpecFluent.HostPortsNested
editHostPort(java.lang.Integer index);
public io.kubernetes.client.openapi.models.V1beta1PodSecurityPolicySpecFluent.HostPortsNested
editFirstHostPort();
public io.kubernetes.client.openapi.models.V1beta1PodSecurityPolicySpecFluent.HostPortsNested
editLastHostPort();
public io.kubernetes.client.openapi.models.V1beta1PodSecurityPolicySpecFluent.HostPortsNested
editMatchingHostPort(
java.util.function.Predicate<
io.kubernetes.client.openapi.models.V1beta1HostPortRangeBuilder>
predicate);
public java.lang.Boolean getPrivileged();
public A withPrivileged(java.lang.Boolean privileged);
public java.lang.Boolean hasPrivileged();
public java.lang.Boolean getReadOnlyRootFilesystem();
public A withReadOnlyRootFilesystem(java.lang.Boolean readOnlyRootFilesystem);
public java.lang.Boolean hasReadOnlyRootFilesystem();
public A addToRequiredDropCapabilities(java.lang.Integer index, java.lang.String item);
public A setToRequiredDropCapabilities(java.lang.Integer index, java.lang.String item);
public A addToRequiredDropCapabilities(java.lang.String... items);
public A addAllToRequiredDropCapabilities(java.util.Collection items);
public A removeFromRequiredDropCapabilities(java.lang.String... items);
public A removeAllFromRequiredDropCapabilities(java.util.Collection items);
public java.util.List getRequiredDropCapabilities();
public java.lang.String getRequiredDropCapability(java.lang.Integer index);
public java.lang.String getFirstRequiredDropCapability();
public java.lang.String getLastRequiredDropCapability();
public java.lang.String getMatchingRequiredDropCapability(
java.util.function.Predicate predicate);
public java.lang.Boolean hasMatchingRequiredDropCapability(
java.util.function.Predicate predicate);
public A withRequiredDropCapabilities(java.util.List requiredDropCapabilities);
public A withRequiredDropCapabilities(java.lang.String... requiredDropCapabilities);
public java.lang.Boolean hasRequiredDropCapabilities();
/**
* This method has been deprecated, please use method buildRunAsGroup instead.
*
* @return The buildable object.
*/
@java.lang.Deprecated
public V1beta1RunAsGroupStrategyOptions getRunAsGroup();
public io.kubernetes.client.openapi.models.V1beta1RunAsGroupStrategyOptions buildRunAsGroup();
public A withRunAsGroup(
io.kubernetes.client.openapi.models.V1beta1RunAsGroupStrategyOptions runAsGroup);
public java.lang.Boolean hasRunAsGroup();
public V1beta1PodSecurityPolicySpecFluent.RunAsGroupNested withNewRunAsGroup();
public io.kubernetes.client.openapi.models.V1beta1PodSecurityPolicySpecFluent.RunAsGroupNested
withNewRunAsGroupLike(
io.kubernetes.client.openapi.models.V1beta1RunAsGroupStrategyOptions item);
public io.kubernetes.client.openapi.models.V1beta1PodSecurityPolicySpecFluent.RunAsGroupNested
editRunAsGroup();
public io.kubernetes.client.openapi.models.V1beta1PodSecurityPolicySpecFluent.RunAsGroupNested
editOrNewRunAsGroup();
public io.kubernetes.client.openapi.models.V1beta1PodSecurityPolicySpecFluent.RunAsGroupNested
editOrNewRunAsGroupLike(
io.kubernetes.client.openapi.models.V1beta1RunAsGroupStrategyOptions item);
/**
* This method has been deprecated, please use method buildRunAsUser instead.
*
* @return The buildable object.
*/
@java.lang.Deprecated
public V1beta1RunAsUserStrategyOptions getRunAsUser();
public io.kubernetes.client.openapi.models.V1beta1RunAsUserStrategyOptions buildRunAsUser();
public A withRunAsUser(
io.kubernetes.client.openapi.models.V1beta1RunAsUserStrategyOptions runAsUser);
public java.lang.Boolean hasRunAsUser();
public V1beta1PodSecurityPolicySpecFluent.RunAsUserNested withNewRunAsUser();
public io.kubernetes.client.openapi.models.V1beta1PodSecurityPolicySpecFluent.RunAsUserNested
withNewRunAsUserLike(
io.kubernetes.client.openapi.models.V1beta1RunAsUserStrategyOptions item);
public io.kubernetes.client.openapi.models.V1beta1PodSecurityPolicySpecFluent.RunAsUserNested
editRunAsUser();
public io.kubernetes.client.openapi.models.V1beta1PodSecurityPolicySpecFluent.RunAsUserNested
editOrNewRunAsUser();
public io.kubernetes.client.openapi.models.V1beta1PodSecurityPolicySpecFluent.RunAsUserNested
editOrNewRunAsUserLike(
io.kubernetes.client.openapi.models.V1beta1RunAsUserStrategyOptions item);
/**
* This method has been deprecated, please use method buildRuntimeClass instead.
*
* @return The buildable object.
*/
@java.lang.Deprecated
public V1beta1RuntimeClassStrategyOptions getRuntimeClass();
public io.kubernetes.client.openapi.models.V1beta1RuntimeClassStrategyOptions buildRuntimeClass();
public A withRuntimeClass(
io.kubernetes.client.openapi.models.V1beta1RuntimeClassStrategyOptions runtimeClass);
public java.lang.Boolean hasRuntimeClass();
public V1beta1PodSecurityPolicySpecFluent.RuntimeClassNested withNewRuntimeClass();
public io.kubernetes.client.openapi.models.V1beta1PodSecurityPolicySpecFluent.RuntimeClassNested<
A>
withNewRuntimeClassLike(
io.kubernetes.client.openapi.models.V1beta1RuntimeClassStrategyOptions item);
public io.kubernetes.client.openapi.models.V1beta1PodSecurityPolicySpecFluent.RuntimeClassNested<
A>
editRuntimeClass();
public io.kubernetes.client.openapi.models.V1beta1PodSecurityPolicySpecFluent.RuntimeClassNested<
A>
editOrNewRuntimeClass();
public io.kubernetes.client.openapi.models.V1beta1PodSecurityPolicySpecFluent.RuntimeClassNested<
A>
editOrNewRuntimeClassLike(
io.kubernetes.client.openapi.models.V1beta1RuntimeClassStrategyOptions item);
/**
* This method has been deprecated, please use method buildSeLinux instead.
*
* @return The buildable object.
*/
@java.lang.Deprecated
public V1beta1SELinuxStrategyOptions getSeLinux();
public io.kubernetes.client.openapi.models.V1beta1SELinuxStrategyOptions buildSeLinux();
public A withSeLinux(io.kubernetes.client.openapi.models.V1beta1SELinuxStrategyOptions seLinux);
public java.lang.Boolean hasSeLinux();
public V1beta1PodSecurityPolicySpecFluent.SeLinuxNested withNewSeLinux();
public io.kubernetes.client.openapi.models.V1beta1PodSecurityPolicySpecFluent.SeLinuxNested
withNewSeLinuxLike(io.kubernetes.client.openapi.models.V1beta1SELinuxStrategyOptions item);
public io.kubernetes.client.openapi.models.V1beta1PodSecurityPolicySpecFluent.SeLinuxNested
editSeLinux();
public io.kubernetes.client.openapi.models.V1beta1PodSecurityPolicySpecFluent.SeLinuxNested
editOrNewSeLinux();
public io.kubernetes.client.openapi.models.V1beta1PodSecurityPolicySpecFluent.SeLinuxNested
editOrNewSeLinuxLike(io.kubernetes.client.openapi.models.V1beta1SELinuxStrategyOptions item);
/**
* This method has been deprecated, please use method buildSupplementalGroups instead.
*
* @return The buildable object.
*/
@java.lang.Deprecated
public V1beta1SupplementalGroupsStrategyOptions getSupplementalGroups();
public io.kubernetes.client.openapi.models.V1beta1SupplementalGroupsStrategyOptions
buildSupplementalGroups();
public A withSupplementalGroups(
io.kubernetes.client.openapi.models.V1beta1SupplementalGroupsStrategyOptions
supplementalGroups);
public java.lang.Boolean hasSupplementalGroups();
public V1beta1PodSecurityPolicySpecFluent.SupplementalGroupsNested withNewSupplementalGroups();
public io.kubernetes.client.openapi.models.V1beta1PodSecurityPolicySpecFluent
.SupplementalGroupsNested<
A>
withNewSupplementalGroupsLike(
io.kubernetes.client.openapi.models.V1beta1SupplementalGroupsStrategyOptions item);
public io.kubernetes.client.openapi.models.V1beta1PodSecurityPolicySpecFluent
.SupplementalGroupsNested<
A>
editSupplementalGroups();
public io.kubernetes.client.openapi.models.V1beta1PodSecurityPolicySpecFluent
.SupplementalGroupsNested<
A>
editOrNewSupplementalGroups();
public io.kubernetes.client.openapi.models.V1beta1PodSecurityPolicySpecFluent
.SupplementalGroupsNested<
A>
editOrNewSupplementalGroupsLike(
io.kubernetes.client.openapi.models.V1beta1SupplementalGroupsStrategyOptions item);
public A addToVolumes(java.lang.Integer index, java.lang.String item);
public A setToVolumes(java.lang.Integer index, java.lang.String item);
public A addToVolumes(java.lang.String... items);
public A addAllToVolumes(java.util.Collection items);
public A removeFromVolumes(java.lang.String... items);
public A removeAllFromVolumes(java.util.Collection items);
public java.util.List getVolumes();
public java.lang.String getVolume(java.lang.Integer index);
public java.lang.String getFirstVolume();
public java.lang.String getLastVolume();
public java.lang.String getMatchingVolume(
java.util.function.Predicate predicate);
public java.lang.Boolean hasMatchingVolume(
java.util.function.Predicate predicate);
public A withVolumes(java.util.List volumes);
public A withVolumes(java.lang.String... volumes);
public java.lang.Boolean hasVolumes();
public A withAllowPrivilegeEscalation();
public A withDefaultAllowPrivilegeEscalation();
public A withHostIPC();
public A withHostNetwork();
public A withHostPID();
public A withPrivileged();
public A withReadOnlyRootFilesystem();
public interface AllowedCSIDriversNested
extends Nested,
V1beta1AllowedCSIDriverFluent<
V1beta1PodSecurityPolicySpecFluent.AllowedCSIDriversNested> {
public N and();
public N endAllowedCSIDriver();
}
public interface AllowedFlexVolumesNested
extends io.kubernetes.client.fluent.Nested,
V1beta1AllowedFlexVolumeFluent<
V1beta1PodSecurityPolicySpecFluent.AllowedFlexVolumesNested> {
public N and();
public N endAllowedFlexVolume();
}
public interface AllowedHostPathsNested
extends io.kubernetes.client.fluent.Nested,
V1beta1AllowedHostPathFluent<
V1beta1PodSecurityPolicySpecFluent.AllowedHostPathsNested> {
public N and();
public N endAllowedHostPath();
}
public interface FsGroupNested
extends io.kubernetes.client.fluent.Nested,
V1beta1FSGroupStrategyOptionsFluent> {
public N and();
public N endFsGroup();
}
public interface HostPortsNested
extends io.kubernetes.client.fluent.Nested,
V1beta1HostPortRangeFluent> {
public N and();
public N endHostPort();
}
public interface RunAsGroupNested
extends io.kubernetes.client.fluent.Nested,
V1beta1RunAsGroupStrategyOptionsFluent<
V1beta1PodSecurityPolicySpecFluent.RunAsGroupNested> {
public N and();
public N endRunAsGroup();
}
public interface RunAsUserNested
extends io.kubernetes.client.fluent.Nested,
V1beta1RunAsUserStrategyOptionsFluent<
V1beta1PodSecurityPolicySpecFluent.RunAsUserNested> {
public N and();
public N endRunAsUser();
}
public interface RuntimeClassNested
extends io.kubernetes.client.fluent.Nested,
V1beta1RuntimeClassStrategyOptionsFluent<
V1beta1PodSecurityPolicySpecFluent.RuntimeClassNested> {
public N and();
public N endRuntimeClass();
}
public interface SeLinuxNested
extends io.kubernetes.client.fluent.Nested,
V1beta1SELinuxStrategyOptionsFluent> {
public N and();
public N endSeLinux();
}
public interface SupplementalGroupsNested
extends io.kubernetes.client.fluent.Nested,
V1beta1SupplementalGroupsStrategyOptionsFluent<
V1beta1PodSecurityPolicySpecFluent.SupplementalGroupsNested> {
public N and();
public N endSupplementalGroups();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy