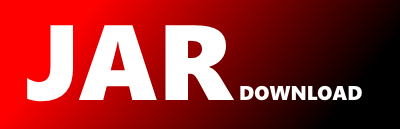
io.leoplatform.sdk.payload.EntityPayload Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leo-api Show documentation
Show all versions of leo-api Show documentation
SDK for LEO Insights platform
The newest version!
package io.leoplatform.sdk.payload;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import io.leoplatform.sdk.bus.LoadingBot;
import javax.json.JsonObject;
import java.time.Instant;
import java.util.Optional;
import static com.fasterxml.jackson.annotation.JsonInclude.Include.NON_NULL;
/**
* All payloads are wrapped in a {@code EntityPayload} object before being loaded to the LEO bus. This
* class provides metadata relating to the payload and its load process.
*/
@JsonPropertyOrder({"payload", "id", "event", "event_source_timestamp", "timestamp", "eid", "correlation_id"})
@JsonInclude(NON_NULL)
public class EntityPayload {
private final JsonObject payload;
private final String id;
private final String event;
private final Long event_source_timestamp;
private final Long timestamp;
private final StreamCorrelation correlation_id;
public EntityPayload(EventPayload eventPayload, LoadingBot bot) {
this.payload = eventPayload.payload();
this.id = bot.name();
this.event = bot.destination().name();
Instant now = Instant.now();
this.event_source_timestamp = Optional.of(eventPayload)
.map(EventPayload::eventTime)
.orElse(now)
.toEpochMilli();
this.timestamp = now.toEpochMilli();
this.correlation_id = eventPayload.streamCorrelation();
}
/**
* A JSON object to be loaded to the LEO bus. There are typically limitations on the size of this
* value based on the destination within the bus. This value is serialized (and typically gzipped)
* before load.
*
* @return A {@link JsonObject} to be loaded onto the LEO bus
*/
public JsonObject getPayload() {
return payload;
}
/**
* The ID of the bot that is creating this event.
* @return A unique ID of the creating bot
*/
public String getId() {
return id;
}
/**
* The destination queue ID.
* @return A unique ID of the destination queue
*/
public String getEvent() {
return event;
}
/**
* An optional, user-supplied claim indicating when this payload was originally created.
* This typically relates to a creation date/time in a persistent storage system.
* @return The original creation time in epoch milliseconds
*/
public Long getEvent_source_timestamp() {
return event_source_timestamp;
}
/**
* The time that this event was created just before loading it to the bus. This value is generated by the SDK.
* @return The event creation time in epoch milliseconds
*/
public Long getTimestamp() {
return timestamp;
}
/**
* Metadata related to the contents of the payload allowing the entities to be traced back to their
* original source.
* @return A {@link StreamCorrelation} instance to help identify the payload's origin
*/
public StreamCorrelation getCorrelation_id() {
return correlation_id;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy