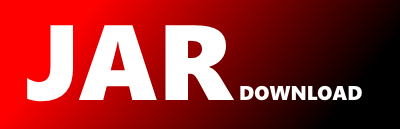
io.lakefs.clients.sdk.HealthCheckApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sdk Show documentation
Show all versions of sdk Show documentation
lakeFS OpenAPI Java client
/*
* lakeFS API
* lakeFS HTTP API
*
* The version of the OpenAPI document: 1.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package io.lakefs.clients.sdk;
import io.lakefs.clients.sdk.ApiCallback;
import io.lakefs.clients.sdk.ApiClient;
import io.lakefs.clients.sdk.ApiException;
import io.lakefs.clients.sdk.ApiResponse;
import io.lakefs.clients.sdk.Configuration;
import io.lakefs.clients.sdk.Pair;
import io.lakefs.clients.sdk.ProgressRequestBody;
import io.lakefs.clients.sdk.ProgressResponseBody;
import com.google.gson.reflect.TypeToken;
import java.io.IOException;
import java.lang.reflect.Type;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class HealthCheckApi {
private ApiClient localVarApiClient;
private int localHostIndex;
private String localCustomBaseUrl;
public HealthCheckApi() {
this(Configuration.getDefaultApiClient());
}
public HealthCheckApi(ApiClient apiClient) {
this.localVarApiClient = apiClient;
}
public ApiClient getApiClient() {
return localVarApiClient;
}
public void setApiClient(ApiClient apiClient) {
this.localVarApiClient = apiClient;
}
public int getHostIndex() {
return localHostIndex;
}
public void setHostIndex(int hostIndex) {
this.localHostIndex = hostIndex;
}
public String getCustomBaseUrl() {
return localCustomBaseUrl;
}
public void setCustomBaseUrl(String customBaseUrl) {
this.localCustomBaseUrl = customBaseUrl;
}
private okhttp3.Call healthCheckCall(final ApiCallback _callback) throws ApiException {
String basePath = null;
// Operation Servers
String[] localBasePaths = new String[] { };
// Determine Base Path to Use
if (localCustomBaseUrl != null){
basePath = localCustomBaseUrl;
} else if ( localBasePaths.length > 0 ) {
basePath = localBasePaths[localHostIndex];
} else {
basePath = null;
}
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/healthcheck";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
};
final String localVarAccept = localVarApiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {
};
final String localVarContentType = localVarApiClient.selectHeaderContentType(localVarContentTypes);
if (localVarContentType != null) {
localVarHeaderParams.put("Content-Type", localVarContentType);
}
String[] localVarAuthNames = new String[] { };
return localVarApiClient.buildCall(basePath, localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarCookieParams, localVarFormParams, localVarAuthNames, _callback);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call healthCheckValidateBeforeCall(final ApiCallback _callback) throws ApiException {
return healthCheckCall(_callback);
}
private ApiResponse healthCheckWithHttpInfo() throws ApiException {
okhttp3.Call localVarCall = healthCheckValidateBeforeCall(null);
return localVarApiClient.execute(localVarCall);
}
private okhttp3.Call healthCheckAsync(final ApiCallback _callback) throws ApiException {
okhttp3.Call localVarCall = healthCheckValidateBeforeCall(_callback);
localVarApiClient.executeAsync(localVarCall, _callback);
return localVarCall;
}
public class APIhealthCheckRequest {
private APIhealthCheckRequest() {
}
/**
* Build call for healthCheck
* @param _callback ApiCallback API callback
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
* @http.response.details
Status Code Description Response Headers
204 NoContent -
*/
public okhttp3.Call buildCall(final ApiCallback _callback) throws ApiException {
return healthCheckCall(_callback);
}
/**
* Execute healthCheck request
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
* @http.response.details
Status Code Description Response Headers
204 NoContent -
*/
public void execute() throws ApiException {
healthCheckWithHttpInfo();
}
/**
* Execute healthCheck request with HTTP info returned
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
* @http.response.details
Status Code Description Response Headers
204 NoContent -
*/
public ApiResponse executeWithHttpInfo() throws ApiException {
return healthCheckWithHttpInfo();
}
/**
* Execute healthCheck request (asynchronously)
* @param _callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
* @http.response.details
Status Code Description Response Headers
204 NoContent -
*/
public okhttp3.Call executeAsync(final ApiCallback _callback) throws ApiException {
return healthCheckAsync(_callback);
}
}
/**
*
* check that the API server is up and running
* @return APIhealthCheckRequest
* @http.response.details
Status Code Description Response Headers
204 NoContent -
*/
public APIhealthCheckRequest healthCheck() {
return new APIhealthCheckRequest();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy