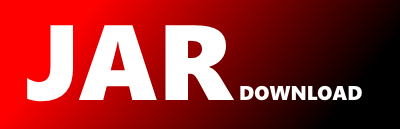
io.kubernetes.client.extended.kubectl.KubectlGet Maven / Gradle / Ivy
The newest version!
/*
Copyright 2020 The Kubernetes Authors.
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package io.kubernetes.client.extended.kubectl;
import io.kubernetes.client.common.KubernetesListObject;
import io.kubernetes.client.common.KubernetesObject;
import io.kubernetes.client.extended.kubectl.exception.KubectlException;
import io.kubernetes.client.openapi.ApiException;
import io.kubernetes.client.util.generic.GenericKubernetesApi;
import io.kubernetes.client.util.generic.options.ListOptions;
import java.util.List;
import org.apache.commons.lang3.StringUtils;
public class KubectlGet
extends Kubectl.ApiClientBuilder>
implements Kubectl.Executable> {
private String namespace;
private ListOptions listOptions;
private Class apiTypeClass;
private Class extends KubernetesListObject> apiTypeListClass;
KubectlGet(Class apiTypeClass) {
this.apiTypeClass = apiTypeClass;
this.listOptions = new ListOptions();
}
public KubectlGet apiListTypeClass(
Class extends KubernetesListObject> apiTypeListClass) {
this.apiTypeListClass = apiTypeListClass;
return this;
}
public KubectlGet options(ListOptions listOptions) {
this.listOptions = listOptions;
return this;
}
public KubectlGet namespace(String namespace) {
this.namespace = namespace;
return this;
}
public KubectlGetSingle name(String name) {
return new KubectlGetSingle(name);
}
@Override
public List execute() throws KubectlException {
refreshDiscovery();
GenericKubernetesApi api =
apiTypeListClass == null
? getGenericApi(apiTypeClass)
: getGenericApi(apiTypeClass, apiTypeListClass);
try {
if (isNamespaced()) {
return (List)
api.list(namespace, listOptions).throwsApiException().getObject().getItems();
} else {
return (List) api.list(listOptions).throwsApiException().getObject().getItems();
}
} catch (ApiException e) {
throw new KubectlException(e);
}
}
private boolean isNamespaced() {
return !StringUtils.isEmpty(namespace);
}
public class KubectlGetSingle extends Kubectl.ResourceBuilder
implements Kubectl.Executable {
private KubectlGetSingle(String name) {
super(KubectlGet.this.apiTypeClass);
KubectlGetSingle.this.name = name;
KubectlGetSingle.this.namespace = KubectlGet.this.namespace;
KubectlGetSingle.this.apiClient = KubectlGet.this.apiClient;
KubectlGetSingle.this.skipDiscovery = KubectlGet.this.skipDiscovery;
}
private boolean isNamespaced() {
return !StringUtils.isEmpty(namespace);
}
@Override
public ApiType execute() throws KubectlException {
refreshDiscovery();
GenericKubernetesApi api =
getGenericApi(KubectlGetSingle.this.apiTypeClass);
try {
if (isNamespaced()) {
return api.get(KubectlGetSingle.this.namespace, KubectlGetSingle.this.name)
.throwsApiException()
.getObject();
} else {
return api.get(KubectlGetSingle.this.name).throwsApiException().getObject();
}
} catch (ApiException e) {
throw new KubectlException(e);
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy