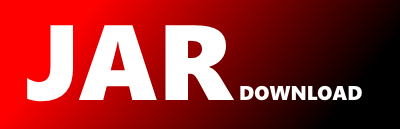
io.kubernetes.client.openapi.models.V1alpha2ResourceClaimStatusFluentImpl Maven / Gradle / Ivy
package io.kubernetes.client.openapi.models;
import io.kubernetes.client.fluent.VisitableBuilder;
import java.lang.SuppressWarnings;
import io.kubernetes.client.fluent.Nested;
import java.util.ArrayList;
import java.lang.String;
import java.util.function.Predicate;
import java.lang.Deprecated;
import io.kubernetes.client.fluent.BaseFluent;
import java.util.Iterator;
import java.util.List;
import java.lang.Boolean;
import java.util.Collection;
import java.lang.Object;
/**
* Generated
*/
@SuppressWarnings(value = "unchecked")
public class V1alpha2ResourceClaimStatusFluentImpl> extends BaseFluent implements V1alpha2ResourceClaimStatusFluent{
public V1alpha2ResourceClaimStatusFluentImpl() {
}
public V1alpha2ResourceClaimStatusFluentImpl(V1alpha2ResourceClaimStatus instance) {
if (instance != null) {
this.withAllocation(instance.getAllocation());
this.withDeallocationRequested(instance.getDeallocationRequested());
this.withDriverName(instance.getDriverName());
this.withReservedFor(instance.getReservedFor());
}
}
private V1alpha2AllocationResultBuilder allocation;
private Boolean deallocationRequested;
private String driverName;
private ArrayList reservedFor;
/**
* This method has been deprecated, please use method buildAllocation instead.
* @return The buildable object.
*/
@Deprecated
public V1alpha2AllocationResult getAllocation() {
return this.allocation!=null ?this.allocation.build():null;
}
public V1alpha2AllocationResult buildAllocation() {
return this.allocation!=null ?this.allocation.build():null;
}
public A withAllocation(V1alpha2AllocationResult allocation) {
_visitables.get("allocation").remove(this.allocation);
if (allocation!=null){ this.allocation= new V1alpha2AllocationResultBuilder(allocation); _visitables.get("allocation").add(this.allocation);} else { this.allocation = null; _visitables.get("allocation").remove(this.allocation); } return (A) this;
}
public Boolean hasAllocation() {
return this.allocation != null;
}
public V1alpha2ResourceClaimStatusFluentImpl.AllocationNested withNewAllocation() {
return new V1alpha2ResourceClaimStatusFluentImpl.AllocationNestedImpl();
}
public V1alpha2ResourceClaimStatusFluentImpl.AllocationNested withNewAllocationLike(V1alpha2AllocationResult item) {
return new V1alpha2ResourceClaimStatusFluentImpl.AllocationNestedImpl(item);
}
public V1alpha2ResourceClaimStatusFluentImpl.AllocationNested editAllocation() {
return withNewAllocationLike(getAllocation());
}
public V1alpha2ResourceClaimStatusFluentImpl.AllocationNested editOrNewAllocation() {
return withNewAllocationLike(getAllocation() != null ? getAllocation(): new V1alpha2AllocationResultBuilder().build());
}
public V1alpha2ResourceClaimStatusFluentImpl.AllocationNested editOrNewAllocationLike(V1alpha2AllocationResult item) {
return withNewAllocationLike(getAllocation() != null ? getAllocation(): item);
}
public Boolean getDeallocationRequested() {
return this.deallocationRequested;
}
public A withDeallocationRequested(Boolean deallocationRequested) {
this.deallocationRequested=deallocationRequested; return (A) this;
}
public Boolean hasDeallocationRequested() {
return this.deallocationRequested != null;
}
public String getDriverName() {
return this.driverName;
}
public A withDriverName(String driverName) {
this.driverName=driverName; return (A) this;
}
public Boolean hasDriverName() {
return this.driverName != null;
}
public A addToReservedFor(int index,V1alpha2ResourceClaimConsumerReference item) {
if (this.reservedFor == null) {this.reservedFor = new ArrayList();}
V1alpha2ResourceClaimConsumerReferenceBuilder builder = new V1alpha2ResourceClaimConsumerReferenceBuilder(item);
if (index < 0 || index >= reservedFor.size()) { _visitables.get("reservedFor").add(builder); reservedFor.add(builder); } else { _visitables.get("reservedFor").add(index, builder); reservedFor.add(index, builder);}
return (A)this;
}
public A setToReservedFor(int index,V1alpha2ResourceClaimConsumerReference item) {
if (this.reservedFor == null) {this.reservedFor = new ArrayList();}
V1alpha2ResourceClaimConsumerReferenceBuilder builder = new V1alpha2ResourceClaimConsumerReferenceBuilder(item);
if (index < 0 || index >= reservedFor.size()) { _visitables.get("reservedFor").add(builder); reservedFor.add(builder); } else { _visitables.get("reservedFor").set(index, builder); reservedFor.set(index, builder);}
return (A)this;
}
public A addToReservedFor(io.kubernetes.client.openapi.models.V1alpha2ResourceClaimConsumerReference... items) {
if (this.reservedFor == null) {this.reservedFor = new ArrayList();}
for (V1alpha2ResourceClaimConsumerReference item : items) {V1alpha2ResourceClaimConsumerReferenceBuilder builder = new V1alpha2ResourceClaimConsumerReferenceBuilder(item);_visitables.get("reservedFor").add(builder);this.reservedFor.add(builder);} return (A)this;
}
public A addAllToReservedFor(Collection items) {
if (this.reservedFor == null) {this.reservedFor = new ArrayList();}
for (V1alpha2ResourceClaimConsumerReference item : items) {V1alpha2ResourceClaimConsumerReferenceBuilder builder = new V1alpha2ResourceClaimConsumerReferenceBuilder(item);_visitables.get("reservedFor").add(builder);this.reservedFor.add(builder);} return (A)this;
}
public A removeFromReservedFor(io.kubernetes.client.openapi.models.V1alpha2ResourceClaimConsumerReference... items) {
for (V1alpha2ResourceClaimConsumerReference item : items) {V1alpha2ResourceClaimConsumerReferenceBuilder builder = new V1alpha2ResourceClaimConsumerReferenceBuilder(item);_visitables.get("reservedFor").remove(builder);if (this.reservedFor != null) {this.reservedFor.remove(builder);}} return (A)this;
}
public A removeAllFromReservedFor(Collection items) {
for (V1alpha2ResourceClaimConsumerReference item : items) {V1alpha2ResourceClaimConsumerReferenceBuilder builder = new V1alpha2ResourceClaimConsumerReferenceBuilder(item);_visitables.get("reservedFor").remove(builder);if (this.reservedFor != null) {this.reservedFor.remove(builder);}} return (A)this;
}
public A removeMatchingFromReservedFor(Predicate predicate) {
if (reservedFor == null) return (A) this;
final Iterator each = reservedFor.iterator();
final List visitables = _visitables.get("reservedFor");
while (each.hasNext()) {
V1alpha2ResourceClaimConsumerReferenceBuilder builder = each.next();
if (predicate.test(builder)) {
visitables.remove(builder);
each.remove();
}
}
return (A)this;
}
/**
* This method has been deprecated, please use method buildReservedFor instead.
* @return The buildable object.
*/
@Deprecated
public List getReservedFor() {
return reservedFor != null ? build(reservedFor) : null;
}
public List buildReservedFor() {
return reservedFor != null ? build(reservedFor) : null;
}
public V1alpha2ResourceClaimConsumerReference buildReservedFor(int index) {
return this.reservedFor.get(index).build();
}
public V1alpha2ResourceClaimConsumerReference buildFirstReservedFor() {
return this.reservedFor.get(0).build();
}
public V1alpha2ResourceClaimConsumerReference buildLastReservedFor() {
return this.reservedFor.get(reservedFor.size() - 1).build();
}
public V1alpha2ResourceClaimConsumerReference buildMatchingReservedFor(Predicate predicate) {
for (V1alpha2ResourceClaimConsumerReferenceBuilder item: reservedFor) { if(predicate.test(item)){ return item.build();} } return null;
}
public Boolean hasMatchingReservedFor(Predicate predicate) {
for (V1alpha2ResourceClaimConsumerReferenceBuilder item: reservedFor) { if(predicate.test(item)){ return true;} } return false;
}
public A withReservedFor(List reservedFor) {
if (this.reservedFor != null) { _visitables.get("reservedFor").clear();}
if (reservedFor != null) {this.reservedFor = new ArrayList(); for (V1alpha2ResourceClaimConsumerReference item : reservedFor){this.addToReservedFor(item);}} else { this.reservedFor = null;} return (A) this;
}
public A withReservedFor(io.kubernetes.client.openapi.models.V1alpha2ResourceClaimConsumerReference... reservedFor) {
if (this.reservedFor != null) {this.reservedFor.clear(); _visitables.remove("reservedFor"); }
if (reservedFor != null) {for (V1alpha2ResourceClaimConsumerReference item :reservedFor){ this.addToReservedFor(item);}} return (A) this;
}
public Boolean hasReservedFor() {
return reservedFor != null && !reservedFor.isEmpty();
}
public V1alpha2ResourceClaimStatusFluentImpl.ReservedForNested addNewReservedFor() {
return new V1alpha2ResourceClaimStatusFluentImpl.ReservedForNestedImpl();
}
public V1alpha2ResourceClaimStatusFluentImpl.ReservedForNested addNewReservedForLike(V1alpha2ResourceClaimConsumerReference item) {
return new V1alpha2ResourceClaimStatusFluentImpl.ReservedForNestedImpl(-1, item);
}
public V1alpha2ResourceClaimStatusFluentImpl.ReservedForNested setNewReservedForLike(int index,V1alpha2ResourceClaimConsumerReference item) {
return new V1alpha2ResourceClaimStatusFluentImpl.ReservedForNestedImpl(index, item);
}
public V1alpha2ResourceClaimStatusFluentImpl.ReservedForNested editReservedFor(int index) {
if (reservedFor.size() <= index) throw new RuntimeException("Can't edit reservedFor. Index exceeds size.");
return setNewReservedForLike(index, buildReservedFor(index));
}
public V1alpha2ResourceClaimStatusFluentImpl.ReservedForNested editFirstReservedFor() {
if (reservedFor.size() == 0) throw new RuntimeException("Can't edit first reservedFor. The list is empty.");
return setNewReservedForLike(0, buildReservedFor(0));
}
public V1alpha2ResourceClaimStatusFluentImpl.ReservedForNested editLastReservedFor() {
int index = reservedFor.size() - 1;
if (index < 0) throw new RuntimeException("Can't edit last reservedFor. The list is empty.");
return setNewReservedForLike(index, buildReservedFor(index));
}
public V1alpha2ResourceClaimStatusFluentImpl.ReservedForNested editMatchingReservedFor(Predicate predicate) {
int index = -1;
for (int i=0;i extends V1alpha2AllocationResultFluentImpl> implements V1alpha2ResourceClaimStatusFluentImpl.AllocationNested,Nested{
AllocationNestedImpl(V1alpha2AllocationResult item) {
this.builder = new V1alpha2AllocationResultBuilder(this, item);
}
AllocationNestedImpl() {
this.builder = new V1alpha2AllocationResultBuilder(this);
}
V1alpha2AllocationResultBuilder builder;
public N and() {
return (N) V1alpha2ResourceClaimStatusFluentImpl.this.withAllocation(builder.build());
}
public N endAllocation() {
return and();
}
}
class ReservedForNestedImpl extends V1alpha2ResourceClaimConsumerReferenceFluentImpl> implements V1alpha2ResourceClaimStatusFluentImpl.ReservedForNested,Nested{
ReservedForNestedImpl(int index,V1alpha2ResourceClaimConsumerReference item) {
this.index = index;
this.builder = new V1alpha2ResourceClaimConsumerReferenceBuilder(this, item);
}
ReservedForNestedImpl() {
this.index = -1;
this.builder = new V1alpha2ResourceClaimConsumerReferenceBuilder(this);
}
V1alpha2ResourceClaimConsumerReferenceBuilder builder;
int index;
public N and() {
return (N) V1alpha2ResourceClaimStatusFluentImpl.this.setToReservedFor(index,builder.build());
}
public N endReservedFor() {
return and();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy