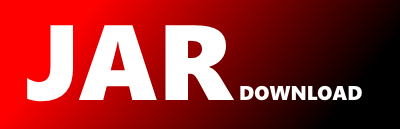
io.kubernetes.client.extended.controller.builder.ControllerWatchBuilder Maven / Gradle / Ivy
/*
Copyright 2020 The Kubernetes Authors.
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package io.kubernetes.client.extended.controller.builder;
import io.kubernetes.client.common.KubernetesObject;
import io.kubernetes.client.extended.controller.Controllers;
import io.kubernetes.client.extended.controller.DefaultControllerWatch;
import io.kubernetes.client.extended.controller.reconciler.Request;
import io.kubernetes.client.extended.workqueue.WorkQueue;
import java.time.Duration;
import java.util.function.BiPredicate;
import java.util.function.Function;
import java.util.function.Predicate;
public class ControllerWatchBuilder {
private Function workKeyGenerator;
private WorkQueue workQueue;
private Class apiTypeClass;
private Duration resyncPeriod = Duration.ZERO;
private Predicate onAddFilterPredicate;
private BiPredicate onUpdateFilterPredicate;
private BiPredicate onDeleteFilterPredicate;
ControllerWatchBuilder(Class apiTypeClass, WorkQueue workQueue) {
this.apiTypeClass = apiTypeClass;
this.workKeyGenerator = Controllers.defaultReflectiveKeyFunc();
this.workQueue = workQueue;
}
/**
* Sets a filter for add notification.
*
* @param filter the filter
* @return the controller builder . controller watch builder
*/
public ControllerWatchBuilder withOnAddFilter(Predicate filter) {
this.onAddFilterPredicate = filter;
return this;
}
/**
* Sets a filter for update notification.
*
* @param filter the filter
* @return the controller builder . controller watch builder
*/
public ControllerWatchBuilder withOnUpdateFilter(BiPredicate filter) {
this.onUpdateFilterPredicate = filter;
return this;
}
/**
* Sets a filter for delete notification.
*
* @param filter the filter
* @return the controller builder . controller watch builder
*/
public ControllerWatchBuilder withOnDeleteFilter(BiPredicate filter) {
this.onDeleteFilterPredicate = filter;
return this;
}
/**
* Overrides work-queue key-func for the watch.
*
* @param workKeyGenerator the work key generator
* @return the controller builder . controller watch builder
*/
public ControllerWatchBuilder withWorkQueueKeyFunc(
Function workKeyGenerator) {
this.workKeyGenerator = workKeyGenerator;
return this;
}
public ControllerWatchBuilder withResyncPeriod(Duration resyncPeriod) {
this.resyncPeriod = resyncPeriod;
return this;
}
/**
* End building controller-watch.
*
* @return the controller builder
* @throws IllegalStateException the illegal state exception
*/
public DefaultControllerWatch build() throws IllegalStateException {
DefaultControllerWatch workQueueHandler =
new DefaultControllerWatch<>(apiTypeClass, workQueue, workKeyGenerator, resyncPeriod);
workQueueHandler.setOnAddFilterPredicate(onAddFilterPredicate);
workQueueHandler.setOnUpdateFilterPredicate(onUpdateFilterPredicate);
workQueueHandler.setOnDeleteFilterPredicate(onDeleteFilterPredicate);
return workQueueHandler;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy