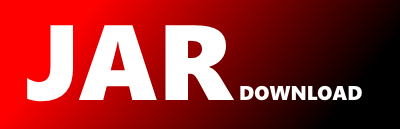
io.lettuce.core.DefaultConnectionFuture Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lettuce-core Show documentation
Show all versions of lettuce-core Show documentation
Advanced and thread-safe Java Redis client for synchronous, asynchronous, and
reactive usage. Supports Cluster, Sentinel, Pipelining, Auto-Reconnect, Codecs
and much more.
The newest version!
package io.lettuce.core;
import java.net.SocketAddress;
import java.util.concurrent.*;
import java.util.function.BiConsumer;
import java.util.function.BiFunction;
import java.util.function.Consumer;
import java.util.function.Function;
/**
* Default {@link CompletableFuture} implementation. Delegates calls to the decorated {@link CompletableFuture} and provides a
* {@link SocketAddress}.
*
* @since 4.4
*/
class DefaultConnectionFuture extends CompletableFuture implements ConnectionFuture {
private final CompletableFuture remoteAddress;
private final CompletableFuture delegate;
public DefaultConnectionFuture(SocketAddress remoteAddress, CompletableFuture delegate) {
this.remoteAddress = CompletableFuture.completedFuture(remoteAddress);
this.delegate = delegate;
}
public DefaultConnectionFuture(CompletableFuture remoteAddress, CompletableFuture delegate) {
this.remoteAddress = remoteAddress;
this.delegate = delegate;
}
public SocketAddress getRemoteAddress() {
if (remoteAddress.isDone() && !remoteAddress.isCompletedExceptionally()) {
return remoteAddress.join();
}
return null;
}
private DefaultConnectionFuture adopt(CompletableFuture newFuture) {
return new DefaultConnectionFuture<>(remoteAddress, newFuture);
}
@Override
public boolean isDone() {
return delegate.isDone();
}
@Override
public T get() throws InterruptedException, ExecutionException {
return delegate.get();
}
@Override
public T get(long timeout, TimeUnit unit) throws InterruptedException, ExecutionException, TimeoutException {
return delegate.get(timeout, unit);
}
@Override
public T join() {
return delegate.join();
}
@Override
public T getNow(T valueIfAbsent) {
return delegate.getNow(valueIfAbsent);
}
@Override
public boolean complete(T value) {
return delegate.complete(value);
}
@Override
public boolean completeExceptionally(Throwable ex) {
return delegate.completeExceptionally(ex);
}
@Override
public DefaultConnectionFuture thenApply(Function super T, ? extends U> fn) {
return adopt(delegate.thenApply(fn));
}
@Override
public DefaultConnectionFuture thenApplyAsync(Function super T, ? extends U> fn) {
return adopt(delegate.thenApplyAsync(fn));
}
@Override
public DefaultConnectionFuture thenApplyAsync(Function super T, ? extends U> fn, Executor executor) {
return adopt(delegate.thenApplyAsync(fn, executor));
}
@Override
public DefaultConnectionFuture thenAccept(Consumer super T> action) {
return adopt(delegate.thenAccept(action));
}
@Override
public DefaultConnectionFuture thenAcceptAsync(Consumer super T> action) {
return adopt(delegate.thenAcceptAsync(action));
}
@Override
public DefaultConnectionFuture thenAcceptAsync(Consumer super T> action, Executor executor) {
return adopt(delegate.thenAcceptAsync(action, executor));
}
@Override
public DefaultConnectionFuture thenRun(Runnable action) {
return adopt(delegate.thenRun(action));
}
@Override
public DefaultConnectionFuture thenRunAsync(Runnable action) {
return adopt(delegate.thenRunAsync(action));
}
@Override
public DefaultConnectionFuture thenRunAsync(Runnable action, Executor executor) {
return adopt(delegate.thenRunAsync(action, executor));
}
@Override
public DefaultConnectionFuture thenCombine(CompletionStage extends U> other,
BiFunction super T, ? super U, ? extends V> fn) {
return adopt(delegate.thenCombine(other, fn));
}
@Override
public DefaultConnectionFuture thenCombineAsync(CompletionStage extends U> other,
BiFunction super T, ? super U, ? extends V> fn) {
return adopt(delegate.thenCombineAsync(other, fn));
}
@Override
public DefaultConnectionFuture thenCombineAsync(CompletionStage extends U> other,
BiFunction super T, ? super U, ? extends V> fn, Executor executor) {
return adopt(delegate.thenCombineAsync(other, fn, executor));
}
@Override
public DefaultConnectionFuture thenAcceptBoth(CompletionStage extends U> other,
BiConsumer super T, ? super U> action) {
return adopt(delegate.thenAcceptBoth(other, action));
}
@Override
public DefaultConnectionFuture thenAcceptBothAsync(CompletionStage extends U> other,
BiConsumer super T, ? super U> action) {
return adopt(delegate.thenAcceptBothAsync(other, action));
}
@Override
public DefaultConnectionFuture thenAcceptBothAsync(CompletionStage extends U> other,
BiConsumer super T, ? super U> action, Executor executor) {
return adopt(delegate.thenAcceptBothAsync(other, action, executor));
}
@Override
public DefaultConnectionFuture runAfterBoth(CompletionStage> other, Runnable action) {
return adopt(delegate.runAfterBoth(other, action));
}
@Override
public DefaultConnectionFuture runAfterBothAsync(CompletionStage> other, Runnable action) {
return adopt(delegate.runAfterBothAsync(other, action));
}
@Override
public DefaultConnectionFuture runAfterBothAsync(CompletionStage> other, Runnable action, Executor executor) {
return adopt(delegate.runAfterBothAsync(other, action, executor));
}
@Override
public DefaultConnectionFuture applyToEither(CompletionStage extends T> other, Function super T, U> fn) {
return adopt(delegate.applyToEither(other, fn));
}
@Override
public DefaultConnectionFuture applyToEitherAsync(CompletionStage extends T> other, Function super T, U> fn) {
return adopt(delegate.applyToEitherAsync(other, fn));
}
@Override
public DefaultConnectionFuture applyToEitherAsync(CompletionStage extends T> other, Function super T, U> fn,
Executor executor) {
return adopt(delegate.applyToEitherAsync(other, fn, executor));
}
@Override
public DefaultConnectionFuture acceptEither(CompletionStage extends T> other, Consumer super T> action) {
return adopt(delegate.acceptEither(other, action));
}
@Override
public DefaultConnectionFuture acceptEitherAsync(CompletionStage extends T> other, Consumer super T> action) {
return adopt(delegate.acceptEitherAsync(other, action));
}
@Override
public DefaultConnectionFuture acceptEitherAsync(CompletionStage extends T> other, Consumer super T> action,
Executor executor) {
return adopt(delegate.acceptEitherAsync(other, action, executor));
}
@Override
public DefaultConnectionFuture runAfterEither(CompletionStage> other, Runnable action) {
return adopt(delegate.runAfterEither(other, action));
}
@Override
public DefaultConnectionFuture runAfterEitherAsync(CompletionStage> other, Runnable action) {
return adopt(delegate.runAfterEitherAsync(other, action));
}
@Override
public DefaultConnectionFuture runAfterEitherAsync(CompletionStage> other, Runnable action, Executor executor) {
return adopt(delegate.runAfterEitherAsync(other, action, executor));
}
@Override
public DefaultConnectionFuture thenCompose(Function super T, ? extends CompletionStage> fn) {
return adopt(delegate.thenCompose(fn));
}
@Override
public ConnectionFuture thenCompose(BiFunction super T, ? super Throwable, ? extends CompletionStage> fn) {
CompletableFuture future = new CompletableFuture<>();
delegate.whenComplete((v, e) -> {
try {
CompletionStage apply = fn.apply(v, e);
apply.whenComplete((u, t) -> {
if (t != null) {
future.completeExceptionally(t);
} else {
future.complete(u);
}
});
} catch (Exception ex) {
ExecutionException result = new ExecutionException("Exception while applying thenCompose", ex);
if (e != null) {
result.addSuppressed(e);
}
future.completeExceptionally(result);
}
});
return adopt(future);
}
@Override
public DefaultConnectionFuture thenComposeAsync(Function super T, ? extends CompletionStage> fn) {
return adopt(delegate.thenComposeAsync(fn));
}
@Override
public DefaultConnectionFuture thenComposeAsync(Function super T, ? extends CompletionStage> fn,
Executor executor) {
return adopt(delegate.thenComposeAsync(fn, executor));
}
@Override
public DefaultConnectionFuture whenComplete(BiConsumer super T, ? super Throwable> action) {
return adopt(delegate.whenComplete(action));
}
@Override
public DefaultConnectionFuture whenCompleteAsync(BiConsumer super T, ? super Throwable> action) {
return adopt(delegate.whenCompleteAsync(action));
}
@Override
public DefaultConnectionFuture whenCompleteAsync(BiConsumer super T, ? super Throwable> action, Executor executor) {
return adopt(delegate.whenCompleteAsync(action, executor));
}
@Override
public DefaultConnectionFuture handle(BiFunction super T, Throwable, ? extends U> fn) {
return adopt(delegate.handle(fn));
}
@Override
public DefaultConnectionFuture handleAsync(BiFunction super T, Throwable, ? extends U> fn) {
return adopt(delegate.handleAsync(fn));
}
@Override
public DefaultConnectionFuture handleAsync(BiFunction super T, Throwable, ? extends U> fn, Executor executor) {
return adopt(delegate.handleAsync(fn, executor));
}
@Override
public CompletableFuture toCompletableFuture() {
return delegate.toCompletableFuture();
}
@Override
public DefaultConnectionFuture exceptionally(Function fn) {
return adopt(delegate.exceptionally(fn));
}
@Override
public boolean cancel(boolean mayInterruptIfRunning) {
return delegate.cancel(mayInterruptIfRunning);
}
@Override
public boolean isCancelled() {
return delegate.isCancelled();
}
@Override
public boolean isCompletedExceptionally() {
return delegate.isCompletedExceptionally();
}
@Override
public void obtrudeValue(T value) {
delegate.obtrudeValue(value);
}
@Override
public void obtrudeException(Throwable ex) {
delegate.obtrudeException(ex);
}
@Override
public int getNumberOfDependents() {
return delegate.getNumberOfDependents();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy