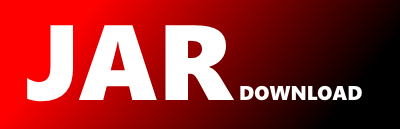
io.kubernetes.client.openapi.models.V1beta2FlowSchemaSpecFluent Maven / Gradle / Ivy
/*
Copyright 2022 The Kubernetes Authors.
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package io.kubernetes.client.openapi.models;
import io.kubernetes.client.fluent.Fluent;
import io.kubernetes.client.fluent.Nested;
import java.util.Collection;
import java.util.List;
import java.util.function.Predicate;
/** Generated */
public interface V1beta2FlowSchemaSpecFluent>
extends Fluent {
/**
* This method has been deprecated, please use method buildDistinguisherMethod instead.
*
* @return The buildable object.
*/
@Deprecated
public V1beta2FlowDistinguisherMethod getDistinguisherMethod();
public io.kubernetes.client.openapi.models.V1beta2FlowDistinguisherMethod
buildDistinguisherMethod();
public A withDistinguisherMethod(
io.kubernetes.client.openapi.models.V1beta2FlowDistinguisherMethod distinguisherMethod);
public Boolean hasDistinguisherMethod();
public V1beta2FlowSchemaSpecFluent.DistinguisherMethodNested withNewDistinguisherMethod();
public io.kubernetes.client.openapi.models.V1beta2FlowSchemaSpecFluent.DistinguisherMethodNested<
A>
withNewDistinguisherMethodLike(
io.kubernetes.client.openapi.models.V1beta2FlowDistinguisherMethod item);
public io.kubernetes.client.openapi.models.V1beta2FlowSchemaSpecFluent.DistinguisherMethodNested<
A>
editDistinguisherMethod();
public io.kubernetes.client.openapi.models.V1beta2FlowSchemaSpecFluent.DistinguisherMethodNested<
A>
editOrNewDistinguisherMethod();
public io.kubernetes.client.openapi.models.V1beta2FlowSchemaSpecFluent.DistinguisherMethodNested<
A>
editOrNewDistinguisherMethodLike(
io.kubernetes.client.openapi.models.V1beta2FlowDistinguisherMethod item);
public Integer getMatchingPrecedence();
public A withMatchingPrecedence(java.lang.Integer matchingPrecedence);
public java.lang.Boolean hasMatchingPrecedence();
/**
* This method has been deprecated, please use method buildPriorityLevelConfiguration instead.
*
* @return The buildable object.
*/
@java.lang.Deprecated
public V1beta2PriorityLevelConfigurationReference getPriorityLevelConfiguration();
public io.kubernetes.client.openapi.models.V1beta2PriorityLevelConfigurationReference
buildPriorityLevelConfiguration();
public A withPriorityLevelConfiguration(
io.kubernetes.client.openapi.models.V1beta2PriorityLevelConfigurationReference
priorityLevelConfiguration);
public java.lang.Boolean hasPriorityLevelConfiguration();
public V1beta2FlowSchemaSpecFluent.PriorityLevelConfigurationNested
withNewPriorityLevelConfiguration();
public io.kubernetes.client.openapi.models.V1beta2FlowSchemaSpecFluent
.PriorityLevelConfigurationNested<
A>
withNewPriorityLevelConfigurationLike(
io.kubernetes.client.openapi.models.V1beta2PriorityLevelConfigurationReference item);
public io.kubernetes.client.openapi.models.V1beta2FlowSchemaSpecFluent
.PriorityLevelConfigurationNested<
A>
editPriorityLevelConfiguration();
public io.kubernetes.client.openapi.models.V1beta2FlowSchemaSpecFluent
.PriorityLevelConfigurationNested<
A>
editOrNewPriorityLevelConfiguration();
public io.kubernetes.client.openapi.models.V1beta2FlowSchemaSpecFluent
.PriorityLevelConfigurationNested<
A>
editOrNewPriorityLevelConfigurationLike(
io.kubernetes.client.openapi.models.V1beta2PriorityLevelConfigurationReference item);
public A addToRules(java.lang.Integer index, V1beta2PolicyRulesWithSubjects item);
public A setToRules(
java.lang.Integer index,
io.kubernetes.client.openapi.models.V1beta2PolicyRulesWithSubjects item);
public A addToRules(io.kubernetes.client.openapi.models.V1beta2PolicyRulesWithSubjects... items);
public A addAllToRules(
Collection items);
public A removeFromRules(
io.kubernetes.client.openapi.models.V1beta2PolicyRulesWithSubjects... items);
public A removeAllFromRules(
java.util.Collection
items);
public A removeMatchingFromRules(Predicate predicate);
/**
* This method has been deprecated, please use method buildRules instead.
*
* @return The buildable object.
*/
@java.lang.Deprecated
public List getRules();
public java.util.List
buildRules();
public io.kubernetes.client.openapi.models.V1beta2PolicyRulesWithSubjects buildRule(
java.lang.Integer index);
public io.kubernetes.client.openapi.models.V1beta2PolicyRulesWithSubjects buildFirstRule();
public io.kubernetes.client.openapi.models.V1beta2PolicyRulesWithSubjects buildLastRule();
public io.kubernetes.client.openapi.models.V1beta2PolicyRulesWithSubjects buildMatchingRule(
java.util.function.Predicate<
io.kubernetes.client.openapi.models.V1beta2PolicyRulesWithSubjectsBuilder>
predicate);
public java.lang.Boolean hasMatchingRule(
java.util.function.Predicate<
io.kubernetes.client.openapi.models.V1beta2PolicyRulesWithSubjectsBuilder>
predicate);
public A withRules(
java.util.List rules);
public A withRules(io.kubernetes.client.openapi.models.V1beta2PolicyRulesWithSubjects... rules);
public java.lang.Boolean hasRules();
public V1beta2FlowSchemaSpecFluent.RulesNested addNewRule();
public io.kubernetes.client.openapi.models.V1beta2FlowSchemaSpecFluent.RulesNested
addNewRuleLike(io.kubernetes.client.openapi.models.V1beta2PolicyRulesWithSubjects item);
public io.kubernetes.client.openapi.models.V1beta2FlowSchemaSpecFluent.RulesNested
setNewRuleLike(
java.lang.Integer index,
io.kubernetes.client.openapi.models.V1beta2PolicyRulesWithSubjects item);
public io.kubernetes.client.openapi.models.V1beta2FlowSchemaSpecFluent.RulesNested editRule(
java.lang.Integer index);
public io.kubernetes.client.openapi.models.V1beta2FlowSchemaSpecFluent.RulesNested
editFirstRule();
public io.kubernetes.client.openapi.models.V1beta2FlowSchemaSpecFluent.RulesNested
editLastRule();
public io.kubernetes.client.openapi.models.V1beta2FlowSchemaSpecFluent.RulesNested
editMatchingRule(
java.util.function.Predicate<
io.kubernetes.client.openapi.models.V1beta2PolicyRulesWithSubjectsBuilder>
predicate);
public interface DistinguisherMethodNested
extends Nested,
V1beta2FlowDistinguisherMethodFluent<
V1beta2FlowSchemaSpecFluent.DistinguisherMethodNested> {
public N and();
public N endDistinguisherMethod();
}
public interface PriorityLevelConfigurationNested
extends io.kubernetes.client.fluent.Nested,
V1beta2PriorityLevelConfigurationReferenceFluent<
V1beta2FlowSchemaSpecFluent.PriorityLevelConfigurationNested> {
public N and();
public N endPriorityLevelConfiguration();
}
public interface RulesNested
extends io.kubernetes.client.fluent.Nested,
V1beta2PolicyRulesWithSubjectsFluent> {
public N and();
public N endRule();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy