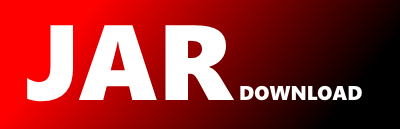
io.lettuce.core.GeoValue Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lettuce-core Show documentation
Show all versions of lettuce-core Show documentation
Advanced and thread-safe Java Redis client for synchronous, asynchronous, and
reactive usage. Supports Cluster, Sentinel, Pipelining, Auto-Reconnect, Codecs
and much more.
The newest version!
package io.lettuce.core;
import java.util.NoSuchElementException;
import java.util.Objects;
import java.util.Optional;
import java.util.function.Function;
import io.lettuce.core.internal.LettuceAssert;
/**
* A Geo value extension to {@link Value}.
*
* @param Value type.
* @author Mark Paluch
* @since 6.1
*/
public class GeoValue extends Value {
private final GeoCoordinates coordinates;
/**
* Serializable constructor.
*/
protected GeoValue() {
super(null);
this.coordinates = null;
}
private GeoValue(GeoCoordinates coordinates, V value) {
super(value);
this.coordinates = coordinates;
}
/**
* Creates a {@link Value} from a {@code key} and an {@link Optional}. The resulting value contains the value from the
* {@link Optional} if a value is present. Value is empty if the {@link Optional} is empty.
*
* @param coordinates the score.
* @param optional the optional. May be empty but never {@code null}.
* @return the {@link Value}.
*/
public static Value from(GeoCoordinates coordinates, Optional optional) {
LettuceAssert.notNull(optional, "Optional must not be null");
if (optional.isPresent()) {
LettuceAssert.notNull(coordinates, "GeoCoordinates must not be null");
return new GeoValue<>(coordinates, optional.get());
}
return Value.empty();
}
/**
* Creates a {@link Value} from a {@code coordinates} and {@code value}. The resulting value contains the value if the
* {@code value} is not null.
*
* @param coordinates the coordinates.
* @param value the value. May be {@code null}.
* @return the {@link Value}.
*/
public static Value fromNullable(GeoCoordinates coordinates, T value) {
if (value == null) {
return empty();
}
LettuceAssert.notNull(coordinates, "GeoCoordinates must not be null");
return new GeoValue<>(coordinates, value);
}
/**
* Creates a {@link GeoValue} from a {@code key} and {@code value}. The resulting value contains the value.
*
* @param longitude the longitude coordinate according to WGS84.
* @param latitude the latitude coordinate according to WGS84.
* @param value the value. Must not be {@code null}.
* @return the {@link GeoValue}
*/
public static GeoValue just(double longitude, double latitude, T value) {
return new GeoValue<>(new GeoCoordinates(longitude, latitude), value);
}
/**
* Creates a {@link GeoValue} from a {@code key} and {@code value}. The resulting value contains the value.
*
* @param coordinates the coordinates.
* @param value the value. Must not be {@code null}.
* @return the {@link GeoValue}.
*/
public static GeoValue just(GeoCoordinates coordinates, T value) {
LettuceAssert.notNull(coordinates, "GeoCoordinates must not be null");
return new GeoValue<>(coordinates, value);
}
public GeoCoordinates getCoordinates() {
return coordinates;
}
/**
* @return the longitude if this instance has a {@link #hasValue()}.
* @throws NoSuchElementException if the value is not present.
*/
public double getLongitude() {
if (coordinates == null) {
throw new NoSuchElementException();
}
return coordinates.getX().doubleValue();
}
/**
* @return the latitude if this instance has a {@link #hasValue()}.
* @throws NoSuchElementException if the value is not present.
*/
public double getLatitude() {
if (coordinates == null) {
throw new NoSuchElementException();
}
return coordinates.getY().doubleValue();
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof GeoValue)) {
return false;
}
if (!super.equals(o)) {
return false;
}
GeoValue> geoValue = (GeoValue>) o;
return Objects.equals(coordinates, geoValue.coordinates);
}
@Override
public int hashCode() {
return Objects.hash(super.hashCode(), coordinates);
}
@Override
public String toString() {
return hasValue() ? String.format("GeoValue[%s, %s]", coordinates, getValue())
: String.format("GeoValue[%s].empty", coordinates);
}
/**
* Returns a {@link GeoValue} consisting of the results of applying the given function to the value of this element. Mapping
* is performed only if a {@link #hasValue() value is present}.
*
* @param element type of the new {@link GeoValue}.
* @param mapper a stateless function to apply to each element.
* @return the new {@link GeoValue}.
*/
@SuppressWarnings("unchecked")
public GeoValue map(Function super V, ? extends R> mapper) {
LettuceAssert.notNull(mapper, "Mapper function must not be null");
if (hasValue()) {
return new GeoValue<>(coordinates, mapper.apply(getValue()));
}
return (GeoValue) this;
}
/**
* Returns a {@link GeoValue} consisting of the results of applying the given function to the {@link GeoCoordinates} of this
* element. Mapping is performed only if a {@link #hasValue() value is present}.
*
* @param mapper a stateless function to apply to each element.
* @return the new {@link GeoValue}.
*/
public GeoValue mapCoordinates(Function super GeoCoordinates, ? extends GeoCoordinates> mapper) {
LettuceAssert.notNull(mapper, "Mapper function must not be null");
if (hasValue()) {
return new GeoValue<>(mapper.apply(coordinates), getValue());
}
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy