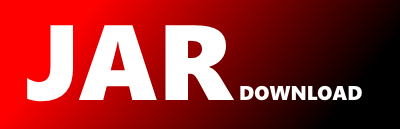
io.kubernetes.client.openapi.models.V2beta1MetricSpecFluent Maven / Gradle / Ivy
/*
Copyright 2022 The Kubernetes Authors.
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package io.kubernetes.client.openapi.models;
import io.kubernetes.client.fluent.Fluent;
import io.kubernetes.client.fluent.Nested;
/** Generated */
public interface V2beta1MetricSpecFluent> extends Fluent {
/**
* This method has been deprecated, please use method buildContainerResource instead.
*
* @return The buildable object.
*/
@Deprecated
public V2beta1ContainerResourceMetricSource getContainerResource();
public io.kubernetes.client.openapi.models.V2beta1ContainerResourceMetricSource
buildContainerResource();
public A withContainerResource(
io.kubernetes.client.openapi.models.V2beta1ContainerResourceMetricSource containerResource);
public Boolean hasContainerResource();
public V2beta1MetricSpecFluent.ContainerResourceNested withNewContainerResource();
public io.kubernetes.client.openapi.models.V2beta1MetricSpecFluent.ContainerResourceNested
withNewContainerResourceLike(
io.kubernetes.client.openapi.models.V2beta1ContainerResourceMetricSource item);
public io.kubernetes.client.openapi.models.V2beta1MetricSpecFluent.ContainerResourceNested
editContainerResource();
public io.kubernetes.client.openapi.models.V2beta1MetricSpecFluent.ContainerResourceNested
editOrNewContainerResource();
public io.kubernetes.client.openapi.models.V2beta1MetricSpecFluent.ContainerResourceNested
editOrNewContainerResourceLike(
io.kubernetes.client.openapi.models.V2beta1ContainerResourceMetricSource item);
/**
* This method has been deprecated, please use method buildExternal instead.
*
* @return The buildable object.
*/
@java.lang.Deprecated
public V2beta1ExternalMetricSource getExternal();
public io.kubernetes.client.openapi.models.V2beta1ExternalMetricSource buildExternal();
public A withExternal(io.kubernetes.client.openapi.models.V2beta1ExternalMetricSource external);
public java.lang.Boolean hasExternal();
public V2beta1MetricSpecFluent.ExternalNested withNewExternal();
public io.kubernetes.client.openapi.models.V2beta1MetricSpecFluent.ExternalNested
withNewExternalLike(io.kubernetes.client.openapi.models.V2beta1ExternalMetricSource item);
public io.kubernetes.client.openapi.models.V2beta1MetricSpecFluent.ExternalNested
editExternal();
public io.kubernetes.client.openapi.models.V2beta1MetricSpecFluent.ExternalNested
editOrNewExternal();
public io.kubernetes.client.openapi.models.V2beta1MetricSpecFluent.ExternalNested
editOrNewExternalLike(io.kubernetes.client.openapi.models.V2beta1ExternalMetricSource item);
/**
* This method has been deprecated, please use method buildObject instead.
*
* @return The buildable object.
*/
@java.lang.Deprecated
public V2beta1ObjectMetricSource getObject();
public io.kubernetes.client.openapi.models.V2beta1ObjectMetricSource buildObject();
public A withObject(io.kubernetes.client.openapi.models.V2beta1ObjectMetricSource _object);
public java.lang.Boolean hasObject();
public V2beta1MetricSpecFluent.ObjectNested withNewObject();
public io.kubernetes.client.openapi.models.V2beta1MetricSpecFluent.ObjectNested
withNewObjectLike(io.kubernetes.client.openapi.models.V2beta1ObjectMetricSource item);
public io.kubernetes.client.openapi.models.V2beta1MetricSpecFluent.ObjectNested editObject();
public io.kubernetes.client.openapi.models.V2beta1MetricSpecFluent.ObjectNested
editOrNewObject();
public io.kubernetes.client.openapi.models.V2beta1MetricSpecFluent.ObjectNested
editOrNewObjectLike(io.kubernetes.client.openapi.models.V2beta1ObjectMetricSource item);
/**
* This method has been deprecated, please use method buildPods instead.
*
* @return The buildable object.
*/
@java.lang.Deprecated
public V2beta1PodsMetricSource getPods();
public io.kubernetes.client.openapi.models.V2beta1PodsMetricSource buildPods();
public A withPods(io.kubernetes.client.openapi.models.V2beta1PodsMetricSource pods);
public java.lang.Boolean hasPods();
public V2beta1MetricSpecFluent.PodsNested withNewPods();
public io.kubernetes.client.openapi.models.V2beta1MetricSpecFluent.PodsNested withNewPodsLike(
io.kubernetes.client.openapi.models.V2beta1PodsMetricSource item);
public io.kubernetes.client.openapi.models.V2beta1MetricSpecFluent.PodsNested editPods();
public io.kubernetes.client.openapi.models.V2beta1MetricSpecFluent.PodsNested editOrNewPods();
public io.kubernetes.client.openapi.models.V2beta1MetricSpecFluent.PodsNested
editOrNewPodsLike(io.kubernetes.client.openapi.models.V2beta1PodsMetricSource item);
/**
* This method has been deprecated, please use method buildResource instead.
*
* @return The buildable object.
*/
@java.lang.Deprecated
public V2beta1ResourceMetricSource getResource();
public io.kubernetes.client.openapi.models.V2beta1ResourceMetricSource buildResource();
public A withResource(io.kubernetes.client.openapi.models.V2beta1ResourceMetricSource resource);
public java.lang.Boolean hasResource();
public V2beta1MetricSpecFluent.ResourceNested withNewResource();
public io.kubernetes.client.openapi.models.V2beta1MetricSpecFluent.ResourceNested
withNewResourceLike(io.kubernetes.client.openapi.models.V2beta1ResourceMetricSource item);
public io.kubernetes.client.openapi.models.V2beta1MetricSpecFluent.ResourceNested
editResource();
public io.kubernetes.client.openapi.models.V2beta1MetricSpecFluent.ResourceNested
editOrNewResource();
public io.kubernetes.client.openapi.models.V2beta1MetricSpecFluent.ResourceNested
editOrNewResourceLike(io.kubernetes.client.openapi.models.V2beta1ResourceMetricSource item);
public String getType();
public A withType(java.lang.String type);
public java.lang.Boolean hasType();
public interface ContainerResourceNested
extends Nested,
V2beta1ContainerResourceMetricSourceFluent<
V2beta1MetricSpecFluent.ContainerResourceNested> {
public N and();
public N endContainerResource();
}
public interface ExternalNested
extends io.kubernetes.client.fluent.Nested,
V2beta1ExternalMetricSourceFluent> {
public N and();
public N endExternal();
}
public interface ObjectNested
extends io.kubernetes.client.fluent.Nested,
V2beta1ObjectMetricSourceFluent> {
public N and();
public N endObject();
}
public interface PodsNested
extends io.kubernetes.client.fluent.Nested,
V2beta1PodsMetricSourceFluent> {
public N and();
public N endPods();
}
public interface ResourceNested
extends io.kubernetes.client.fluent.Nested,
V2beta1ResourceMetricSourceFluent> {
public N and();
public N endResource();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy