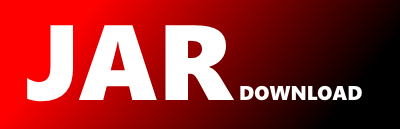
io.lenses.jdbc4.statements.statements.kt Maven / Gradle / Ivy
package io.lenses.jdbc4.statements
import io.lenses.jdbc4.IWrapper
import io.lenses.jdbc4.util.Logging
import java.io.InputStream
import java.io.Reader
import java.math.BigDecimal
import java.net.URL
import java.sql.Blob
import java.sql.Clob
import java.sql.Date
import java.sql.NClob
import java.sql.PreparedStatement
import java.sql.Ref
import java.sql.ResultSet
import java.sql.RowId
import java.sql.SQLFeatureNotSupportedException
import java.sql.SQLWarning
import java.sql.SQLXML
import java.sql.Statement
import java.sql.Time
import java.sql.Timestamp
import java.util.*
typealias Unsupported = SQLFeatureNotSupportedException
fun unsupported(): Nothing = throw SQLFeatureNotSupportedException()
/**
* Overrides functions in [Statement] that close a stream.
* With HTTP implementations there is nothing to close, so these are noops.
*/
interface OfflineStatement : Statement, Logging {
// -- Lenses statements are offline, and so there's nothing to close
override fun isCloseOnCompletion(): Boolean = true
override fun close() {} // lsql-statements have no resources associated
override fun isClosed(): Boolean = true
override fun closeOnCompletion() {}
override fun cancel() {}
}
interface IWrapperStatement : Statement, IWrapper {
override fun isWrapperFor(iface: Class<*>?): Boolean = _isWrapperFor(iface)
override fun unwrap(iface: Class): T = _unwrap(iface)
}
/**
* Sets up some default values for all Lenses [Statement] instances.
*/
interface DefaultStatement : Statement {
override fun clearWarnings() {}
override fun getWarnings(): SQLWarning = SQLWarning()
override fun getMoreResults(): Boolean = false
override fun getMoreResults(current: Int): Boolean = false
// max bytes in a column, irrelevant for lenses sql
override fun getMaxFieldSize(): Int = -1
override fun setMaxFieldSize(max: Int) {}
// equivalent to a limit
override fun getMaxRows(): Int = -1
override fun setMaxRows(max: Int) {}
override fun getFetchSize(): Int = -1
override fun setFetchSize(rows: Int) {}
override fun getFetchDirection(): Int = ResultSet.FETCH_FORWARD
override fun setFetchDirection(direction: Int) {
if (direction != ResultSet.FETCH_FORWARD)
throw SQLFeatureNotSupportedException("Lenses ResultSets can only be ResultSet.FETCH_FORWARD")
}
override fun setPoolable(poolable: Boolean) {
}
override fun getResultSetHoldability(): Int = ResultSet.CLOSE_CURSORS_AT_COMMIT
override fun setCursorName(name: String?) = throw SQLFeatureNotSupportedException()
override fun setEscapeProcessing(enable: Boolean) {}
override fun isPoolable(): Boolean = false
override fun getResultSetType(): Int = ResultSet.TYPE_FORWARD_ONLY
override fun getResultSetConcurrency(): Int = ResultSet.CONCUR_READ_ONLY
// == auto generated keys are not supported by kafka/lenses ==
override fun getGeneratedKeys(): ResultSet = throw SQLFeatureNotSupportedException("Auto generated keys are not supported by Lenses")
}
/**
* Overrides functions in [Statement] that cannot be executed on a [PreparedStatement].
*/
interface AbstractPreparedStatement : PreparedStatement,
UnsupportedTypesPreparedStatement,
IWrapperStatement,
DefaultStatement,
OfflineStatement,
Logging {
private fun unsupported(): Nothing = throw Unsupported("This method cannot be called on a prepared statement")
override fun addBatch(sql: String?) = unsupported()
// -- execute methods that accept SQL are not used by prepared statements
override fun executeQuery(sql: String): ResultSet = unsupported()
override fun execute(sql: String): Boolean = unsupported()
override fun execute(sql: String?, autoGeneratedKeys: Int): Boolean = unsupported()
override fun execute(sql: String?, columnNames: Array?): Boolean = unsupported()
override fun execute(sql: String?, columnIndexes: IntArray?): Boolean = unsupported()
override fun executeUpdate(sql: String?): Int = unsupported()
override fun executeUpdate(sql: String?, autoGeneratedKeys: Int): Int = unsupported()
override fun executeUpdate(sql: String?, columnIndexes: IntArray?): Int = unsupported()
override fun executeUpdate(sql: String?, columnNames: Array?): Int = unsupported()
}
interface ReadOnlyPreparedStatement : PreparedStatement {
override fun addBatch() = unsupported()
override fun clearBatch() = unsupported()
override fun executeBatch(): IntArray = unsupported()
override fun getUpdateCount(): Int = -1
}
/**
* Overrides functions in [Statement] that operate on types not supported by Lenses SQL, such as [Clob].
*/
interface UnsupportedTypesPreparedStatement : PreparedStatement {
// -- methods which set values on the current record
override fun setCharacterStream(parameterIndex: Int, reader: Reader?, length: Int) = unsupported()
override fun setCharacterStream(parameterIndex: Int, reader: Reader?, length: Long) = unsupported()
override fun setCharacterStream(parameterIndex: Int, reader: Reader?) = unsupported()
override fun setDate(parameterIndex: Int, d: Date?) = unsupported()
override fun setDate(parameterIndex: Int, d: Date?, cal: Calendar?) = unsupported()
override fun setObject(parameterIndex: Int, x: Any?) = unsupported()
override fun setLong(parameterIndex: Int, x: Long) = unsupported()
override fun setNString(parameterIndex: Int, x: String?) = unsupported()
override fun setURL(parameterIndex: Int, u: URL?) = unsupported()
override fun setFloat(parameterIndex: Int, f: Float) = unsupported()
override fun setTime(parameterIndex: Int, t: Time?) = unsupported()
override fun setTime(parameterIndex: Int, x: Time?, cal: Calendar?) = unsupported()
override fun setNCharacterStream(parameterIndex: Int, value: Reader?, length: Long) = unsupported()
override fun setNCharacterStream(parameterIndex: Int, value: Reader?) = unsupported()
override fun setInt(parameterIndex: Int, x: Int) = unsupported()
override fun setDouble(parameterIndex: Int, x: Double) = unsupported()
override fun setBigDecimal(parameterIndex: Int, x: BigDecimal?) = unsupported()
override fun setObject(parameterIndex: Int, x: Any?, targetSqlType: Int) = unsupported()
override fun setString(parameterIndex: Int, x: String?) = unsupported()
override fun setNull(parameterIndex: Int, sqlType: Int) = unsupported()
override fun setNull(parameterIndex: Int, sqlType: Int, typeName: String?) = unsupported()
override fun setTimestamp(parameterIndex: Int, ts: Timestamp?) = unsupported()
override fun setTimestamp(parameterIndex: Int, ts: Timestamp?, cal: Calendar?) = unsupported()
override fun setShort(parameterIndex: Int, s: Short) = unsupported()
override fun setBoolean(parameterIndex: Int, b: Boolean) = unsupported()
override fun setByte(parameterIndex: Int, b: Byte) = unsupported()
// -- unsupported types --
override fun setBinaryStream(parameterIndex: Int, x: InputStream?, length: Int) = unsupported()
override fun setBinaryStream(parameterIndex: Int, x: InputStream?, length: Long) = unsupported()
override fun setBinaryStream(parameterIndex: Int, x: InputStream?) = unsupported()
override fun setClob(parameterIndex: Int, x: Clob?) = unsupported()
override fun setClob(parameterIndex: Int, reader: Reader?, length: Long) = unsupported()
override fun setClob(parameterIndex: Int, reader: Reader?) = unsupported()
override fun setUnicodeStream(parameterIndex: Int, x: InputStream?, length: Int) = unsupported()
override fun setObject(parameterIndex: Int, x: Any?, targetSqlType: Int, scaleOrLength: Int) = unsupported()
override fun setBytes(parameterIndex: Int, x: ByteArray?) = unsupported()
override fun setSQLXML(parameterIndex: Int, xmlObject: SQLXML?) = unsupported()
override fun setRef(parameterIndex: Int, x: Ref?) = unsupported()
override fun setBlob(parameterIndex: Int, x: Blob?) = unsupported()
override fun setBlob(parameterIndex: Int, inputStream: InputStream?, length: Long) = unsupported()
override fun setBlob(parameterIndex: Int, inputStream: InputStream?) = unsupported()
override fun setArray(parameterIndex: Int, x: java.sql.Array?) = unsupported()
override fun setRowId(parameterIndex: Int, x: RowId?) = unsupported()
override fun setAsciiStream(parameterIndex: Int, x: InputStream?, length: Int) = unsupported()
override fun setAsciiStream(parameterIndex: Int, x: InputStream?, length: Long) = unsupported()
override fun setAsciiStream(parameterIndex: Int, x: InputStream?) = unsupported()
override fun setNClob(parameterIndex: Int, value: NClob?) = unsupported()
override fun setNClob(parameterIndex: Int, reader: Reader?, length: Long) = unsupported()
override fun setNClob(parameterIndex: Int, reader: Reader?) = unsupported()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy