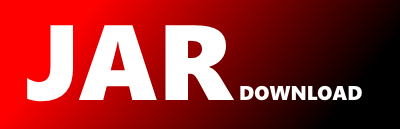
io.kubernetes.client.openapi.models.V1SecurityContextFluent Maven / Gradle / Ivy
/*
Copyright 2022 The Kubernetes Authors.
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package io.kubernetes.client.openapi.models;
import io.kubernetes.client.fluent.Fluent;
import io.kubernetes.client.fluent.Nested;
/** Generated */
public interface V1SecurityContextFluent> extends Fluent {
public Boolean getAllowPrivilegeEscalation();
public A withAllowPrivilegeEscalation(java.lang.Boolean allowPrivilegeEscalation);
public java.lang.Boolean hasAllowPrivilegeEscalation();
/**
* This method has been deprecated, please use method buildCapabilities instead.
*
* @return The buildable object.
*/
@Deprecated
public V1Capabilities getCapabilities();
public io.kubernetes.client.openapi.models.V1Capabilities buildCapabilities();
public A withCapabilities(io.kubernetes.client.openapi.models.V1Capabilities capabilities);
public java.lang.Boolean hasCapabilities();
public V1SecurityContextFluent.CapabilitiesNested withNewCapabilities();
public io.kubernetes.client.openapi.models.V1SecurityContextFluent.CapabilitiesNested
withNewCapabilitiesLike(io.kubernetes.client.openapi.models.V1Capabilities item);
public io.kubernetes.client.openapi.models.V1SecurityContextFluent.CapabilitiesNested
editCapabilities();
public io.kubernetes.client.openapi.models.V1SecurityContextFluent.CapabilitiesNested
editOrNewCapabilities();
public io.kubernetes.client.openapi.models.V1SecurityContextFluent.CapabilitiesNested
editOrNewCapabilitiesLike(io.kubernetes.client.openapi.models.V1Capabilities item);
public java.lang.Boolean getPrivileged();
public A withPrivileged(java.lang.Boolean privileged);
public java.lang.Boolean hasPrivileged();
public String getProcMount();
public A withProcMount(java.lang.String procMount);
public java.lang.Boolean hasProcMount();
public java.lang.Boolean getReadOnlyRootFilesystem();
public A withReadOnlyRootFilesystem(java.lang.Boolean readOnlyRootFilesystem);
public java.lang.Boolean hasReadOnlyRootFilesystem();
public Long getRunAsGroup();
public A withRunAsGroup(java.lang.Long runAsGroup);
public java.lang.Boolean hasRunAsGroup();
public java.lang.Boolean getRunAsNonRoot();
public A withRunAsNonRoot(java.lang.Boolean runAsNonRoot);
public java.lang.Boolean hasRunAsNonRoot();
public java.lang.Long getRunAsUser();
public A withRunAsUser(java.lang.Long runAsUser);
public java.lang.Boolean hasRunAsUser();
/**
* This method has been deprecated, please use method buildSeLinuxOptions instead.
*
* @return The buildable object.
*/
@java.lang.Deprecated
public V1SELinuxOptions getSeLinuxOptions();
public io.kubernetes.client.openapi.models.V1SELinuxOptions buildSeLinuxOptions();
public A withSeLinuxOptions(io.kubernetes.client.openapi.models.V1SELinuxOptions seLinuxOptions);
public java.lang.Boolean hasSeLinuxOptions();
public V1SecurityContextFluent.SeLinuxOptionsNested withNewSeLinuxOptions();
public io.kubernetes.client.openapi.models.V1SecurityContextFluent.SeLinuxOptionsNested
withNewSeLinuxOptionsLike(io.kubernetes.client.openapi.models.V1SELinuxOptions item);
public io.kubernetes.client.openapi.models.V1SecurityContextFluent.SeLinuxOptionsNested
editSeLinuxOptions();
public io.kubernetes.client.openapi.models.V1SecurityContextFluent.SeLinuxOptionsNested
editOrNewSeLinuxOptions();
public io.kubernetes.client.openapi.models.V1SecurityContextFluent.SeLinuxOptionsNested
editOrNewSeLinuxOptionsLike(io.kubernetes.client.openapi.models.V1SELinuxOptions item);
/**
* This method has been deprecated, please use method buildSeccompProfile instead.
*
* @return The buildable object.
*/
@java.lang.Deprecated
public V1SeccompProfile getSeccompProfile();
public io.kubernetes.client.openapi.models.V1SeccompProfile buildSeccompProfile();
public A withSeccompProfile(io.kubernetes.client.openapi.models.V1SeccompProfile seccompProfile);
public java.lang.Boolean hasSeccompProfile();
public V1SecurityContextFluent.SeccompProfileNested withNewSeccompProfile();
public io.kubernetes.client.openapi.models.V1SecurityContextFluent.SeccompProfileNested
withNewSeccompProfileLike(io.kubernetes.client.openapi.models.V1SeccompProfile item);
public io.kubernetes.client.openapi.models.V1SecurityContextFluent.SeccompProfileNested
editSeccompProfile();
public io.kubernetes.client.openapi.models.V1SecurityContextFluent.SeccompProfileNested
editOrNewSeccompProfile();
public io.kubernetes.client.openapi.models.V1SecurityContextFluent.SeccompProfileNested
editOrNewSeccompProfileLike(io.kubernetes.client.openapi.models.V1SeccompProfile item);
/**
* This method has been deprecated, please use method buildWindowsOptions instead.
*
* @return The buildable object.
*/
@java.lang.Deprecated
public V1WindowsSecurityContextOptions getWindowsOptions();
public io.kubernetes.client.openapi.models.V1WindowsSecurityContextOptions buildWindowsOptions();
public A withWindowsOptions(
io.kubernetes.client.openapi.models.V1WindowsSecurityContextOptions windowsOptions);
public java.lang.Boolean hasWindowsOptions();
public V1SecurityContextFluent.WindowsOptionsNested withNewWindowsOptions();
public io.kubernetes.client.openapi.models.V1SecurityContextFluent.WindowsOptionsNested
withNewWindowsOptionsLike(
io.kubernetes.client.openapi.models.V1WindowsSecurityContextOptions item);
public io.kubernetes.client.openapi.models.V1SecurityContextFluent.WindowsOptionsNested
editWindowsOptions();
public io.kubernetes.client.openapi.models.V1SecurityContextFluent.WindowsOptionsNested
editOrNewWindowsOptions();
public io.kubernetes.client.openapi.models.V1SecurityContextFluent.WindowsOptionsNested
editOrNewWindowsOptionsLike(
io.kubernetes.client.openapi.models.V1WindowsSecurityContextOptions item);
public A withAllowPrivilegeEscalation();
public A withPrivileged();
public A withReadOnlyRootFilesystem();
public A withRunAsNonRoot();
public interface CapabilitiesNested
extends Nested, V1CapabilitiesFluent> {
public N and();
public N endCapabilities();
}
public interface SeLinuxOptionsNested
extends io.kubernetes.client.fluent.Nested,
V1SELinuxOptionsFluent> {
public N and();
public N endSeLinuxOptions();
}
public interface SeccompProfileNested
extends io.kubernetes.client.fluent.Nested,
V1SeccompProfileFluent> {
public N and();
public N endSeccompProfile();
}
public interface WindowsOptionsNested
extends io.kubernetes.client.fluent.Nested,
V1WindowsSecurityContextOptionsFluent> {
public N and();
public N endWindowsOptions();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy