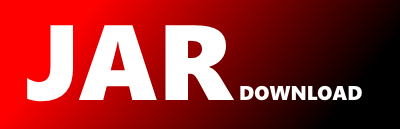
io.lettuce.core.RedisCommandBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lettuce-core Show documentation
Show all versions of lettuce-core Show documentation
Advanced and thread-safe Java Redis client for synchronous, asynchronous, and
reactive usage. Supports Cluster, Sentinel, Pipelining, Auto-Reconnect, Codecs
and much more.
The newest version!
/*
* Copyright 2011-Present, Redis Ltd. and Contributors
* All rights reserved.
*
* Licensed under the MIT License.
*
* This file contains contributions from third-party contributors
* licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.lettuce.core;
import io.lettuce.core.Range.Boundary;
import io.lettuce.core.XReadArgs.StreamOffset;
import io.lettuce.core.codec.RedisCodec;
import io.lettuce.core.codec.StringCodec;
import io.lettuce.core.internal.LettuceAssert;
import io.lettuce.core.models.stream.ClaimedMessages;
import io.lettuce.core.models.stream.PendingMessage;
import io.lettuce.core.models.stream.PendingMessages;
import io.lettuce.core.output.*;
import io.lettuce.core.protocol.BaseRedisCommandBuilder;
import io.lettuce.core.protocol.Command;
import io.lettuce.core.protocol.CommandArgs;
import io.lettuce.core.protocol.CommandKeyword;
import io.lettuce.core.protocol.CommandType;
import io.lettuce.core.protocol.RedisCommand;
import java.nio.ByteBuffer;
import java.util.Arrays;
import java.util.Date;
import java.util.List;
import java.util.Map;
import java.util.Set;
import static io.lettuce.core.internal.LettuceStrings.string;
import static io.lettuce.core.protocol.CommandKeyword.*;
import static io.lettuce.core.protocol.CommandType.*;
import static io.lettuce.core.protocol.CommandType.COPY;
import static io.lettuce.core.protocol.CommandType.SAVE;
/**
* @param
* @param
* @author Mark Paluch
* @author Zhang Jessey
* @author Tugdual Grall
* @author dengliming
* @author Mikhael Sokolov
* @author Tihomir Mateev
* @author Ali Takavci
* @author Seonghwan Lee
*/
@SuppressWarnings({ "unchecked", "varargs" })
class RedisCommandBuilder extends BaseRedisCommandBuilder {
RedisCommandBuilder(RedisCodec codec) {
super(codec);
}
Command> aclCat() {
CommandArgs args = new CommandArgs<>(codec);
args.add(CAT);
return createCommand(ACL, new EnumSetOutput<>(codec, AclCategory.class, String::toUpperCase, it -> null), args);
}
Command> aclCat(AclCategory category) {
LettuceAssert.notNull(category, "Category " + MUST_NOT_BE_NULL);
CommandArgs args = new CommandArgs<>(codec);
args.add(CAT).add(category.name().toLowerCase());
return createCommand(ACL, new EnumSetOutput<>(codec, CommandType.class, String::toUpperCase, it -> null), args);
}
Command aclDeluser(String... usernames) {
notEmpty(usernames);
CommandArgs args = new CommandArgs<>(codec);
args.add(DELUSER);
for (String username : usernames) {
args.add(username);
}
return createCommand(ACL, new IntegerOutput<>(codec), args);
}
Command aclDryRun(String username, String command, String... commandArgs) {
LettuceAssert.notNull(username, "username " + MUST_NOT_BE_NULL);
LettuceAssert.notNull(command, "command " + MUST_NOT_BE_NULL);
CommandArgs args = new CommandArgs<>(codec);
args.add(DRYRUN).add(username).add(command);
for (String commandArg : commandArgs) {
args.add(commandArg);
}
return createCommand(ACL, new StatusOutput<>(codec), args);
}
Command aclDryRun(String username, RedisCommand command) {
LettuceAssert.notNull(username, "username " + MUST_NOT_BE_NULL);
LettuceAssert.notNull(command, "command " + MUST_NOT_BE_NULL);
CommandArgs args = new CommandArgs<>(codec);
args.add(DRYRUN).add(username).add(command.getType()).addAll(command.getArgs());
return createCommand(ACL, new StatusOutput<>(codec), args);
}
Command aclGenpass() {
CommandArgs args = new CommandArgs<>(codec);
args.add(GENPASS);
return createCommand(ACL, new StatusOutput<>(codec), args);
}
Command aclGenpass(int bits) {
CommandArgs args = new CommandArgs<>(codec);
args.add(GENPASS).add(bits);
return createCommand(ACL, new StatusOutput<>(codec), args);
}
Command> aclGetuser(String username) {
LettuceAssert.notNull(username, "Username " + MUST_NOT_BE_NULL);
CommandArgs args = new CommandArgs<>(codec);
args.add(GETUSER).add(username);
return createCommand(ACL, new NestedMultiOutput<>(codec), args);
}
Command> aclList() {
CommandArgs args = new CommandArgs<>(codec);
args.add(LIST);
return createCommand(ACL, new StringListOutput<>(codec), args);
}
Command aclLoad() {
CommandArgs args = new CommandArgs<>(codec);
args.add(LOAD);
return createCommand(ACL, new StatusOutput<>(codec), args);
}
Command>> aclLog() {
CommandArgs args = new CommandArgs<>(codec);
args.add(LOG);
return new Command(ACL, new ListOfGenericMapsOutput<>(StringCodec.ASCII), args);
}
Command>> aclLog(int count) {
CommandArgs args = new CommandArgs<>(codec);
args.add(LOG).add(count);
return new Command(ACL, new ListOfGenericMapsOutput<>(StringCodec.ASCII), args);
}
Command aclLogReset() {
CommandArgs args = new CommandArgs<>(codec);
args.add(LOG).add(RESET);
return createCommand(ACL, new StatusOutput<>(codec), args);
}
Command aclSave() {
CommandArgs args = new CommandArgs<>(codec);
args.add(CommandKeyword.SAVE);
return createCommand(ACL, new StatusOutput<>(codec), args);
}
Command aclSetuser(String username, AclSetuserArgs setuserArgs) {
notNullKey(username);
CommandArgs args = new CommandArgs<>(codec);
args.add(SETUSER).add(username);
setuserArgs.build(args);
return createCommand(ACL, new StatusOutput<>(codec), args);
}
Command> aclUsers() {
CommandArgs args = new CommandArgs<>(codec);
args.add(USERS);
return createCommand(ACL, new StringListOutput<>(codec), args);
}
Command aclWhoami() {
CommandArgs args = new CommandArgs<>(codec);
args.add(WHOAMI);
return createCommand(ACL, new StatusOutput<>(codec), args);
}
Command append(K key, V value) {
notNullKey(key);
return createCommand(APPEND, new IntegerOutput<>(codec), key, value);
}
Command asking() {
CommandArgs args = new CommandArgs<>(codec);
return createCommand(ASKING, new StatusOutput<>(codec), args);
}
Command auth(CharSequence password) {
LettuceAssert.notNull(password, "Password " + MUST_NOT_BE_NULL);
char[] chars = new char[password.length()];
for (int i = 0; i < password.length(); i++) {
chars[i] = password.charAt(i);
}
return auth(chars);
}
Command auth(char[] password) {
LettuceAssert.notNull(password, "Password " + MUST_NOT_BE_NULL);
CommandArgs args = new CommandArgs<>(codec).add(password);
return createCommand(AUTH, new StatusOutput<>(codec), args);
}
Command auth(String username, CharSequence password) {
LettuceAssert.notNull(username, "Username " + MUST_NOT_BE_NULL);
LettuceAssert.isTrue(!username.isEmpty(), "Username " + MUST_NOT_BE_EMPTY);
LettuceAssert.notNull(password, "Password " + MUST_NOT_BE_NULL);
char[] chars = new char[password.length()];
for (int i = 0; i < password.length(); i++) {
chars[i] = password.charAt(i);
}
return auth(username, chars);
}
Command auth(String username, char[] password) {
LettuceAssert.notNull(username, "Username " + MUST_NOT_BE_NULL);
LettuceAssert.isTrue(!username.isEmpty(), "Username " + MUST_NOT_BE_EMPTY);
LettuceAssert.notNull(password, "Password " + MUST_NOT_BE_NULL);
CommandArgs args = new CommandArgs<>(codec).add(username).add(password);
return createCommand(AUTH, new StatusOutput<>(codec), args);
}
Command bgrewriteaof() {
return createCommand(BGREWRITEAOF, new StatusOutput<>(codec));
}
Command bgsave() {
return createCommand(BGSAVE, new StatusOutput<>(codec));
}
Command bitcount(K key) {
notNullKey(key);
CommandArgs args = new CommandArgs<>(codec).addKey(key);
return createCommand(BITCOUNT, new IntegerOutput<>(codec), args);
}
Command bitcount(K key, long start, long end) {
notNullKey(key);
CommandArgs args = new CommandArgs<>(codec);
args.addKey(key).add(start).add(end);
return createCommand(BITCOUNT, new IntegerOutput<>(codec), args);
}
Command> bitfield(K key, BitFieldArgs bitFieldArgs) {
notNullKey(key);
LettuceAssert.notNull(bitFieldArgs, "BitFieldArgs must not be null");
CommandArgs args = new CommandArgs<>(codec);
args.addKey(key);
bitFieldArgs.build(args);
return createCommand(BITFIELD, (CommandOutput) new ArrayOutput<>(codec), args);
}
Command>> bitfieldValue(K key, BitFieldArgs bitFieldArgs) {
notNullKey(key);
LettuceAssert.notNull(bitFieldArgs, "BitFieldArgs must not be null");
CommandArgs args = new CommandArgs<>(codec);
args.addKey(key);
bitFieldArgs.build(args);
return createCommand(BITFIELD, (CommandOutput) new ValueValueListOutput<>(codec), args);
}
Command bitopAnd(K destination, K... keys) {
LettuceAssert.notNull(destination, "Destination " + MUST_NOT_BE_NULL);
notEmpty(keys);
CommandArgs args = new CommandArgs<>(codec);
args.add(AND).addKey(destination).addKeys(keys);
return createCommand(BITOP, new IntegerOutput<>(codec), args);
}
Command bitopNot(K destination, K source) {
LettuceAssert.notNull(destination, "Destination " + MUST_NOT_BE_NULL);
LettuceAssert.notNull(source, "Source " + MUST_NOT_BE_NULL);
CommandArgs args = new CommandArgs<>(codec);
args.add(NOT).addKey(destination).addKey(source);
return createCommand(BITOP, new IntegerOutput<>(codec), args);
}
Command bitopOr(K destination, K... keys) {
LettuceAssert.notNull(destination, "Destination " + MUST_NOT_BE_NULL);
notEmpty(keys);
CommandArgs args = new CommandArgs<>(codec);
args.add(OR).addKey(destination).addKeys(keys);
return createCommand(BITOP, new IntegerOutput<>(codec), args);
}
Command bitopXor(K destination, K... keys) {
LettuceAssert.notNull(destination, "Destination " + MUST_NOT_BE_NULL);
notEmpty(keys);
CommandArgs args = new CommandArgs<>(codec);
args.add(XOR).addKey(destination).addKeys(keys);
return createCommand(BITOP, new IntegerOutput<>(codec), args);
}
Command bitpos(K key, boolean state) {
notNullKey(key);
CommandArgs args = new CommandArgs<>(codec);
args.addKey(key).add(state ? 1 : 0);
return createCommand(BITPOS, new IntegerOutput<>(codec), args);
}
Command bitpos(K key, boolean state, long start) {
notNullKey(key);
CommandArgs args = new CommandArgs<>(codec);
args.addKey(key).add(state ? 1 : 0).add(start);
return createCommand(BITPOS, new IntegerOutput<>(codec), args);
}
Command bitpos(K key, boolean state, long start, long end) {
notNullKey(key);
CommandArgs args = new CommandArgs<>(codec);
args.addKey(key).add(state ? 1 : 0).add(start).add(end);
return createCommand(BITPOS, new IntegerOutput<>(codec), args);
}
Command blmove(K source, K destination, LMoveArgs lMoveArgs, long timeout) {
LettuceAssert.notNull(source, "Source " + MUST_NOT_BE_NULL);
LettuceAssert.notNull(destination, "Destination " + MUST_NOT_BE_NULL);
LettuceAssert.notNull(lMoveArgs, "LMoveArgs " + MUST_NOT_BE_NULL);
CommandArgs args = new CommandArgs<>(codec);
args.addKey(source).addKey(destination);
lMoveArgs.build(args);
args.add(timeout);
return createCommand(BLMOVE, new ValueOutput<>(codec), args);
}
Command blmove(K source, K destination, LMoveArgs lMoveArgs, double timeout) {
LettuceAssert.notNull(source, "Source " + MUST_NOT_BE_NULL);
LettuceAssert.notNull(destination, "Destination " + MUST_NOT_BE_NULL);
LettuceAssert.notNull(lMoveArgs, "LMoveArgs " + MUST_NOT_BE_NULL);
CommandArgs args = new CommandArgs<>(codec);
args.addKey(source).addKey(destination);
lMoveArgs.build(args);
args.add(timeout);
return createCommand(BLMOVE, new ValueOutput<>(codec), args);
}
Command>> blmpop(long timeout, LMPopArgs lmPopArgs, K... keys) {
LettuceAssert.notNull(keys, "Keys " + MUST_NOT_BE_NULL);
LettuceAssert.notNull(lmPopArgs, "LMPopArgs " + MUST_NOT_BE_NULL);
CommandArgs args = new CommandArgs<>(codec).add(timeout).add(keys.length).addKeys(keys);
lmPopArgs.build(args);
return createCommand(BLMPOP, new KeyValueValueListOutput<>(codec), args);
}
Command>> blmpop(double timeout, LMPopArgs lmPopArgs, K... keys) {
LettuceAssert.notNull(keys, "Keys " + MUST_NOT_BE_NULL);
LettuceAssert.notNull(lmPopArgs, "LMPopArgs " + MUST_NOT_BE_NULL);
CommandArgs args = new CommandArgs<>(codec).add(timeout).add(keys.length).addKeys(keys);
lmPopArgs.build(args);
return createCommand(BLMPOP, new KeyValueValueListOutput<>(codec), args);
}
Command> blpop(long timeout, K... keys) {
notEmpty(keys);
CommandArgs args = new CommandArgs<>(codec).addKeys(keys).add(timeout);
return createCommand(BLPOP, new KeyValueOutput<>(codec), args);
}
Command> blpop(double timeout, K... keys) {
notEmpty(keys);
CommandArgs args = new CommandArgs<>(codec).addKeys(keys).add(timeout);
return createCommand(BLPOP, new KeyValueOutput<>(codec), args);
}
Command> brpop(long timeout, K... keys) {
notEmpty(keys);
CommandArgs args = new CommandArgs<>(codec).addKeys(keys).add(timeout);
return createCommand(BRPOP, new KeyValueOutput<>(codec), args);
}
Command> brpop(double timeout, K... keys) {
notEmpty(keys);
CommandArgs args = new CommandArgs<>(codec).addKeys(keys).add(timeout);
return createCommand(BRPOP, new KeyValueOutput<>(codec), args);
}
Command brpoplpush(long timeout, K source, K destination) {
LettuceAssert.notNull(source, "Source " + MUST_NOT_BE_NULL);
LettuceAssert.notNull(destination, "Destination " + MUST_NOT_BE_NULL);
CommandArgs args = new CommandArgs<>(codec);
args.addKey(source).addKey(destination).add(timeout);
return createCommand(BRPOPLPUSH, new ValueOutput<>(codec), args);
}
Command brpoplpush(double timeout, K source, K destination) {
LettuceAssert.notNull(source, "Source " + MUST_NOT_BE_NULL);
LettuceAssert.notNull(destination, "Destination " + MUST_NOT_BE_NULL);
CommandArgs args = new CommandArgs<>(codec);
args.addKey(source).addKey(destination).add(timeout);
return createCommand(BRPOPLPUSH, new ValueOutput<>(codec), args);
}
Command clientCaching(boolean enabled) {
CommandArgs args = new CommandArgs<>(codec).add(CACHING).add(enabled ? YES : NO);
return createCommand(CLIENT, new StatusOutput<>(codec), args);
}
Command clientGetname() {
CommandArgs args = new CommandArgs<>(codec).add(GETNAME);
return createCommand(CLIENT, new KeyOutput<>(codec), args);
}
Command clientGetredir() {
CommandArgs args = new CommandArgs<>(codec).add(GETREDIR);
return createCommand(CLIENT, new IntegerOutput<>(codec), args);
}
Command clientKill(String addr) {
LettuceAssert.notNull(addr, "Addr " + MUST_NOT_BE_NULL);
LettuceAssert.notEmpty(addr, "Addr " + MUST_NOT_BE_EMPTY);
CommandArgs args = new CommandArgs<>(codec).add(KILL).add(addr);
return createCommand(CLIENT, new StatusOutput<>(codec), args);
}
Command clientKill(KillArgs killArgs) {
LettuceAssert.notNull(killArgs, "KillArgs " + MUST_NOT_BE_NULL);
CommandArgs args = new CommandArgs<>(codec).add(KILL);
killArgs.build(args);
return createCommand(CLIENT, new IntegerOutput<>(codec), args);
}
Command clientList() {
CommandArgs args = new CommandArgs<>(codec).add(LIST);
return createCommand(CLIENT, new StatusOutput<>(codec), args);
}
Command clientList(ClientListArgs clientListArgs) {
LettuceAssert.notNull(clientListArgs, "ClientListArgs " + MUST_NOT_BE_NULL);
CommandArgs args = new CommandArgs<>(codec).add(LIST);
clientListArgs.build(args);
return createCommand(CLIENT, new StatusOutput<>(codec), args);
}
Command clientInfo() {
CommandArgs args = new CommandArgs<>(codec).add(CommandKeyword.INFO);
return createCommand(CLIENT, new StatusOutput<>(codec), args);
}
Command clientNoEvict(boolean on) {
CommandArgs args = new CommandArgs<>(codec).add("NO-EVICT").add(on ? ON : OFF);
return createCommand(CLIENT, new StatusOutput<>(codec), args);
}
Command clientId() {
CommandArgs args = new CommandArgs<>(codec).add(ID);
return createCommand(CLIENT, new IntegerOutput<>(codec), args);
}
Command clientPause(long timeout) {
CommandArgs args = new CommandArgs<>(codec).add(PAUSE).add(timeout);
return createCommand(CLIENT, new StatusOutput<>(codec), args);
}
Command clientSetname(K name) {
LettuceAssert.notNull(name, "Name " + MUST_NOT_BE_NULL);
CommandArgs args = new CommandArgs<>(codec).add(SETNAME).addKey(name);
return createCommand(CLIENT, new StatusOutput<>(codec), args);
}
Command clientSetinfo(String key, String value) {
CommandArgs args = new CommandArgs<>(codec).add(SETINFO).add(key).add(value);
return createCommand(CLIENT, new StatusOutput<>(codec), args);
}
Command clientTracking(TrackingArgs trackingArgs) {
LettuceAssert.notNull(trackingArgs, "TrackingArgs " + MUST_NOT_BE_NULL);
CommandArgs args = new CommandArgs<>(codec).add(TRACKING);
trackingArgs.build(args);
return createCommand(CLIENT, new StatusOutput<>(codec), args);
}
Command clientTrackinginfo() {
CommandArgs args = new CommandArgs<>(codec).add(TRACKINGINFO);
return new Command<>(CLIENT, new ComplexOutput<>(codec, TrackingInfoParser.INSTANCE), args);
}
Command clientUnblock(long id, UnblockType type) {
LettuceAssert.notNull(type, "UnblockType " + MUST_NOT_BE_NULL);
CommandArgs args = new CommandArgs<>(codec).add(UNBLOCK).add(id).add(type);
return createCommand(CLIENT, new IntegerOutput<>(codec), args);
}
Command clusterAddslots(int[] slots) {
notEmptySlots(slots);
CommandArgs args = new CommandArgs<>(codec).add(ADDSLOTS);
for (int slot : slots) {
args.add(slot);
}
return createCommand(CLUSTER, new StatusOutput<>(codec), args);
}
Command clusterAddSlotsRange(Range... ranges) {
notEmptyRanges(ranges);
CommandArgs args = new CommandArgs<>(codec).add(ADDSLOTSRANGE);
for (Range range : ranges) {
args.add(range.getLower().getValue());
args.add(range.getUpper().getValue());
}
return createCommand(CLUSTER, new StatusOutput<>(codec), args);
}
Command clusterBumpepoch() {
CommandArgs args = new CommandArgs<>(codec).add(BUMPEPOCH);
return createCommand(CLUSTER, new StatusOutput<>(codec), args);
}
Command clusterCountFailureReports(String nodeId) {
assertNodeId(nodeId);
CommandArgs args = new CommandArgs<>(codec).add("COUNT-FAILURE-REPORTS").add(nodeId);
return createCommand(CLUSTER, new IntegerOutput<>(codec), args);
}
Command clusterCountKeysInSlot(int slot) {
CommandArgs args = new CommandArgs<>(codec).add(COUNTKEYSINSLOT).add(slot);
return createCommand(CLUSTER, new IntegerOutput<>(codec), args);
}
Command clusterDelslots(int[] slots) {
notEmptySlots(slots);
CommandArgs args = new CommandArgs<>(codec).add(DELSLOTS);
for (int slot : slots) {
args.add(slot);
}
return createCommand(CLUSTER, new StatusOutput<>(codec), args);
}
Command clusterDelSlotsRange(Range... ranges) {
notEmptyRanges(ranges);
CommandArgs args = new CommandArgs<>(codec).add(DELSLOTSRANGE);
for (Range range : ranges) {
args.add(range.getLower().getValue());
args.add(range.getUpper().getValue());
}
return createCommand(CLUSTER, new StatusOutput<>(codec), args);
}
Command clusterFailover(boolean force) {
return clusterFailover(force, false);
}
Command clusterFailover(boolean force, boolean takeOver) {
CommandArgs args = new CommandArgs<>(codec).add(FAILOVER);
if (force) {
args.add(FORCE);
} else if (takeOver) {
args.add(TAKEOVER);
}
return createCommand(CLUSTER, new StatusOutput<>(codec), args);
}
Command clusterFlushslots() {
CommandArgs args = new CommandArgs<>(codec).add(FLUSHSLOTS);
return createCommand(CLUSTER, new StatusOutput<>(codec), args);
}
Command clusterForget(String nodeId) {
assertNodeId(nodeId);
CommandArgs args = new CommandArgs<>(codec).add(FORGET).add(nodeId);
return createCommand(CLUSTER, new StatusOutput<>(codec), args);
}
Command> clusterGetKeysInSlot(int slot, int count) {
CommandArgs args = new CommandArgs<>(codec).add(GETKEYSINSLOT).add(slot).add(count);
return createCommand(CLUSTER, new KeyListOutput<>(codec), args);
}
Command clusterInfo() {
CommandArgs args = new CommandArgs<>(codec).add(CommandType.INFO);
return createCommand(CLUSTER, new StatusOutput<>(codec), args);
}
Command clusterKeyslot(K key) {
CommandArgs args = new CommandArgs<>(codec).add(KEYSLOT).addKey(key);
return createCommand(CLUSTER, new IntegerOutput<>(codec), args);
}
Command clusterMeet(String ip, int port) {
LettuceAssert.notNull(ip, "IP " + MUST_NOT_BE_NULL);
LettuceAssert.notEmpty(ip, "IP " + MUST_NOT_BE_EMPTY);
CommandArgs args = new CommandArgs<>(codec).add(MEET).add(ip).add(port);
return createCommand(CLUSTER, new StatusOutput<>(codec), args);
}
Command clusterMyId() {
CommandArgs args = new CommandArgs<>(codec).add(MYID);
return createCommand(CLUSTER, new StatusOutput<>(codec), args);
}
Command clusterMyShardId() {
CommandArgs args = new CommandArgs<>(codec).add(MYSHARDID);
return createCommand(CLUSTER, new StatusOutput<>(codec), args);
}
Command clusterNodes() {
CommandArgs args = new CommandArgs<>(codec).add(NODES);
return createCommand(CLUSTER, new StatusOutput<>(codec), args);
}
Command clusterReplicate(String nodeId) {
assertNodeId(nodeId);
CommandArgs args = new CommandArgs<>(codec).add(REPLICATE).add(nodeId);
return createCommand(CLUSTER, new StatusOutput<>(codec), args);
}
Command> clusterReplicas(String nodeId) {
assertNodeId(nodeId);
CommandArgs args = new CommandArgs<>(codec).add(REPLICAS).add(nodeId);
return createCommand(CLUSTER, new StringListOutput<>(codec), args);
}
Command clusterReset(boolean hard) {
CommandArgs args = new CommandArgs<>(codec).add(RESET);
if (hard) {
args.add(HARD);
} else {
args.add(SOFT);
}
return createCommand(CLUSTER, new StatusOutput<>(codec), args);
}
Command clusterSaveconfig() {
CommandArgs args = new CommandArgs<>(codec).add(SAVECONFIG);
return createCommand(CLUSTER, new StatusOutput<>(codec), args);
}
Command clusterSetConfigEpoch(long configEpoch) {
CommandArgs args = new CommandArgs<>(codec).add("SET-CONFIG-EPOCH").add(configEpoch);
return createCommand(CLUSTER, new StatusOutput<>(codec), args);
}
Command clusterSetSlotImporting(int slot, String nodeId) {
assertNodeId(nodeId);
CommandArgs args = new CommandArgs<>(codec).add(SETSLOT).add(slot).add(IMPORTING).add(nodeId);
return createCommand(CLUSTER, new StatusOutput<>(codec), args);
}
Command clusterSetSlotMigrating(int slot, String nodeId) {
assertNodeId(nodeId);
CommandArgs args = new CommandArgs<>(codec).add(SETSLOT).add(slot).add(MIGRATING).add(nodeId);
return createCommand(CLUSTER, new StatusOutput<>(codec), args);
}
Command clusterSetSlotNode(int slot, String nodeId) {
assertNodeId(nodeId);
CommandArgs args = new CommandArgs<>(codec).add(SETSLOT).add(slot).add(NODE).add(nodeId);
return createCommand(CLUSTER, new StatusOutput<>(codec), args);
}
Command clusterSetSlotStable(int slot) {
CommandArgs args = new CommandArgs<>(codec).add(SETSLOT).add(slot).add(STABLE);
return createCommand(CLUSTER, new StatusOutput<>(codec), args);
}
Command> clusterShards() {
CommandArgs args = new CommandArgs<>(codec).add(SHARDS);
return createCommand(CLUSTER, new ArrayOutput<>(codec), args);
}
Command> clusterSlaves(String nodeId) {
assertNodeId(nodeId);
CommandArgs args = new CommandArgs<>(codec).add(SLAVES).add(nodeId);
return createCommand(CLUSTER, new StringListOutput<>(codec), args);
}
Command> clusterSlots() {
CommandArgs args = new CommandArgs<>(codec).add(SLOTS);
return createCommand(CLUSTER, new ArrayOutput<>(codec), args);
}
Command> command() {
CommandArgs args = new CommandArgs<>(StringCodec.UTF8);
return Command.class.cast(new Command(COMMAND, new ArrayOutput<>(StringCodec.UTF8), args));
}
Command commandCount() {
CommandArgs args = new CommandArgs<>(codec).add(COUNT);
return createCommand(COMMAND, new IntegerOutput<>(codec), args);
}
Command> commandInfo(String... commands) {
LettuceAssert.notNull(commands, "Commands " + MUST_NOT_BE_NULL);
LettuceAssert.notEmpty(commands, "Commands " + MUST_NOT_BE_EMPTY);
LettuceAssert.noNullElements(commands, "Commands " + MUST_NOT_CONTAIN_NULL_ELEMENTS);
CommandArgs args = new CommandArgs<>(StringCodec.UTF8);
args.add(CommandKeyword.INFO);
for (String command : commands) {
args.add(command);
}
return Command.class.cast(new Command<>(COMMAND, new ArrayOutput<>(StringCodec.UTF8), args));
}
Command> configGet(String parameter) {
LettuceAssert.notNull(parameter, "Parameter " + MUST_NOT_BE_NULL);
LettuceAssert.notEmpty(parameter, "Parameter " + MUST_NOT_BE_EMPTY);
CommandArgs args = new CommandArgs<>(StringCodec.UTF8).add(GET).add(parameter);
return Command.class.cast(new Command<>(CONFIG, new MapOutput<>(StringCodec.UTF8), args));
}
Command> configGet(String... parameters) {
LettuceAssert.notNull(parameters, "Parameters " + MUST_NOT_BE_NULL);
LettuceAssert.notEmpty(parameters, "Parameters " + MUST_NOT_BE_EMPTY);
CommandArgs args = new CommandArgs<>(StringCodec.UTF8).add(GET);
for (String parameter : parameters) {
args.add(parameter);
}
return Command.class.cast(new Command<>(CONFIG, new MapOutput<>(StringCodec.UTF8), args));
}
Command configResetstat() {
CommandArgs args = new CommandArgs<>(codec).add(RESETSTAT);
return createCommand(CONFIG, new StatusOutput<>(codec), args);
}
Command configRewrite() {
CommandArgs args = new CommandArgs<>(codec).add(REWRITE);
return createCommand(CONFIG, new StatusOutput<>(codec), args);
}
Command configSet(String parameter, String value) {
LettuceAssert.notNull(parameter, "Parameter " + MUST_NOT_BE_NULL);
LettuceAssert.notEmpty(parameter, "Parameter " + MUST_NOT_BE_EMPTY);
LettuceAssert.notNull(value, "Value " + MUST_NOT_BE_NULL);
CommandArgs args = new CommandArgs<>(codec).add(SET).add(parameter).add(value);
return createCommand(CONFIG, new StatusOutput<>(codec), args);
}
Command configSet(Map configValues) {
LettuceAssert.notNull(configValues, "ConfigValues " + MUST_NOT_BE_NULL);
LettuceAssert.isTrue(!configValues.isEmpty(), "ConfigValues " + MUST_NOT_BE_EMPTY);
CommandArgs args = new CommandArgs<>(codec).add(SET);
configValues.forEach((parameter, value) -> {
args.add(parameter);
args.add(value);
});
return createCommand(CONFIG, new StatusOutput<>(codec), args);
}
Command dbsize() {
return createCommand(DBSIZE, new IntegerOutput<>(codec));
}
Command debugCrashAndRecover(Long delay) {
CommandArgs args = new CommandArgs<>(codec).add("CRASH-AND-RECOVER");
if (delay != null) {
args.add(delay);
}
return createCommand(DEBUG, new StatusOutput<>(codec), args);
}
Command debugHtstats(int db) {
CommandArgs args = new CommandArgs<>(codec).add(HTSTATS).add(db);
return createCommand(DEBUG, new StatusOutput<>(codec), args);
}
Command debugObject(K key) {
notNullKey(key);
CommandArgs args = new CommandArgs<>(codec).add(OBJECT).addKey(key);
return createCommand(DEBUG, new StatusOutput<>(codec), args);
}
Command debugOom() {
return createCommand(DEBUG, null, new CommandArgs<>(codec).add("OOM"));
}
Command debugReload() {
return createCommand(DEBUG, new StatusOutput<>(codec), new CommandArgs<>(codec).add(RELOAD));
}
Command debugRestart(Long delay) {
CommandArgs args = new CommandArgs<>(codec).add(RESTART);
if (delay != null) {
args.add(delay);
}
return createCommand(DEBUG, new StatusOutput<>(codec), args);
}
Command debugSdslen(K key) {
notNullKey(key);
return createCommand(DEBUG, new StatusOutput<>(codec), new CommandArgs<>(codec).add("SDSLEN").addKey(key));
}
Command debugSegfault() {
CommandArgs args = new CommandArgs<>(codec).add(SEGFAULT);
return createCommand(DEBUG, null, args);
}
Command decr(K key) {
notNullKey(key);
return createCommand(DECR, new IntegerOutput<>(codec), key);
}
Command decrby(K key, long amount) {
notNullKey(key);
CommandArgs args = new CommandArgs<>(codec).addKey(key).add(amount);
return createCommand(DECRBY, new IntegerOutput<>(codec), args);
}
Command del(K... keys) {
notEmpty(keys);
CommandArgs args = new CommandArgs<>(codec).addKeys(keys);
return createCommand(DEL, new IntegerOutput<>(codec), args);
}
Command del(Iterable keys) {
LettuceAssert.notNull(keys, "Keys " + MUST_NOT_BE_NULL);
CommandArgs args = new CommandArgs<>(codec).addKeys(keys);
return createCommand(DEL, new IntegerOutput<>(codec), args);
}
Command discard() {
return createCommand(DISCARD, new StatusOutput<>(codec));
}
Command dump(K key) {
notNullKey(key);
CommandArgs args = new CommandArgs<>(codec).addKey(key);
return createCommand(DUMP, new ByteArrayOutput<>(codec), args);
}
Command echo(V msg) {
LettuceAssert.notNull(msg, "message " + MUST_NOT_BE_NULL);
CommandArgs args = new CommandArgs<>(codec).addValue(msg);
return createCommand(ECHO, new ValueOutput<>(codec), args);
}
Command eval(byte[] script, ScriptOutputType type, K[] keys, V... values) {
return eval(script, type, false, keys, values);
}
Command eval(byte[] script, ScriptOutputType type, boolean readonly, K[] keys, V... values) {
LettuceAssert.notNull(script, "Script " + MUST_NOT_BE_NULL);
LettuceAssert.notNull(type, "ScriptOutputType " + MUST_NOT_BE_NULL);
LettuceAssert.notNull(keys, "Keys " + MUST_NOT_BE_NULL);
LettuceAssert.notNull(values, "Values " + MUST_NOT_BE_NULL);
CommandArgs args = new CommandArgs<>(codec);
args.add(script).add(keys.length).addKeys(keys).addValues(values);
CommandOutput output = newScriptOutput(codec, type);
return createCommand(readonly ? EVAL_RO : EVAL, output, args);
}
Command evalsha(String digest, ScriptOutputType type, K[] keys, V... values) {
return evalsha(digest, type, false, keys, values);
}
Command evalsha(String digest, ScriptOutputType type, boolean readonly, K[] keys, V... values) {
LettuceAssert.notNull(digest, "Digest " + MUST_NOT_BE_NULL);
LettuceAssert.notEmpty(digest, "Digest " + MUST_NOT_BE_EMPTY);
LettuceAssert.notNull(type, "ScriptOutputType " + MUST_NOT_BE_NULL);
LettuceAssert.notNull(keys, "Keys " + MUST_NOT_BE_NULL);
LettuceAssert.notNull(values, "Values " + MUST_NOT_BE_NULL);
CommandArgs args = new CommandArgs<>(codec);
args.add(digest).add(keys.length).addKeys(keys).addValues(values);
CommandOutput output = newScriptOutput(codec, type);
return createCommand(readonly ? EVALSHA_RO : EVALSHA, output, args);
}
Command exists(K key) {
notNullKey(key);
return createCommand(EXISTS, new BooleanOutput<>(codec), key);
}
Command exists(K... keys) {
notEmpty(keys);
return createCommand(EXISTS, new IntegerOutput<>(codec), new CommandArgs<>(codec).addKeys(keys));
}
Command exists(Iterable keys) {
LettuceAssert.notNull(keys, "Keys " + MUST_NOT_BE_NULL);
return createCommand(EXISTS, new IntegerOutput<>(codec), new CommandArgs<>(codec).addKeys(keys));
}
Command