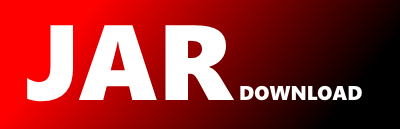
io.legaldocml.business.builder.MetaBuilder Maven / Gradle / Ivy
package io.legaldocml.business.builder;
import io.legaldocml.akn.AknElements;
import io.legaldocml.akn.element.CoreProperties;
import io.legaldocml.akn.element.FRBRauthor;
import io.legaldocml.akn.element.FRBRdate;
import io.legaldocml.akn.element.Identification;
import io.legaldocml.akn.type.AgentRef;
import io.legaldocml.akn.util.AknList;
import io.legaldocml.akn.util.FRBRHelper;
import io.legaldocml.business.AknIdentifier;
import io.legaldocml.model.Country;
import io.legaldocml.model.Language;
import io.legaldocml.util.DateHelper;
import io.legaldocml.util.Uri;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.time.LocalDate;
import java.util.function.Function;
import static io.legaldocml.akn.util.FRBRHelper.newFRBRlanguage;
/**
* @author Jacques Militello
*/
public class MetaBuilder {
public static final Function FRBR_WORK = new CorePropertiesGetter(AknElements.FRBR_WORK) {
/**
* {@inheritDoc}
*/
@Override
public CoreProperties apply(Identification identification) {
return identification.getFRBRWork();
}
};
public static final Function FRBR_EXPRESSION = new CorePropertiesGetter(AknElements.FRBR_EXPRESSION) {
/**
* {@inheritDoc}
*/
@Override
public CoreProperties apply(Identification identification) {
return identification.getFRBRExpression();
}
};
public static final Function FRBR_MANIFESTATION = new CorePropertiesGetter(AknElements.FRBR_MANIFESTATION) {
/**
* {@inheritDoc}
*/
@Override
public CoreProperties apply(Identification identification) {
return identification.getFRBRManifestation();
}
};
/**
* SLF4J Logger.
*/
private static final Logger LOGGER = LoggerFactory.getLogger(MetaBuilder.class);
private final BusinessBuilder businessBuilder;
private final Identification identification;
protected MetaBuilder(BusinessBuilder businessBuilder, AgentRef source) {
this.businessBuilder = businessBuilder;
this.identification = businessBuilder.getAkomaNtoso().getDocumentType().getMeta().getIdentification();
this.identification.setSource(source);
}
public void setAknIdentifier(AknIdentifier identifier) {
if (LOGGER.isDebugEnabled()) {
LOGGER.debug("setAknIdentifier({}]", identifier);
}
if (identifier == null) {
throw new BusinessBuilderException("argument identifier is null");
}
identifier.apply(this.businessBuilder.getAkomaNtoso());
}
public void addLanguage(Language language) {
this.addLanguage(language, Language::getCode);
}
public void addLanguage(Language language, Function mapper) {
if (LOGGER.isDebugEnabled()) {
LOGGER.debug("addLanguage({}) -> {}", language, mapper.apply(language));
}
this.identification.getFRBRExpression().add(newFRBRlanguage(mapper.apply(language)));
}
public void setDate(LocalDate date, String name) {
if (LOGGER.isDebugEnabled()) {
LOGGER.debug("setDate({})", date);
}
setDate(date, name, FRBR_WORK);
setDate(date, name, FRBR_EXPRESSION);
setDate(date, name, FRBR_MANIFESTATION);
}
public void setDate(LocalDate date, String name, Function map) {
if (LOGGER.isDebugEnabled()) {
LOGGER.debug("setDate({})", date);
}
FRBRdate frbr = map.apply(this.identification).getFRBRdate();
frbr.setDate(DateHelper.convert(date));
frbr.setName(name);
}
public void setCountry(Country country) {
this.setCountry(country, Country::getAlpha2);
}
public void setCountry(Country country, Function mapper) {
if (LOGGER.isDebugEnabled()) {
LOGGER.debug("setCountry({})", country);
}
this.identification.getFRBRWork().getFRBRcountry().setValue(mapper.apply(country));
}
public void addAuthor(Uri href) {
addAuthor(href, FRBR_WORK);
addAuthor(href, FRBR_EXPRESSION);
addAuthor(href, FRBR_MANIFESTATION);
}
public void addAuthor(Uri href, Function type) {
if (LOGGER.isDebugEnabled()) {
LOGGER.debug("addAuthor({}) for ({})", href, type);
}
FRBRauthor frbRauthor = FRBRHelper.newFRBRauthor(href);
AknList authors = type.apply(this.identification).getAuthors();
if (!authors.contains(frbRauthor)) {
authors.add(frbRauthor);
} else {
if (LOGGER.isWarnEnabled()) {
LOGGER.warn("author [{}] already exists in [{}]", frbRauthor, type);
}
}
}
private abstract static class CorePropertiesGetter implements Function {
private final String name;
private CorePropertiesGetter(String name) {
this.name = name;
}
@Override
public String toString() {
return this.name;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy