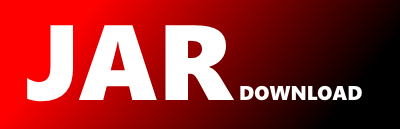
io.kubernetes.client.openapi.models.V1NodeStatusFluent Maven / Gradle / Ivy
package io.kubernetes.client.openapi.models;
import io.kubernetes.client.fluent.VisitableBuilder;
import java.util.ArrayList;
import java.lang.String;
import java.util.LinkedHashMap;
import java.util.function.Predicate;
import io.kubernetes.client.fluent.BaseFluent;
import java.util.List;
import java.util.Collection;
import java.lang.Object;
import java.util.Map;
import java.lang.SuppressWarnings;
import io.kubernetes.client.fluent.Nested;
import java.util.Iterator;
import io.kubernetes.client.custom.Quantity;
/**
* Generated
*/
@SuppressWarnings("unchecked")
public class V1NodeStatusFluent> extends BaseFluent{
public V1NodeStatusFluent() {
}
public V1NodeStatusFluent(V1NodeStatus instance) {
this.copyInstance(instance);
}
private ArrayList addresses;
private Map allocatable;
private Map capacity;
private ArrayList conditions;
private V1NodeConfigStatusBuilder config;
private V1NodeDaemonEndpointsBuilder daemonEndpoints;
private ArrayList images;
private V1NodeSystemInfoBuilder nodeInfo;
private String phase;
private ArrayList runtimeHandlers;
private ArrayList volumesAttached;
private List volumesInUse;
protected void copyInstance(V1NodeStatus instance) {
instance = (instance != null ? instance : new V1NodeStatus());
if (instance != null) {
this.withAddresses(instance.getAddresses());
this.withAllocatable(instance.getAllocatable());
this.withCapacity(instance.getCapacity());
this.withConditions(instance.getConditions());
this.withConfig(instance.getConfig());
this.withDaemonEndpoints(instance.getDaemonEndpoints());
this.withImages(instance.getImages());
this.withNodeInfo(instance.getNodeInfo());
this.withPhase(instance.getPhase());
this.withRuntimeHandlers(instance.getRuntimeHandlers());
this.withVolumesAttached(instance.getVolumesAttached());
this.withVolumesInUse(instance.getVolumesInUse());
}
}
public A addToAddresses(int index,V1NodeAddress item) {
if (this.addresses == null) {this.addresses = new ArrayList();}
V1NodeAddressBuilder builder = new V1NodeAddressBuilder(item);
if (index < 0 || index >= addresses.size()) { _visitables.get("addresses").add(builder); addresses.add(builder); } else { _visitables.get("addresses").add(index, builder); addresses.add(index, builder);}
return (A)this;
}
public A setToAddresses(int index,V1NodeAddress item) {
if (this.addresses == null) {this.addresses = new ArrayList();}
V1NodeAddressBuilder builder = new V1NodeAddressBuilder(item);
if (index < 0 || index >= addresses.size()) { _visitables.get("addresses").add(builder); addresses.add(builder); } else { _visitables.get("addresses").set(index, builder); addresses.set(index, builder);}
return (A)this;
}
public A addToAddresses(io.kubernetes.client.openapi.models.V1NodeAddress... items) {
if (this.addresses == null) {this.addresses = new ArrayList();}
for (V1NodeAddress item : items) {V1NodeAddressBuilder builder = new V1NodeAddressBuilder(item);_visitables.get("addresses").add(builder);this.addresses.add(builder);} return (A)this;
}
public A addAllToAddresses(Collection items) {
if (this.addresses == null) {this.addresses = new ArrayList();}
for (V1NodeAddress item : items) {V1NodeAddressBuilder builder = new V1NodeAddressBuilder(item);_visitables.get("addresses").add(builder);this.addresses.add(builder);} return (A)this;
}
public A removeFromAddresses(io.kubernetes.client.openapi.models.V1NodeAddress... items) {
if (this.addresses == null) return (A)this;
for (V1NodeAddress item : items) {V1NodeAddressBuilder builder = new V1NodeAddressBuilder(item);_visitables.get("addresses").remove(builder); this.addresses.remove(builder);} return (A)this;
}
public A removeAllFromAddresses(Collection items) {
if (this.addresses == null) return (A)this;
for (V1NodeAddress item : items) {V1NodeAddressBuilder builder = new V1NodeAddressBuilder(item);_visitables.get("addresses").remove(builder); this.addresses.remove(builder);} return (A)this;
}
public A removeMatchingFromAddresses(Predicate predicate) {
if (addresses == null) return (A) this;
final Iterator each = addresses.iterator();
final List visitables = _visitables.get("addresses");
while (each.hasNext()) {
V1NodeAddressBuilder builder = each.next();
if (predicate.test(builder)) {
visitables.remove(builder);
each.remove();
}
}
return (A)this;
}
public List buildAddresses() {
return this.addresses != null ? build(addresses) : null;
}
public V1NodeAddress buildAddress(int index) {
return this.addresses.get(index).build();
}
public V1NodeAddress buildFirstAddress() {
return this.addresses.get(0).build();
}
public V1NodeAddress buildLastAddress() {
return this.addresses.get(addresses.size() - 1).build();
}
public V1NodeAddress buildMatchingAddress(Predicate predicate) {
for (V1NodeAddressBuilder item : addresses) {
if (predicate.test(item)) {
return item.build();
}
}
return null;
}
public boolean hasMatchingAddress(Predicate predicate) {
for (V1NodeAddressBuilder item : addresses) {
if (predicate.test(item)) {
return true;
}
}
return false;
}
public A withAddresses(List addresses) {
if (this.addresses != null) {
this._visitables.get("addresses").clear();
}
if (addresses != null) {
this.addresses = new ArrayList();
for (V1NodeAddress item : addresses) {
this.addToAddresses(item);
}
} else {
this.addresses = null;
}
return (A) this;
}
public A withAddresses(io.kubernetes.client.openapi.models.V1NodeAddress... addresses) {
if (this.addresses != null) {
this.addresses.clear();
_visitables.remove("addresses");
}
if (addresses != null) {
for (V1NodeAddress item : addresses) {
this.addToAddresses(item);
}
}
return (A) this;
}
public boolean hasAddresses() {
return this.addresses != null && !this.addresses.isEmpty();
}
public AddressesNested addNewAddress() {
return new AddressesNested(-1, null);
}
public AddressesNested addNewAddressLike(V1NodeAddress item) {
return new AddressesNested(-1, item);
}
public AddressesNested setNewAddressLike(int index,V1NodeAddress item) {
return new AddressesNested(index, item);
}
public AddressesNested editAddress(int index) {
if (addresses.size() <= index) throw new RuntimeException("Can't edit addresses. Index exceeds size.");
return setNewAddressLike(index, buildAddress(index));
}
public AddressesNested editFirstAddress() {
if (addresses.size() == 0) throw new RuntimeException("Can't edit first addresses. The list is empty.");
return setNewAddressLike(0, buildAddress(0));
}
public AddressesNested editLastAddress() {
int index = addresses.size() - 1;
if (index < 0) throw new RuntimeException("Can't edit last addresses. The list is empty.");
return setNewAddressLike(index, buildAddress(index));
}
public AddressesNested editMatchingAddress(Predicate predicate) {
int index = -1;
for (int i=0;i map) {
if(this.allocatable == null && map != null) { this.allocatable = new LinkedHashMap(); }
if(map != null) { this.allocatable.putAll(map);} return (A)this;
}
public A removeFromAllocatable(String key) {
if(this.allocatable == null) { return (A) this; }
if(key != null && this.allocatable != null) {this.allocatable.remove(key);} return (A)this;
}
public A removeFromAllocatable(Map map) {
if(this.allocatable == null) { return (A) this; }
if(map != null) { for(Object key : map.keySet()) {if (this.allocatable != null){this.allocatable.remove(key);}}} return (A)this;
}
public Map getAllocatable() {
return this.allocatable;
}
public A withAllocatable(Map allocatable) {
if (allocatable == null) {
this.allocatable = null;
} else {
this.allocatable = new LinkedHashMap(allocatable);
}
return (A) this;
}
public boolean hasAllocatable() {
return this.allocatable != null;
}
public A addToCapacity(String key,Quantity value) {
if(this.capacity == null && key != null && value != null) { this.capacity = new LinkedHashMap(); }
if(key != null && value != null) {this.capacity.put(key, value);} return (A)this;
}
public A addToCapacity(Map map) {
if(this.capacity == null && map != null) { this.capacity = new LinkedHashMap(); }
if(map != null) { this.capacity.putAll(map);} return (A)this;
}
public A removeFromCapacity(String key) {
if(this.capacity == null) { return (A) this; }
if(key != null && this.capacity != null) {this.capacity.remove(key);} return (A)this;
}
public A removeFromCapacity(Map map) {
if(this.capacity == null) { return (A) this; }
if(map != null) { for(Object key : map.keySet()) {if (this.capacity != null){this.capacity.remove(key);}}} return (A)this;
}
public Map getCapacity() {
return this.capacity;
}
public A withCapacity(Map capacity) {
if (capacity == null) {
this.capacity = null;
} else {
this.capacity = new LinkedHashMap(capacity);
}
return (A) this;
}
public boolean hasCapacity() {
return this.capacity != null;
}
public A addToConditions(int index,V1NodeCondition item) {
if (this.conditions == null) {this.conditions = new ArrayList();}
V1NodeConditionBuilder builder = new V1NodeConditionBuilder(item);
if (index < 0 || index >= conditions.size()) { _visitables.get("conditions").add(builder); conditions.add(builder); } else { _visitables.get("conditions").add(index, builder); conditions.add(index, builder);}
return (A)this;
}
public A setToConditions(int index,V1NodeCondition item) {
if (this.conditions == null) {this.conditions = new ArrayList();}
V1NodeConditionBuilder builder = new V1NodeConditionBuilder(item);
if (index < 0 || index >= conditions.size()) { _visitables.get("conditions").add(builder); conditions.add(builder); } else { _visitables.get("conditions").set(index, builder); conditions.set(index, builder);}
return (A)this;
}
public A addToConditions(io.kubernetes.client.openapi.models.V1NodeCondition... items) {
if (this.conditions == null) {this.conditions = new ArrayList();}
for (V1NodeCondition item : items) {V1NodeConditionBuilder builder = new V1NodeConditionBuilder(item);_visitables.get("conditions").add(builder);this.conditions.add(builder);} return (A)this;
}
public A addAllToConditions(Collection items) {
if (this.conditions == null) {this.conditions = new ArrayList();}
for (V1NodeCondition item : items) {V1NodeConditionBuilder builder = new V1NodeConditionBuilder(item);_visitables.get("conditions").add(builder);this.conditions.add(builder);} return (A)this;
}
public A removeFromConditions(io.kubernetes.client.openapi.models.V1NodeCondition... items) {
if (this.conditions == null) return (A)this;
for (V1NodeCondition item : items) {V1NodeConditionBuilder builder = new V1NodeConditionBuilder(item);_visitables.get("conditions").remove(builder); this.conditions.remove(builder);} return (A)this;
}
public A removeAllFromConditions(Collection items) {
if (this.conditions == null) return (A)this;
for (V1NodeCondition item : items) {V1NodeConditionBuilder builder = new V1NodeConditionBuilder(item);_visitables.get("conditions").remove(builder); this.conditions.remove(builder);} return (A)this;
}
public A removeMatchingFromConditions(Predicate predicate) {
if (conditions == null) return (A) this;
final Iterator each = conditions.iterator();
final List visitables = _visitables.get("conditions");
while (each.hasNext()) {
V1NodeConditionBuilder builder = each.next();
if (predicate.test(builder)) {
visitables.remove(builder);
each.remove();
}
}
return (A)this;
}
public List buildConditions() {
return this.conditions != null ? build(conditions) : null;
}
public V1NodeCondition buildCondition(int index) {
return this.conditions.get(index).build();
}
public V1NodeCondition buildFirstCondition() {
return this.conditions.get(0).build();
}
public V1NodeCondition buildLastCondition() {
return this.conditions.get(conditions.size() - 1).build();
}
public V1NodeCondition buildMatchingCondition(Predicate predicate) {
for (V1NodeConditionBuilder item : conditions) {
if (predicate.test(item)) {
return item.build();
}
}
return null;
}
public boolean hasMatchingCondition(Predicate predicate) {
for (V1NodeConditionBuilder item : conditions) {
if (predicate.test(item)) {
return true;
}
}
return false;
}
public A withConditions(List conditions) {
if (this.conditions != null) {
this._visitables.get("conditions").clear();
}
if (conditions != null) {
this.conditions = new ArrayList();
for (V1NodeCondition item : conditions) {
this.addToConditions(item);
}
} else {
this.conditions = null;
}
return (A) this;
}
public A withConditions(io.kubernetes.client.openapi.models.V1NodeCondition... conditions) {
if (this.conditions != null) {
this.conditions.clear();
_visitables.remove("conditions");
}
if (conditions != null) {
for (V1NodeCondition item : conditions) {
this.addToConditions(item);
}
}
return (A) this;
}
public boolean hasConditions() {
return this.conditions != null && !this.conditions.isEmpty();
}
public ConditionsNested addNewCondition() {
return new ConditionsNested(-1, null);
}
public ConditionsNested addNewConditionLike(V1NodeCondition item) {
return new ConditionsNested(-1, item);
}
public ConditionsNested setNewConditionLike(int index,V1NodeCondition item) {
return new ConditionsNested(index, item);
}
public ConditionsNested editCondition(int index) {
if (conditions.size() <= index) throw new RuntimeException("Can't edit conditions. Index exceeds size.");
return setNewConditionLike(index, buildCondition(index));
}
public ConditionsNested editFirstCondition() {
if (conditions.size() == 0) throw new RuntimeException("Can't edit first conditions. The list is empty.");
return setNewConditionLike(0, buildCondition(0));
}
public ConditionsNested editLastCondition() {
int index = conditions.size() - 1;
if (index < 0) throw new RuntimeException("Can't edit last conditions. The list is empty.");
return setNewConditionLike(index, buildCondition(index));
}
public ConditionsNested editMatchingCondition(Predicate predicate) {
int index = -1;
for (int i=0;i withNewConfig() {
return new ConfigNested(null);
}
public ConfigNested withNewConfigLike(V1NodeConfigStatus item) {
return new ConfigNested(item);
}
public ConfigNested editConfig() {
return withNewConfigLike(java.util.Optional.ofNullable(buildConfig()).orElse(null));
}
public ConfigNested editOrNewConfig() {
return withNewConfigLike(java.util.Optional.ofNullable(buildConfig()).orElse(new V1NodeConfigStatusBuilder().build()));
}
public ConfigNested editOrNewConfigLike(V1NodeConfigStatus item) {
return withNewConfigLike(java.util.Optional.ofNullable(buildConfig()).orElse(item));
}
public V1NodeDaemonEndpoints buildDaemonEndpoints() {
return this.daemonEndpoints != null ? this.daemonEndpoints.build() : null;
}
public A withDaemonEndpoints(V1NodeDaemonEndpoints daemonEndpoints) {
this._visitables.remove("daemonEndpoints");
if (daemonEndpoints != null) {
this.daemonEndpoints = new V1NodeDaemonEndpointsBuilder(daemonEndpoints);
this._visitables.get("daemonEndpoints").add(this.daemonEndpoints);
} else {
this.daemonEndpoints = null;
this._visitables.get("daemonEndpoints").remove(this.daemonEndpoints);
}
return (A) this;
}
public boolean hasDaemonEndpoints() {
return this.daemonEndpoints != null;
}
public DaemonEndpointsNested withNewDaemonEndpoints() {
return new DaemonEndpointsNested(null);
}
public DaemonEndpointsNested withNewDaemonEndpointsLike(V1NodeDaemonEndpoints item) {
return new DaemonEndpointsNested(item);
}
public DaemonEndpointsNested editDaemonEndpoints() {
return withNewDaemonEndpointsLike(java.util.Optional.ofNullable(buildDaemonEndpoints()).orElse(null));
}
public DaemonEndpointsNested editOrNewDaemonEndpoints() {
return withNewDaemonEndpointsLike(java.util.Optional.ofNullable(buildDaemonEndpoints()).orElse(new V1NodeDaemonEndpointsBuilder().build()));
}
public DaemonEndpointsNested editOrNewDaemonEndpointsLike(V1NodeDaemonEndpoints item) {
return withNewDaemonEndpointsLike(java.util.Optional.ofNullable(buildDaemonEndpoints()).orElse(item));
}
public A addToImages(int index,V1ContainerImage item) {
if (this.images == null) {this.images = new ArrayList();}
V1ContainerImageBuilder builder = new V1ContainerImageBuilder(item);
if (index < 0 || index >= images.size()) { _visitables.get("images").add(builder); images.add(builder); } else { _visitables.get("images").add(index, builder); images.add(index, builder);}
return (A)this;
}
public A setToImages(int index,V1ContainerImage item) {
if (this.images == null) {this.images = new ArrayList();}
V1ContainerImageBuilder builder = new V1ContainerImageBuilder(item);
if (index < 0 || index >= images.size()) { _visitables.get("images").add(builder); images.add(builder); } else { _visitables.get("images").set(index, builder); images.set(index, builder);}
return (A)this;
}
public A addToImages(io.kubernetes.client.openapi.models.V1ContainerImage... items) {
if (this.images == null) {this.images = new ArrayList();}
for (V1ContainerImage item : items) {V1ContainerImageBuilder builder = new V1ContainerImageBuilder(item);_visitables.get("images").add(builder);this.images.add(builder);} return (A)this;
}
public A addAllToImages(Collection items) {
if (this.images == null) {this.images = new ArrayList();}
for (V1ContainerImage item : items) {V1ContainerImageBuilder builder = new V1ContainerImageBuilder(item);_visitables.get("images").add(builder);this.images.add(builder);} return (A)this;
}
public A removeFromImages(io.kubernetes.client.openapi.models.V1ContainerImage... items) {
if (this.images == null) return (A)this;
for (V1ContainerImage item : items) {V1ContainerImageBuilder builder = new V1ContainerImageBuilder(item);_visitables.get("images").remove(builder); this.images.remove(builder);} return (A)this;
}
public A removeAllFromImages(Collection items) {
if (this.images == null) return (A)this;
for (V1ContainerImage item : items) {V1ContainerImageBuilder builder = new V1ContainerImageBuilder(item);_visitables.get("images").remove(builder); this.images.remove(builder);} return (A)this;
}
public A removeMatchingFromImages(Predicate predicate) {
if (images == null) return (A) this;
final Iterator each = images.iterator();
final List visitables = _visitables.get("images");
while (each.hasNext()) {
V1ContainerImageBuilder builder = each.next();
if (predicate.test(builder)) {
visitables.remove(builder);
each.remove();
}
}
return (A)this;
}
public List buildImages() {
return this.images != null ? build(images) : null;
}
public V1ContainerImage buildImage(int index) {
return this.images.get(index).build();
}
public V1ContainerImage buildFirstImage() {
return this.images.get(0).build();
}
public V1ContainerImage buildLastImage() {
return this.images.get(images.size() - 1).build();
}
public V1ContainerImage buildMatchingImage(Predicate predicate) {
for (V1ContainerImageBuilder item : images) {
if (predicate.test(item)) {
return item.build();
}
}
return null;
}
public boolean hasMatchingImage(Predicate predicate) {
for (V1ContainerImageBuilder item : images) {
if (predicate.test(item)) {
return true;
}
}
return false;
}
public A withImages(List images) {
if (this.images != null) {
this._visitables.get("images").clear();
}
if (images != null) {
this.images = new ArrayList();
for (V1ContainerImage item : images) {
this.addToImages(item);
}
} else {
this.images = null;
}
return (A) this;
}
public A withImages(io.kubernetes.client.openapi.models.V1ContainerImage... images) {
if (this.images != null) {
this.images.clear();
_visitables.remove("images");
}
if (images != null) {
for (V1ContainerImage item : images) {
this.addToImages(item);
}
}
return (A) this;
}
public boolean hasImages() {
return this.images != null && !this.images.isEmpty();
}
public ImagesNested addNewImage() {
return new ImagesNested(-1, null);
}
public ImagesNested addNewImageLike(V1ContainerImage item) {
return new ImagesNested(-1, item);
}
public ImagesNested setNewImageLike(int index,V1ContainerImage item) {
return new ImagesNested(index, item);
}
public ImagesNested editImage(int index) {
if (images.size() <= index) throw new RuntimeException("Can't edit images. Index exceeds size.");
return setNewImageLike(index, buildImage(index));
}
public ImagesNested editFirstImage() {
if (images.size() == 0) throw new RuntimeException("Can't edit first images. The list is empty.");
return setNewImageLike(0, buildImage(0));
}
public ImagesNested editLastImage() {
int index = images.size() - 1;
if (index < 0) throw new RuntimeException("Can't edit last images. The list is empty.");
return setNewImageLike(index, buildImage(index));
}
public ImagesNested editMatchingImage(Predicate predicate) {
int index = -1;
for (int i=0;i withNewNodeInfo() {
return new NodeInfoNested(null);
}
public NodeInfoNested withNewNodeInfoLike(V1NodeSystemInfo item) {
return new NodeInfoNested(item);
}
public NodeInfoNested editNodeInfo() {
return withNewNodeInfoLike(java.util.Optional.ofNullable(buildNodeInfo()).orElse(null));
}
public NodeInfoNested editOrNewNodeInfo() {
return withNewNodeInfoLike(java.util.Optional.ofNullable(buildNodeInfo()).orElse(new V1NodeSystemInfoBuilder().build()));
}
public NodeInfoNested editOrNewNodeInfoLike(V1NodeSystemInfo item) {
return withNewNodeInfoLike(java.util.Optional.ofNullable(buildNodeInfo()).orElse(item));
}
public String getPhase() {
return this.phase;
}
public A withPhase(String phase) {
this.phase = phase;
return (A) this;
}
public boolean hasPhase() {
return this.phase != null;
}
public A addToRuntimeHandlers(int index,V1NodeRuntimeHandler item) {
if (this.runtimeHandlers == null) {this.runtimeHandlers = new ArrayList();}
V1NodeRuntimeHandlerBuilder builder = new V1NodeRuntimeHandlerBuilder(item);
if (index < 0 || index >= runtimeHandlers.size()) { _visitables.get("runtimeHandlers").add(builder); runtimeHandlers.add(builder); } else { _visitables.get("runtimeHandlers").add(index, builder); runtimeHandlers.add(index, builder);}
return (A)this;
}
public A setToRuntimeHandlers(int index,V1NodeRuntimeHandler item) {
if (this.runtimeHandlers == null) {this.runtimeHandlers = new ArrayList();}
V1NodeRuntimeHandlerBuilder builder = new V1NodeRuntimeHandlerBuilder(item);
if (index < 0 || index >= runtimeHandlers.size()) { _visitables.get("runtimeHandlers").add(builder); runtimeHandlers.add(builder); } else { _visitables.get("runtimeHandlers").set(index, builder); runtimeHandlers.set(index, builder);}
return (A)this;
}
public A addToRuntimeHandlers(io.kubernetes.client.openapi.models.V1NodeRuntimeHandler... items) {
if (this.runtimeHandlers == null) {this.runtimeHandlers = new ArrayList();}
for (V1NodeRuntimeHandler item : items) {V1NodeRuntimeHandlerBuilder builder = new V1NodeRuntimeHandlerBuilder(item);_visitables.get("runtimeHandlers").add(builder);this.runtimeHandlers.add(builder);} return (A)this;
}
public A addAllToRuntimeHandlers(Collection items) {
if (this.runtimeHandlers == null) {this.runtimeHandlers = new ArrayList();}
for (V1NodeRuntimeHandler item : items) {V1NodeRuntimeHandlerBuilder builder = new V1NodeRuntimeHandlerBuilder(item);_visitables.get("runtimeHandlers").add(builder);this.runtimeHandlers.add(builder);} return (A)this;
}
public A removeFromRuntimeHandlers(io.kubernetes.client.openapi.models.V1NodeRuntimeHandler... items) {
if (this.runtimeHandlers == null) return (A)this;
for (V1NodeRuntimeHandler item : items) {V1NodeRuntimeHandlerBuilder builder = new V1NodeRuntimeHandlerBuilder(item);_visitables.get("runtimeHandlers").remove(builder); this.runtimeHandlers.remove(builder);} return (A)this;
}
public A removeAllFromRuntimeHandlers(Collection items) {
if (this.runtimeHandlers == null) return (A)this;
for (V1NodeRuntimeHandler item : items) {V1NodeRuntimeHandlerBuilder builder = new V1NodeRuntimeHandlerBuilder(item);_visitables.get("runtimeHandlers").remove(builder); this.runtimeHandlers.remove(builder);} return (A)this;
}
public A removeMatchingFromRuntimeHandlers(Predicate predicate) {
if (runtimeHandlers == null) return (A) this;
final Iterator each = runtimeHandlers.iterator();
final List visitables = _visitables.get("runtimeHandlers");
while (each.hasNext()) {
V1NodeRuntimeHandlerBuilder builder = each.next();
if (predicate.test(builder)) {
visitables.remove(builder);
each.remove();
}
}
return (A)this;
}
public List buildRuntimeHandlers() {
return this.runtimeHandlers != null ? build(runtimeHandlers) : null;
}
public V1NodeRuntimeHandler buildRuntimeHandler(int index) {
return this.runtimeHandlers.get(index).build();
}
public V1NodeRuntimeHandler buildFirstRuntimeHandler() {
return this.runtimeHandlers.get(0).build();
}
public V1NodeRuntimeHandler buildLastRuntimeHandler() {
return this.runtimeHandlers.get(runtimeHandlers.size() - 1).build();
}
public V1NodeRuntimeHandler buildMatchingRuntimeHandler(Predicate predicate) {
for (V1NodeRuntimeHandlerBuilder item : runtimeHandlers) {
if (predicate.test(item)) {
return item.build();
}
}
return null;
}
public boolean hasMatchingRuntimeHandler(Predicate predicate) {
for (V1NodeRuntimeHandlerBuilder item : runtimeHandlers) {
if (predicate.test(item)) {
return true;
}
}
return false;
}
public A withRuntimeHandlers(List runtimeHandlers) {
if (this.runtimeHandlers != null) {
this._visitables.get("runtimeHandlers").clear();
}
if (runtimeHandlers != null) {
this.runtimeHandlers = new ArrayList();
for (V1NodeRuntimeHandler item : runtimeHandlers) {
this.addToRuntimeHandlers(item);
}
} else {
this.runtimeHandlers = null;
}
return (A) this;
}
public A withRuntimeHandlers(io.kubernetes.client.openapi.models.V1NodeRuntimeHandler... runtimeHandlers) {
if (this.runtimeHandlers != null) {
this.runtimeHandlers.clear();
_visitables.remove("runtimeHandlers");
}
if (runtimeHandlers != null) {
for (V1NodeRuntimeHandler item : runtimeHandlers) {
this.addToRuntimeHandlers(item);
}
}
return (A) this;
}
public boolean hasRuntimeHandlers() {
return this.runtimeHandlers != null && !this.runtimeHandlers.isEmpty();
}
public RuntimeHandlersNested addNewRuntimeHandler() {
return new RuntimeHandlersNested(-1, null);
}
public RuntimeHandlersNested addNewRuntimeHandlerLike(V1NodeRuntimeHandler item) {
return new RuntimeHandlersNested(-1, item);
}
public RuntimeHandlersNested setNewRuntimeHandlerLike(int index,V1NodeRuntimeHandler item) {
return new RuntimeHandlersNested(index, item);
}
public RuntimeHandlersNested editRuntimeHandler(int index) {
if (runtimeHandlers.size() <= index) throw new RuntimeException("Can't edit runtimeHandlers. Index exceeds size.");
return setNewRuntimeHandlerLike(index, buildRuntimeHandler(index));
}
public RuntimeHandlersNested editFirstRuntimeHandler() {
if (runtimeHandlers.size() == 0) throw new RuntimeException("Can't edit first runtimeHandlers. The list is empty.");
return setNewRuntimeHandlerLike(0, buildRuntimeHandler(0));
}
public RuntimeHandlersNested editLastRuntimeHandler() {
int index = runtimeHandlers.size() - 1;
if (index < 0) throw new RuntimeException("Can't edit last runtimeHandlers. The list is empty.");
return setNewRuntimeHandlerLike(index, buildRuntimeHandler(index));
}
public RuntimeHandlersNested editMatchingRuntimeHandler(Predicate predicate) {
int index = -1;
for (int i=0;i();}
V1AttachedVolumeBuilder builder = new V1AttachedVolumeBuilder(item);
if (index < 0 || index >= volumesAttached.size()) { _visitables.get("volumesAttached").add(builder); volumesAttached.add(builder); } else { _visitables.get("volumesAttached").add(index, builder); volumesAttached.add(index, builder);}
return (A)this;
}
public A setToVolumesAttached(int index,V1AttachedVolume item) {
if (this.volumesAttached == null) {this.volumesAttached = new ArrayList();}
V1AttachedVolumeBuilder builder = new V1AttachedVolumeBuilder(item);
if (index < 0 || index >= volumesAttached.size()) { _visitables.get("volumesAttached").add(builder); volumesAttached.add(builder); } else { _visitables.get("volumesAttached").set(index, builder); volumesAttached.set(index, builder);}
return (A)this;
}
public A addToVolumesAttached(io.kubernetes.client.openapi.models.V1AttachedVolume... items) {
if (this.volumesAttached == null) {this.volumesAttached = new ArrayList();}
for (V1AttachedVolume item : items) {V1AttachedVolumeBuilder builder = new V1AttachedVolumeBuilder(item);_visitables.get("volumesAttached").add(builder);this.volumesAttached.add(builder);} return (A)this;
}
public A addAllToVolumesAttached(Collection items) {
if (this.volumesAttached == null) {this.volumesAttached = new ArrayList();}
for (V1AttachedVolume item : items) {V1AttachedVolumeBuilder builder = new V1AttachedVolumeBuilder(item);_visitables.get("volumesAttached").add(builder);this.volumesAttached.add(builder);} return (A)this;
}
public A removeFromVolumesAttached(io.kubernetes.client.openapi.models.V1AttachedVolume... items) {
if (this.volumesAttached == null) return (A)this;
for (V1AttachedVolume item : items) {V1AttachedVolumeBuilder builder = new V1AttachedVolumeBuilder(item);_visitables.get("volumesAttached").remove(builder); this.volumesAttached.remove(builder);} return (A)this;
}
public A removeAllFromVolumesAttached(Collection items) {
if (this.volumesAttached == null) return (A)this;
for (V1AttachedVolume item : items) {V1AttachedVolumeBuilder builder = new V1AttachedVolumeBuilder(item);_visitables.get("volumesAttached").remove(builder); this.volumesAttached.remove(builder);} return (A)this;
}
public A removeMatchingFromVolumesAttached(Predicate predicate) {
if (volumesAttached == null) return (A) this;
final Iterator each = volumesAttached.iterator();
final List visitables = _visitables.get("volumesAttached");
while (each.hasNext()) {
V1AttachedVolumeBuilder builder = each.next();
if (predicate.test(builder)) {
visitables.remove(builder);
each.remove();
}
}
return (A)this;
}
public List buildVolumesAttached() {
return this.volumesAttached != null ? build(volumesAttached) : null;
}
public V1AttachedVolume buildVolumesAttached(int index) {
return this.volumesAttached.get(index).build();
}
public V1AttachedVolume buildFirstVolumesAttached() {
return this.volumesAttached.get(0).build();
}
public V1AttachedVolume buildLastVolumesAttached() {
return this.volumesAttached.get(volumesAttached.size() - 1).build();
}
public V1AttachedVolume buildMatchingVolumesAttached(Predicate predicate) {
for (V1AttachedVolumeBuilder item : volumesAttached) {
if (predicate.test(item)) {
return item.build();
}
}
return null;
}
public boolean hasMatchingVolumesAttached(Predicate predicate) {
for (V1AttachedVolumeBuilder item : volumesAttached) {
if (predicate.test(item)) {
return true;
}
}
return false;
}
public A withVolumesAttached(List volumesAttached) {
if (this.volumesAttached != null) {
this._visitables.get("volumesAttached").clear();
}
if (volumesAttached != null) {
this.volumesAttached = new ArrayList();
for (V1AttachedVolume item : volumesAttached) {
this.addToVolumesAttached(item);
}
} else {
this.volumesAttached = null;
}
return (A) this;
}
public A withVolumesAttached(io.kubernetes.client.openapi.models.V1AttachedVolume... volumesAttached) {
if (this.volumesAttached != null) {
this.volumesAttached.clear();
_visitables.remove("volumesAttached");
}
if (volumesAttached != null) {
for (V1AttachedVolume item : volumesAttached) {
this.addToVolumesAttached(item);
}
}
return (A) this;
}
public boolean hasVolumesAttached() {
return this.volumesAttached != null && !this.volumesAttached.isEmpty();
}
public VolumesAttachedNested addNewVolumesAttached() {
return new VolumesAttachedNested(-1, null);
}
public VolumesAttachedNested addNewVolumesAttachedLike(V1AttachedVolume item) {
return new VolumesAttachedNested(-1, item);
}
public VolumesAttachedNested setNewVolumesAttachedLike(int index,V1AttachedVolume item) {
return new VolumesAttachedNested(index, item);
}
public VolumesAttachedNested editVolumesAttached(int index) {
if (volumesAttached.size() <= index) throw new RuntimeException("Can't edit volumesAttached. Index exceeds size.");
return setNewVolumesAttachedLike(index, buildVolumesAttached(index));
}
public VolumesAttachedNested editFirstVolumesAttached() {
if (volumesAttached.size() == 0) throw new RuntimeException("Can't edit first volumesAttached. The list is empty.");
return setNewVolumesAttachedLike(0, buildVolumesAttached(0));
}
public VolumesAttachedNested editLastVolumesAttached() {
int index = volumesAttached.size() - 1;
if (index < 0) throw new RuntimeException("Can't edit last volumesAttached. The list is empty.");
return setNewVolumesAttachedLike(index, buildVolumesAttached(index));
}
public VolumesAttachedNested editMatchingVolumesAttached(Predicate predicate) {
int index = -1;
for (int i=0;i();}
this.volumesInUse.add(index, item);
return (A)this;
}
public A setToVolumesInUse(int index,String item) {
if (this.volumesInUse == null) {this.volumesInUse = new ArrayList();}
this.volumesInUse.set(index, item); return (A)this;
}
public A addToVolumesInUse(java.lang.String... items) {
if (this.volumesInUse == null) {this.volumesInUse = new ArrayList();}
for (String item : items) {this.volumesInUse.add(item);} return (A)this;
}
public A addAllToVolumesInUse(Collection items) {
if (this.volumesInUse == null) {this.volumesInUse = new ArrayList();}
for (String item : items) {this.volumesInUse.add(item);} return (A)this;
}
public A removeFromVolumesInUse(java.lang.String... items) {
if (this.volumesInUse == null) return (A)this;
for (String item : items) { this.volumesInUse.remove(item);} return (A)this;
}
public A removeAllFromVolumesInUse(Collection items) {
if (this.volumesInUse == null) return (A)this;
for (String item : items) { this.volumesInUse.remove(item);} return (A)this;
}
public List getVolumesInUse() {
return this.volumesInUse;
}
public String getVolumesInUse(int index) {
return this.volumesInUse.get(index);
}
public String getFirstVolumesInUse() {
return this.volumesInUse.get(0);
}
public String getLastVolumesInUse() {
return this.volumesInUse.get(volumesInUse.size() - 1);
}
public String getMatchingVolumesInUse(Predicate predicate) {
for (String item : volumesInUse) {
if (predicate.test(item)) {
return item;
}
}
return null;
}
public boolean hasMatchingVolumesInUse(Predicate predicate) {
for (String item : volumesInUse) {
if (predicate.test(item)) {
return true;
}
}
return false;
}
public A withVolumesInUse(List volumesInUse) {
if (volumesInUse != null) {
this.volumesInUse = new ArrayList();
for (String item : volumesInUse) {
this.addToVolumesInUse(item);
}
} else {
this.volumesInUse = null;
}
return (A) this;
}
public A withVolumesInUse(java.lang.String... volumesInUse) {
if (this.volumesInUse != null) {
this.volumesInUse.clear();
_visitables.remove("volumesInUse");
}
if (volumesInUse != null) {
for (String item : volumesInUse) {
this.addToVolumesInUse(item);
}
}
return (A) this;
}
public boolean hasVolumesInUse() {
return this.volumesInUse != null && !this.volumesInUse.isEmpty();
}
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
if (!super.equals(o)) return false;
V1NodeStatusFluent that = (V1NodeStatusFluent) o;
if (!java.util.Objects.equals(addresses, that.addresses)) return false;
if (!java.util.Objects.equals(allocatable, that.allocatable)) return false;
if (!java.util.Objects.equals(capacity, that.capacity)) return false;
if (!java.util.Objects.equals(conditions, that.conditions)) return false;
if (!java.util.Objects.equals(config, that.config)) return false;
if (!java.util.Objects.equals(daemonEndpoints, that.daemonEndpoints)) return false;
if (!java.util.Objects.equals(images, that.images)) return false;
if (!java.util.Objects.equals(nodeInfo, that.nodeInfo)) return false;
if (!java.util.Objects.equals(phase, that.phase)) return false;
if (!java.util.Objects.equals(runtimeHandlers, that.runtimeHandlers)) return false;
if (!java.util.Objects.equals(volumesAttached, that.volumesAttached)) return false;
if (!java.util.Objects.equals(volumesInUse, that.volumesInUse)) return false;
return true;
}
public int hashCode() {
return java.util.Objects.hash(addresses, allocatable, capacity, conditions, config, daemonEndpoints, images, nodeInfo, phase, runtimeHandlers, volumesAttached, volumesInUse, super.hashCode());
}
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (addresses != null && !addresses.isEmpty()) { sb.append("addresses:"); sb.append(addresses + ","); }
if (allocatable != null && !allocatable.isEmpty()) { sb.append("allocatable:"); sb.append(allocatable + ","); }
if (capacity != null && !capacity.isEmpty()) { sb.append("capacity:"); sb.append(capacity + ","); }
if (conditions != null && !conditions.isEmpty()) { sb.append("conditions:"); sb.append(conditions + ","); }
if (config != null) { sb.append("config:"); sb.append(config + ","); }
if (daemonEndpoints != null) { sb.append("daemonEndpoints:"); sb.append(daemonEndpoints + ","); }
if (images != null && !images.isEmpty()) { sb.append("images:"); sb.append(images + ","); }
if (nodeInfo != null) { sb.append("nodeInfo:"); sb.append(nodeInfo + ","); }
if (phase != null) { sb.append("phase:"); sb.append(phase + ","); }
if (runtimeHandlers != null && !runtimeHandlers.isEmpty()) { sb.append("runtimeHandlers:"); sb.append(runtimeHandlers + ","); }
if (volumesAttached != null && !volumesAttached.isEmpty()) { sb.append("volumesAttached:"); sb.append(volumesAttached + ","); }
if (volumesInUse != null && !volumesInUse.isEmpty()) { sb.append("volumesInUse:"); sb.append(volumesInUse); }
sb.append("}");
return sb.toString();
}
public class AddressesNested extends V1NodeAddressFluent> implements Nested{
AddressesNested(int index,V1NodeAddress item) {
this.index = index;
this.builder = new V1NodeAddressBuilder(this, item);
}
V1NodeAddressBuilder builder;
int index;
public N and() {
return (N) V1NodeStatusFluent.this.setToAddresses(index,builder.build());
}
public N endAddress() {
return and();
}
}
public class ConditionsNested extends V1NodeConditionFluent> implements Nested{
ConditionsNested(int index,V1NodeCondition item) {
this.index = index;
this.builder = new V1NodeConditionBuilder(this, item);
}
V1NodeConditionBuilder builder;
int index;
public N and() {
return (N) V1NodeStatusFluent.this.setToConditions(index,builder.build());
}
public N endCondition() {
return and();
}
}
public class ConfigNested extends V1NodeConfigStatusFluent> implements Nested{
ConfigNested(V1NodeConfigStatus item) {
this.builder = new V1NodeConfigStatusBuilder(this, item);
}
V1NodeConfigStatusBuilder builder;
public N and() {
return (N) V1NodeStatusFluent.this.withConfig(builder.build());
}
public N endConfig() {
return and();
}
}
public class DaemonEndpointsNested extends V1NodeDaemonEndpointsFluent> implements Nested{
DaemonEndpointsNested(V1NodeDaemonEndpoints item) {
this.builder = new V1NodeDaemonEndpointsBuilder(this, item);
}
V1NodeDaemonEndpointsBuilder builder;
public N and() {
return (N) V1NodeStatusFluent.this.withDaemonEndpoints(builder.build());
}
public N endDaemonEndpoints() {
return and();
}
}
public class ImagesNested extends V1ContainerImageFluent> implements Nested{
ImagesNested(int index,V1ContainerImage item) {
this.index = index;
this.builder = new V1ContainerImageBuilder(this, item);
}
V1ContainerImageBuilder builder;
int index;
public N and() {
return (N) V1NodeStatusFluent.this.setToImages(index,builder.build());
}
public N endImage() {
return and();
}
}
public class NodeInfoNested extends V1NodeSystemInfoFluent> implements Nested{
NodeInfoNested(V1NodeSystemInfo item) {
this.builder = new V1NodeSystemInfoBuilder(this, item);
}
V1NodeSystemInfoBuilder builder;
public N and() {
return (N) V1NodeStatusFluent.this.withNodeInfo(builder.build());
}
public N endNodeInfo() {
return and();
}
}
public class RuntimeHandlersNested extends V1NodeRuntimeHandlerFluent> implements Nested{
RuntimeHandlersNested(int index,V1NodeRuntimeHandler item) {
this.index = index;
this.builder = new V1NodeRuntimeHandlerBuilder(this, item);
}
V1NodeRuntimeHandlerBuilder builder;
int index;
public N and() {
return (N) V1NodeStatusFluent.this.setToRuntimeHandlers(index,builder.build());
}
public N endRuntimeHandler() {
return and();
}
}
public class VolumesAttachedNested extends V1AttachedVolumeFluent> implements Nested{
VolumesAttachedNested(int index,V1AttachedVolume item) {
this.index = index;
this.builder = new V1AttachedVolumeBuilder(this, item);
}
V1AttachedVolumeBuilder builder;
int index;
public N and() {
return (N) V1NodeStatusFluent.this.setToVolumesAttached(index,builder.build());
}
public N endVolumesAttached() {
return and();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy