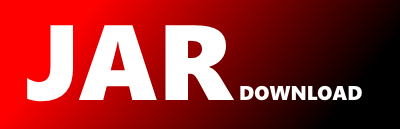
io.lettuce.core.ConnectionFuture Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lettuce-core Show documentation
Show all versions of lettuce-core Show documentation
Advanced and thread-safe Java Redis client for synchronous, asynchronous, and
reactive usage. Supports Cluster, Sentinel, Pipelining, Auto-Reconnect, Codecs
and much more.
The newest version!
package io.lettuce.core;
import java.net.SocketAddress;
import java.util.concurrent.*;
import java.util.function.BiConsumer;
import java.util.function.BiFunction;
import java.util.function.Consumer;
import java.util.function.Function;
import io.lettuce.core.api.StatefulConnection;
/**
* A {@code ConnectionFuture} represents the result of an asynchronous connection initialization. The future provides a
* {@link StatefulConnection} on successful completion. It also provides the remote {@link SocketAddress}.
*
* @since 4.4
*/
public interface ConnectionFuture extends CompletionStage, Future {
/**
* Create a {@link ConnectionFuture} given {@link SocketAddress} and {@link CompletableFuture} holding the connection
* progress.
*
* @param remoteAddress initial connection endpoint, must not be {@code null}.
* @param delegate must not be {@code null}.
* @return the {@link ConnectionFuture} for {@link SocketAddress} and {@link CompletableFuture}.
* @since 5.0
*/
static ConnectionFuture from(SocketAddress remoteAddress, CompletableFuture delegate) {
return new DefaultConnectionFuture<>(remoteAddress, delegate);
}
/**
* Create a completed {@link ConnectionFuture} given {@link SocketAddress} and {@code value} holding the value.
*
* @param remoteAddress initial connection endpoint, must not be {@code null}.
* @param value must not be {@code null}.
* @return the {@link ConnectionFuture} for {@link SocketAddress} and {@code value}.
* @since 5.1
*/
static ConnectionFuture completed(SocketAddress remoteAddress, T value) {
return new DefaultConnectionFuture<>(remoteAddress, CompletableFuture.completedFuture(value));
}
/**
* Waits if necessary for the computation to complete, and then retrieves its result.
*
* @return the computed result
* @throws CancellationException if the computation was cancelled
* @throws ExecutionException if the computation threw an exception
* @throws InterruptedException if the current thread was interrupted while waiting
*/
T get() throws InterruptedException, ExecutionException;
/**
* Return the remote {@link SocketAddress}.
*
* @return the remote {@link SocketAddress}. May be {@code null} until the socket address is resolved.
*/
SocketAddress getRemoteAddress();
/**
* Returns the result value when complete, or throws an (unchecked) exception if completed exceptionally. To better conform
* with the use of common functional forms, if a computation involved in the completion of this CompletableFuture threw an
* exception, this method throws an (unchecked) {@link CompletionException} with the underlying exception as its cause.
*
* @return the result value
* @throws CancellationException if the computation was cancelled
* @throws CompletionException if this future completed exceptionally or a completion computation threw an exception
*/
T join();
@Override
ConnectionFuture thenApply(Function super T, ? extends U> fn);
@Override
ConnectionFuture thenApplyAsync(Function super T, ? extends U> fn);
@Override
ConnectionFuture thenApplyAsync(Function super T, ? extends U> fn, Executor executor);
@Override
ConnectionFuture thenAccept(Consumer super T> action);
@Override
ConnectionFuture thenAcceptAsync(Consumer super T> action);
@Override
ConnectionFuture thenAcceptAsync(Consumer super T> action, Executor executor);
@Override
ConnectionFuture thenRun(Runnable action);
@Override
ConnectionFuture thenRunAsync(Runnable action);
@Override
ConnectionFuture thenRunAsync(Runnable action, Executor executor);
@Override
ConnectionFuture thenCombine(CompletionStage extends U> other,
BiFunction super T, ? super U, ? extends V> fn);
@Override
ConnectionFuture thenCombineAsync(CompletionStage extends U> other,
BiFunction super T, ? super U, ? extends V> fn);
@Override
ConnectionFuture thenCombineAsync(CompletionStage extends U> other,
BiFunction super T, ? super U, ? extends V> fn, Executor executor);
@Override
ConnectionFuture thenAcceptBoth(CompletionStage extends U> other, BiConsumer super T, ? super U> action);
@Override
ConnectionFuture thenAcceptBothAsync(CompletionStage extends U> other, BiConsumer super T, ? super U> action);
@Override
ConnectionFuture thenAcceptBothAsync(CompletionStage extends U> other, BiConsumer super T, ? super U> action,
Executor executor);
@Override
ConnectionFuture runAfterBoth(CompletionStage> other, Runnable action);
@Override
ConnectionFuture runAfterBothAsync(CompletionStage> other, Runnable action);
@Override
ConnectionFuture runAfterBothAsync(CompletionStage> other, Runnable action, Executor executor);
@Override
ConnectionFuture applyToEither(CompletionStage extends T> other, Function super T, U> fn);
@Override
ConnectionFuture applyToEitherAsync(CompletionStage extends T> other, Function super T, U> fn);
@Override
ConnectionFuture applyToEitherAsync(CompletionStage extends T> other, Function super T, U> fn,
Executor executor);
@Override
ConnectionFuture acceptEither(CompletionStage extends T> other, Consumer super T> action);
@Override
ConnectionFuture acceptEitherAsync(CompletionStage extends T> other, Consumer super T> action);
@Override
ConnectionFuture acceptEitherAsync(CompletionStage extends T> other, Consumer super T> action, Executor executor);
@Override
ConnectionFuture runAfterEither(CompletionStage> other, Runnable action);
@Override
ConnectionFuture runAfterEitherAsync(CompletionStage> other, Runnable action);
@Override
ConnectionFuture runAfterEitherAsync(CompletionStage> other, Runnable action, Executor executor);
@Override
ConnectionFuture thenCompose(Function super T, ? extends CompletionStage> fn);
ConnectionFuture thenCompose(BiFunction super T, ? super Throwable, ? extends CompletionStage> fn);
@Override
ConnectionFuture thenComposeAsync(Function super T, ? extends CompletionStage> fn);
@Override
ConnectionFuture thenComposeAsync(Function super T, ? extends CompletionStage> fn, Executor executor);
@Override
ConnectionFuture exceptionally(Function fn);
@Override
ConnectionFuture whenComplete(BiConsumer super T, ? super Throwable> action);
@Override
ConnectionFuture whenCompleteAsync(BiConsumer super T, ? super Throwable> action);
@Override
ConnectionFuture whenCompleteAsync(BiConsumer super T, ? super Throwable> action, Executor executor);
@Override
ConnectionFuture handle(BiFunction super T, Throwable, ? extends U> fn);
@Override
ConnectionFuture handleAsync(BiFunction super T, Throwable, ? extends U> fn);
@Override
ConnectionFuture handleAsync(BiFunction super T, Throwable, ? extends U> fn, Executor executor);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy