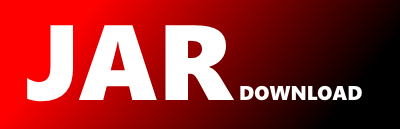
io.kubernetes.client.openapi.models.V1beta2PolicyRulesWithSubjectsFluent Maven / Gradle / Ivy
/*
Copyright 2022 The Kubernetes Authors.
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package io.kubernetes.client.openapi.models;
import io.kubernetes.client.fluent.Fluent;
import io.kubernetes.client.fluent.Nested;
import java.util.Collection;
import java.util.List;
import java.util.function.Predicate;
/** Generated */
public interface V1beta2PolicyRulesWithSubjectsFluent<
A extends V1beta2PolicyRulesWithSubjectsFluent>
extends Fluent {
public A addToNonResourceRules(Integer index, V1beta2NonResourcePolicyRule item);
public A setToNonResourceRules(
java.lang.Integer index,
io.kubernetes.client.openapi.models.V1beta2NonResourcePolicyRule item);
public A addToNonResourceRules(
io.kubernetes.client.openapi.models.V1beta2NonResourcePolicyRule... items);
public A addAllToNonResourceRules(
Collection items);
public A removeFromNonResourceRules(
io.kubernetes.client.openapi.models.V1beta2NonResourcePolicyRule... items);
public A removeAllFromNonResourceRules(
java.util.Collection items);
public A removeMatchingFromNonResourceRules(
Predicate predicate);
/**
* This method has been deprecated, please use method buildNonResourceRules instead.
*
* @return The buildable object.
*/
@Deprecated
public List
getNonResourceRules();
public java.util.List
buildNonResourceRules();
public io.kubernetes.client.openapi.models.V1beta2NonResourcePolicyRule buildNonResourceRule(
java.lang.Integer index);
public io.kubernetes.client.openapi.models.V1beta2NonResourcePolicyRule
buildFirstNonResourceRule();
public io.kubernetes.client.openapi.models.V1beta2NonResourcePolicyRule
buildLastNonResourceRule();
public io.kubernetes.client.openapi.models.V1beta2NonResourcePolicyRule
buildMatchingNonResourceRule(
java.util.function.Predicate<
io.kubernetes.client.openapi.models.V1beta2NonResourcePolicyRuleBuilder>
predicate);
public Boolean hasMatchingNonResourceRule(
java.util.function.Predicate<
io.kubernetes.client.openapi.models.V1beta2NonResourcePolicyRuleBuilder>
predicate);
public A withNonResourceRules(
java.util.List
nonResourceRules);
public A withNonResourceRules(
io.kubernetes.client.openapi.models.V1beta2NonResourcePolicyRule... nonResourceRules);
public java.lang.Boolean hasNonResourceRules();
public V1beta2PolicyRulesWithSubjectsFluent.NonResourceRulesNested addNewNonResourceRule();
public io.kubernetes.client.openapi.models.V1beta2PolicyRulesWithSubjectsFluent
.NonResourceRulesNested<
A>
addNewNonResourceRuleLike(
io.kubernetes.client.openapi.models.V1beta2NonResourcePolicyRule item);
public io.kubernetes.client.openapi.models.V1beta2PolicyRulesWithSubjectsFluent
.NonResourceRulesNested<
A>
setNewNonResourceRuleLike(
java.lang.Integer index,
io.kubernetes.client.openapi.models.V1beta2NonResourcePolicyRule item);
public io.kubernetes.client.openapi.models.V1beta2PolicyRulesWithSubjectsFluent
.NonResourceRulesNested<
A>
editNonResourceRule(java.lang.Integer index);
public io.kubernetes.client.openapi.models.V1beta2PolicyRulesWithSubjectsFluent
.NonResourceRulesNested<
A>
editFirstNonResourceRule();
public io.kubernetes.client.openapi.models.V1beta2PolicyRulesWithSubjectsFluent
.NonResourceRulesNested<
A>
editLastNonResourceRule();
public io.kubernetes.client.openapi.models.V1beta2PolicyRulesWithSubjectsFluent
.NonResourceRulesNested<
A>
editMatchingNonResourceRule(
java.util.function.Predicate<
io.kubernetes.client.openapi.models.V1beta2NonResourcePolicyRuleBuilder>
predicate);
public A addToResourceRules(java.lang.Integer index, V1beta2ResourcePolicyRule item);
public A setToResourceRules(
java.lang.Integer index, io.kubernetes.client.openapi.models.V1beta2ResourcePolicyRule item);
public A addToResourceRules(
io.kubernetes.client.openapi.models.V1beta2ResourcePolicyRule... items);
public A addAllToResourceRules(
java.util.Collection items);
public A removeFromResourceRules(
io.kubernetes.client.openapi.models.V1beta2ResourcePolicyRule... items);
public A removeAllFromResourceRules(
java.util.Collection items);
public A removeMatchingFromResourceRules(
java.util.function.Predicate predicate);
/**
* This method has been deprecated, please use method buildResourceRules instead.
*
* @return The buildable object.
*/
@java.lang.Deprecated
public java.util.List
getResourceRules();
public java.util.List
buildResourceRules();
public io.kubernetes.client.openapi.models.V1beta2ResourcePolicyRule buildResourceRule(
java.lang.Integer index);
public io.kubernetes.client.openapi.models.V1beta2ResourcePolicyRule buildFirstResourceRule();
public io.kubernetes.client.openapi.models.V1beta2ResourcePolicyRule buildLastResourceRule();
public io.kubernetes.client.openapi.models.V1beta2ResourcePolicyRule buildMatchingResourceRule(
java.util.function.Predicate<
io.kubernetes.client.openapi.models.V1beta2ResourcePolicyRuleBuilder>
predicate);
public java.lang.Boolean hasMatchingResourceRule(
java.util.function.Predicate<
io.kubernetes.client.openapi.models.V1beta2ResourcePolicyRuleBuilder>
predicate);
public A withResourceRules(
java.util.List resourceRules);
public A withResourceRules(
io.kubernetes.client.openapi.models.V1beta2ResourcePolicyRule... resourceRules);
public java.lang.Boolean hasResourceRules();
public V1beta2PolicyRulesWithSubjectsFluent.ResourceRulesNested addNewResourceRule();
public io.kubernetes.client.openapi.models.V1beta2PolicyRulesWithSubjectsFluent
.ResourceRulesNested<
A>
addNewResourceRuleLike(io.kubernetes.client.openapi.models.V1beta2ResourcePolicyRule item);
public io.kubernetes.client.openapi.models.V1beta2PolicyRulesWithSubjectsFluent
.ResourceRulesNested<
A>
setNewResourceRuleLike(
java.lang.Integer index,
io.kubernetes.client.openapi.models.V1beta2ResourcePolicyRule item);
public io.kubernetes.client.openapi.models.V1beta2PolicyRulesWithSubjectsFluent
.ResourceRulesNested<
A>
editResourceRule(java.lang.Integer index);
public io.kubernetes.client.openapi.models.V1beta2PolicyRulesWithSubjectsFluent
.ResourceRulesNested<
A>
editFirstResourceRule();
public io.kubernetes.client.openapi.models.V1beta2PolicyRulesWithSubjectsFluent
.ResourceRulesNested<
A>
editLastResourceRule();
public io.kubernetes.client.openapi.models.V1beta2PolicyRulesWithSubjectsFluent
.ResourceRulesNested<
A>
editMatchingResourceRule(
java.util.function.Predicate<
io.kubernetes.client.openapi.models.V1beta2ResourcePolicyRuleBuilder>
predicate);
public A addToSubjects(java.lang.Integer index, V1beta2Subject item);
public A setToSubjects(
java.lang.Integer index, io.kubernetes.client.openapi.models.V1beta2Subject item);
public A addToSubjects(io.kubernetes.client.openapi.models.V1beta2Subject... items);
public A addAllToSubjects(
java.util.Collection items);
public A removeFromSubjects(io.kubernetes.client.openapi.models.V1beta2Subject... items);
public A removeAllFromSubjects(
java.util.Collection items);
public A removeMatchingFromSubjects(
java.util.function.Predicate predicate);
/**
* This method has been deprecated, please use method buildSubjects instead.
*
* @return The buildable object.
*/
@java.lang.Deprecated
public java.util.List getSubjects();
public java.util.List buildSubjects();
public io.kubernetes.client.openapi.models.V1beta2Subject buildSubject(java.lang.Integer index);
public io.kubernetes.client.openapi.models.V1beta2Subject buildFirstSubject();
public io.kubernetes.client.openapi.models.V1beta2Subject buildLastSubject();
public io.kubernetes.client.openapi.models.V1beta2Subject buildMatchingSubject(
java.util.function.Predicate
predicate);
public java.lang.Boolean hasMatchingSubject(
java.util.function.Predicate
predicate);
public A withSubjects(
java.util.List subjects);
public A withSubjects(io.kubernetes.client.openapi.models.V1beta2Subject... subjects);
public java.lang.Boolean hasSubjects();
public V1beta2PolicyRulesWithSubjectsFluent.SubjectsNested addNewSubject();
public io.kubernetes.client.openapi.models.V1beta2PolicyRulesWithSubjectsFluent.SubjectsNested
addNewSubjectLike(io.kubernetes.client.openapi.models.V1beta2Subject item);
public io.kubernetes.client.openapi.models.V1beta2PolicyRulesWithSubjectsFluent.SubjectsNested
setNewSubjectLike(
java.lang.Integer index, io.kubernetes.client.openapi.models.V1beta2Subject item);
public io.kubernetes.client.openapi.models.V1beta2PolicyRulesWithSubjectsFluent.SubjectsNested
editSubject(java.lang.Integer index);
public io.kubernetes.client.openapi.models.V1beta2PolicyRulesWithSubjectsFluent.SubjectsNested
editFirstSubject();
public io.kubernetes.client.openapi.models.V1beta2PolicyRulesWithSubjectsFluent.SubjectsNested
editLastSubject();
public io.kubernetes.client.openapi.models.V1beta2PolicyRulesWithSubjectsFluent.SubjectsNested
editMatchingSubject(
java.util.function.Predicate
predicate);
public interface NonResourceRulesNested
extends Nested,
V1beta2NonResourcePolicyRuleFluent<
V1beta2PolicyRulesWithSubjectsFluent.NonResourceRulesNested> {
public N and();
public N endNonResourceRule();
}
public interface ResourceRulesNested
extends io.kubernetes.client.fluent.Nested,
V1beta2ResourcePolicyRuleFluent<
V1beta2PolicyRulesWithSubjectsFluent.ResourceRulesNested> {
public N and();
public N endResourceRule();
}
public interface SubjectsNested
extends io.kubernetes.client.fluent.Nested,
V1beta2SubjectFluent> {
public N and();
public N endSubject();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy