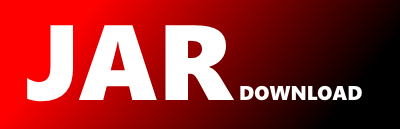
io.kubernetes.client.openapi.models.V1EndpointSubsetFluent Maven / Gradle / Ivy
/*
Copyright 2022 The Kubernetes Authors.
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package io.kubernetes.client.openapi.models;
import io.kubernetes.client.fluent.Fluent;
import io.kubernetes.client.fluent.Nested;
import java.util.Collection;
import java.util.List;
import java.util.function.Predicate;
/** Generated */
public interface V1EndpointSubsetFluent> extends Fluent {
public A addToAddresses(Integer index, V1EndpointAddress item);
public A setToAddresses(
java.lang.Integer index, io.kubernetes.client.openapi.models.V1EndpointAddress item);
public A addToAddresses(io.kubernetes.client.openapi.models.V1EndpointAddress... items);
public A addAllToAddresses(
Collection items);
public A removeFromAddresses(io.kubernetes.client.openapi.models.V1EndpointAddress... items);
public A removeAllFromAddresses(
java.util.Collection items);
public A removeMatchingFromAddresses(Predicate predicate);
/**
* This method has been deprecated, please use method buildAddresses instead.
*
* @return The buildable object.
*/
@Deprecated
public List getAddresses();
public java.util.List buildAddresses();
public io.kubernetes.client.openapi.models.V1EndpointAddress buildAddress(
java.lang.Integer index);
public io.kubernetes.client.openapi.models.V1EndpointAddress buildFirstAddress();
public io.kubernetes.client.openapi.models.V1EndpointAddress buildLastAddress();
public io.kubernetes.client.openapi.models.V1EndpointAddress buildMatchingAddress(
java.util.function.Predicate
predicate);
public Boolean hasMatchingAddress(
java.util.function.Predicate
predicate);
public A withAddresses(
java.util.List addresses);
public A withAddresses(io.kubernetes.client.openapi.models.V1EndpointAddress... addresses);
public java.lang.Boolean hasAddresses();
public V1EndpointSubsetFluent.AddressesNested addNewAddress();
public io.kubernetes.client.openapi.models.V1EndpointSubsetFluent.AddressesNested
addNewAddressLike(io.kubernetes.client.openapi.models.V1EndpointAddress item);
public io.kubernetes.client.openapi.models.V1EndpointSubsetFluent.AddressesNested
setNewAddressLike(
java.lang.Integer index, io.kubernetes.client.openapi.models.V1EndpointAddress item);
public io.kubernetes.client.openapi.models.V1EndpointSubsetFluent.AddressesNested editAddress(
java.lang.Integer index);
public io.kubernetes.client.openapi.models.V1EndpointSubsetFluent.AddressesNested
editFirstAddress();
public io.kubernetes.client.openapi.models.V1EndpointSubsetFluent.AddressesNested
editLastAddress();
public io.kubernetes.client.openapi.models.V1EndpointSubsetFluent.AddressesNested
editMatchingAddress(
java.util.function.Predicate
predicate);
public A addToNotReadyAddresses(
java.lang.Integer index, io.kubernetes.client.openapi.models.V1EndpointAddress item);
public A setToNotReadyAddresses(
java.lang.Integer index, io.kubernetes.client.openapi.models.V1EndpointAddress item);
public A addToNotReadyAddresses(io.kubernetes.client.openapi.models.V1EndpointAddress... items);
public A addAllToNotReadyAddresses(
java.util.Collection items);
public A removeFromNotReadyAddresses(
io.kubernetes.client.openapi.models.V1EndpointAddress... items);
public A removeAllFromNotReadyAddresses(
java.util.Collection items);
public A removeMatchingFromNotReadyAddresses(
java.util.function.Predicate
predicate);
/**
* This method has been deprecated, please use method buildNotReadyAddresses instead.
*
* @return The buildable object.
*/
@java.lang.Deprecated
public java.util.List
getNotReadyAddresses();
public java.util.List
buildNotReadyAddresses();
public io.kubernetes.client.openapi.models.V1EndpointAddress buildNotReadyAddress(
java.lang.Integer index);
public io.kubernetes.client.openapi.models.V1EndpointAddress buildFirstNotReadyAddress();
public io.kubernetes.client.openapi.models.V1EndpointAddress buildLastNotReadyAddress();
public io.kubernetes.client.openapi.models.V1EndpointAddress buildMatchingNotReadyAddress(
java.util.function.Predicate
predicate);
public java.lang.Boolean hasMatchingNotReadyAddress(
java.util.function.Predicate
predicate);
public A withNotReadyAddresses(
java.util.List notReadyAddresses);
public A withNotReadyAddresses(
io.kubernetes.client.openapi.models.V1EndpointAddress... notReadyAddresses);
public java.lang.Boolean hasNotReadyAddresses();
public V1EndpointSubsetFluent.NotReadyAddressesNested addNewNotReadyAddress();
public io.kubernetes.client.openapi.models.V1EndpointSubsetFluent.NotReadyAddressesNested
addNewNotReadyAddressLike(io.kubernetes.client.openapi.models.V1EndpointAddress item);
public io.kubernetes.client.openapi.models.V1EndpointSubsetFluent.NotReadyAddressesNested
setNewNotReadyAddressLike(
java.lang.Integer index, io.kubernetes.client.openapi.models.V1EndpointAddress item);
public io.kubernetes.client.openapi.models.V1EndpointSubsetFluent.NotReadyAddressesNested
editNotReadyAddress(java.lang.Integer index);
public io.kubernetes.client.openapi.models.V1EndpointSubsetFluent.NotReadyAddressesNested
editFirstNotReadyAddress();
public io.kubernetes.client.openapi.models.V1EndpointSubsetFluent.NotReadyAddressesNested
editLastNotReadyAddress();
public io.kubernetes.client.openapi.models.V1EndpointSubsetFluent.NotReadyAddressesNested
editMatchingNotReadyAddress(
java.util.function.Predicate
predicate);
public A addToPorts(java.lang.Integer index, CoreV1EndpointPort item);
public A setToPorts(
java.lang.Integer index, io.kubernetes.client.openapi.models.CoreV1EndpointPort item);
public A addToPorts(io.kubernetes.client.openapi.models.CoreV1EndpointPort... items);
public A addAllToPorts(
java.util.Collection items);
public A removeFromPorts(io.kubernetes.client.openapi.models.CoreV1EndpointPort... items);
public A removeAllFromPorts(
java.util.Collection items);
public A removeMatchingFromPorts(
java.util.function.Predicate predicate);
/**
* This method has been deprecated, please use method buildPorts instead.
*
* @return The buildable object.
*/
@java.lang.Deprecated
public java.util.List getPorts();
public java.util.List buildPorts();
public io.kubernetes.client.openapi.models.CoreV1EndpointPort buildPort(java.lang.Integer index);
public io.kubernetes.client.openapi.models.CoreV1EndpointPort buildFirstPort();
public io.kubernetes.client.openapi.models.CoreV1EndpointPort buildLastPort();
public io.kubernetes.client.openapi.models.CoreV1EndpointPort buildMatchingPort(
java.util.function.Predicate
predicate);
public java.lang.Boolean hasMatchingPort(
java.util.function.Predicate
predicate);
public A withPorts(java.util.List ports);
public A withPorts(io.kubernetes.client.openapi.models.CoreV1EndpointPort... ports);
public java.lang.Boolean hasPorts();
public V1EndpointSubsetFluent.PortsNested addNewPort();
public io.kubernetes.client.openapi.models.V1EndpointSubsetFluent.PortsNested addNewPortLike(
io.kubernetes.client.openapi.models.CoreV1EndpointPort item);
public io.kubernetes.client.openapi.models.V1EndpointSubsetFluent.PortsNested setNewPortLike(
java.lang.Integer index, io.kubernetes.client.openapi.models.CoreV1EndpointPort item);
public io.kubernetes.client.openapi.models.V1EndpointSubsetFluent.PortsNested editPort(
java.lang.Integer index);
public io.kubernetes.client.openapi.models.V1EndpointSubsetFluent.PortsNested editFirstPort();
public io.kubernetes.client.openapi.models.V1EndpointSubsetFluent.PortsNested editLastPort();
public io.kubernetes.client.openapi.models.V1EndpointSubsetFluent.PortsNested editMatchingPort(
java.util.function.Predicate
predicate);
public interface AddressesNested
extends Nested, V1EndpointAddressFluent> {
public N and();
public N endAddress();
}
public interface NotReadyAddressesNested
extends io.kubernetes.client.fluent.Nested,
V1EndpointAddressFluent> {
public N and();
public N endNotReadyAddress();
}
public interface PortsNested
extends io.kubernetes.client.fluent.Nested,
CoreV1EndpointPortFluent> {
public N and();
public N endPort();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy