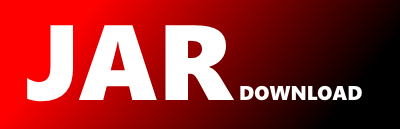
io.leopard.burrow.lang.inum.EnumUtil Maven / Gradle / Ivy
package io.leopard.burrow.lang.inum;
import java.util.EnumSet;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
public class EnumUtil {
protected static final Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy