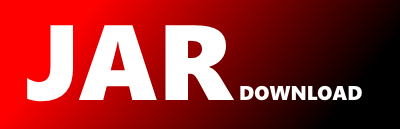
io.kubernetes.client.openapi.models.V1SubjectAccessReviewSpecFluent Maven / Gradle / Ivy
/*
Copyright 2022 The Kubernetes Authors.
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package io.kubernetes.client.openapi.models;
import io.kubernetes.client.fluent.Fluent;
import io.kubernetes.client.fluent.Nested;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.function.Predicate;
/** Generated */
public interface V1SubjectAccessReviewSpecFluent>
extends Fluent {
public A addToExtra(String key, List value);
public A addToExtra(Map> map);
public A removeFromExtra(java.lang.String key);
public A removeFromExtra(java.util.Map> map);
public java.util.Map> getExtra();
public A withExtra(
java.util.Map> extra);
public Boolean hasExtra();
public A addToGroups(Integer index, java.lang.String item);
public A setToGroups(java.lang.Integer index, java.lang.String item);
public A addToGroups(java.lang.String... items);
public A addAllToGroups(Collection items);
public A removeFromGroups(java.lang.String... items);
public A removeAllFromGroups(java.util.Collection items);
public java.util.List getGroups();
public java.lang.String getGroup(java.lang.Integer index);
public java.lang.String getFirstGroup();
public java.lang.String getLastGroup();
public java.lang.String getMatchingGroup(Predicate predicate);
public java.lang.Boolean hasMatchingGroup(
java.util.function.Predicate predicate);
public A withGroups(java.util.List groups);
public A withGroups(java.lang.String... groups);
public java.lang.Boolean hasGroups();
/**
* This method has been deprecated, please use method buildNonResourceAttributes instead.
*
* @return The buildable object.
*/
@Deprecated
public V1NonResourceAttributes getNonResourceAttributes();
public io.kubernetes.client.openapi.models.V1NonResourceAttributes buildNonResourceAttributes();
public A withNonResourceAttributes(
io.kubernetes.client.openapi.models.V1NonResourceAttributes nonResourceAttributes);
public java.lang.Boolean hasNonResourceAttributes();
public V1SubjectAccessReviewSpecFluent.NonResourceAttributesNested
withNewNonResourceAttributes();
public io.kubernetes.client.openapi.models.V1SubjectAccessReviewSpecFluent
.NonResourceAttributesNested<
A>
withNewNonResourceAttributesLike(
io.kubernetes.client.openapi.models.V1NonResourceAttributes item);
public io.kubernetes.client.openapi.models.V1SubjectAccessReviewSpecFluent
.NonResourceAttributesNested<
A>
editNonResourceAttributes();
public io.kubernetes.client.openapi.models.V1SubjectAccessReviewSpecFluent
.NonResourceAttributesNested<
A>
editOrNewNonResourceAttributes();
public io.kubernetes.client.openapi.models.V1SubjectAccessReviewSpecFluent
.NonResourceAttributesNested<
A>
editOrNewNonResourceAttributesLike(
io.kubernetes.client.openapi.models.V1NonResourceAttributes item);
/**
* This method has been deprecated, please use method buildResourceAttributes instead.
*
* @return The buildable object.
*/
@java.lang.Deprecated
public V1ResourceAttributes getResourceAttributes();
public io.kubernetes.client.openapi.models.V1ResourceAttributes buildResourceAttributes();
public A withResourceAttributes(
io.kubernetes.client.openapi.models.V1ResourceAttributes resourceAttributes);
public java.lang.Boolean hasResourceAttributes();
public V1SubjectAccessReviewSpecFluent.ResourceAttributesNested withNewResourceAttributes();
public io.kubernetes.client.openapi.models.V1SubjectAccessReviewSpecFluent
.ResourceAttributesNested<
A>
withNewResourceAttributesLike(io.kubernetes.client.openapi.models.V1ResourceAttributes item);
public io.kubernetes.client.openapi.models.V1SubjectAccessReviewSpecFluent
.ResourceAttributesNested<
A>
editResourceAttributes();
public io.kubernetes.client.openapi.models.V1SubjectAccessReviewSpecFluent
.ResourceAttributesNested<
A>
editOrNewResourceAttributes();
public io.kubernetes.client.openapi.models.V1SubjectAccessReviewSpecFluent
.ResourceAttributesNested<
A>
editOrNewResourceAttributesLike(
io.kubernetes.client.openapi.models.V1ResourceAttributes item);
public java.lang.String getUid();
public A withUid(java.lang.String uid);
public java.lang.Boolean hasUid();
public java.lang.String getUser();
public A withUser(java.lang.String user);
public java.lang.Boolean hasUser();
public interface NonResourceAttributesNested
extends Nested,
V1NonResourceAttributesFluent<
V1SubjectAccessReviewSpecFluent.NonResourceAttributesNested> {
public N and();
public N endNonResourceAttributes();
}
public interface ResourceAttributesNested
extends io.kubernetes.client.fluent.Nested,
V1ResourceAttributesFluent> {
public N and();
public N endResourceAttributes();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy