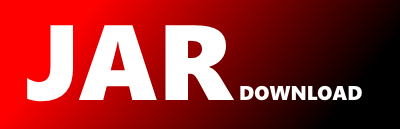
io.lettuce.core.ScanFlow.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lettuce-core Show documentation
Show all versions of lettuce-core Show documentation
Advanced and thread-safe Java Redis client for synchronous, asynchronous, and
reactive usage. Supports Cluster, Sentinel, Pipelining, Auto-Reconnect, Codecs
and much more.
The newest version!
/*
* Copyright 2020-Present, Redis Ltd. and Contributors
* All rights reserved.
*
* Licensed under the MIT License.
*
* This file contains contributions from third-party contributors
* licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.lettuce.core
import io.lettuce.core.api.coroutines.*
import io.lettuce.core.cluster.api.coroutines.RedisClusterCoroutinesCommandsImpl
import kotlinx.coroutines.flow.Flow
import kotlinx.coroutines.reactive.asFlow
/**
* Coroutines adapter for [ScanStream].
*
* @author Mikhael Sokolov
* @author Mark Paluch
* @since 6.1
*/
@ExperimentalLettuceCoroutinesApi
object ScanFlow {
/**
* Sequentially iterate the keys space.
*
* @param commands coroutines commands
* @param scanArgs scan arguments.
* @return `Flow` flow of keys.
*/
fun scan(commands: RedisKeyCoroutinesCommands, scanArgs: ScanArgs? = null): Flow {
val ops = when (commands) {
is RedisCoroutinesCommandsImpl -> commands.ops
is RedisClusterCoroutinesCommandsImpl -> commands.ops
is RedisKeyCoroutinesCommandsImpl -> commands.ops
else -> throw IllegalArgumentException("Cannot access underlying reactive API")
}
return when (scanArgs) {
null -> ScanStream.scan(ops)
else -> ScanStream.scan(ops, scanArgs)
}.asFlow()
}
/**
* Sequentially iterate hash fields and associated values.
*
* @param commands coroutines commands
* @param key the key.
* @param scanArgs scan arguments.
* @return `Flow>` flow of key-values.
*/
fun hscan(commands: RedisHashCoroutinesCommands, key: K, scanArgs: ScanArgs? = null): Flow> {
val ops = when (commands) {
is RedisCoroutinesCommandsImpl -> commands.ops
is RedisClusterCoroutinesCommandsImpl -> commands.ops
is RedisHashCoroutinesCommandsImpl -> commands.ops
else -> throw IllegalArgumentException("Cannot access underlying reactive API")
}
return when (scanArgs) {
null -> ScanStream.hscan(ops, key)
else -> ScanStream.hscan(ops, key, scanArgs)
}.asFlow()
}
/**
* Sequentially iterate hash key, without associated values.
*
* @param commands coroutines commands
* @param key the key.
* @param scanArgs scan arguments.
* @return `Flow>` flow of key-values.
* @since 6.4
*/
fun hscanNovalues(commands: RedisHashCoroutinesCommands, key: K, scanArgs: ScanArgs? = null): Flow {
val ops = when (commands) {
is RedisCoroutinesCommandsImpl -> commands.ops
is RedisClusterCoroutinesCommandsImpl -> commands.ops
is RedisHashCoroutinesCommandsImpl -> commands.ops
else -> throw IllegalArgumentException("Cannot access underlying reactive API")
}
return when (scanArgs) {
null -> ScanStream.hscanNovalues(ops, key)
else -> ScanStream.hscanNovalues(ops, key, scanArgs)
}.asFlow()
}
/**
* Sequentially iterate Set elements.
*
* @param commands coroutines commands
* @param key the key.
* @param scanArgs scan arguments.
* @return `Flow` flow of value.
*/
fun sscan(commands: RedisSetCoroutinesCommands, key: K, scanArgs: ScanArgs? = null): Flow {
val ops = when (commands) {
is RedisCoroutinesCommandsImpl -> commands.ops
is RedisClusterCoroutinesCommandsImpl -> commands.ops
is RedisSetCoroutinesCommandsImpl -> commands.ops
else -> throw IllegalArgumentException("Cannot access underlying reactive API")
}
return when (scanArgs) {
null -> ScanStream.sscan(ops, key)
else -> ScanStream.sscan(ops, key, scanArgs)
}.asFlow()
}
/**
* Sequentially iterate Sorted Set elements.
*
* @param commands coroutines commands
* @param key the key.
* @param scanArgs scan arguments.
* @return `Flow` flow of [ScoredValue].
*/
fun zscan(commands: RedisSortedSetCoroutinesCommands, key: K, scanArgs: ScanArgs? = null): Flow> {
val ops = when (commands) {
is RedisCoroutinesCommandsImpl -> commands.ops
is RedisClusterCoroutinesCommandsImpl -> commands.ops
is RedisSortedSetCoroutinesCommandsImpl -> commands.ops
else -> throw IllegalArgumentException("Cannot access underlying reactive API")
}
return when (scanArgs) {
null -> ScanStream.zscan(ops, key)
else -> ScanStream.zscan(ops, key, scanArgs)
}.asFlow()
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy