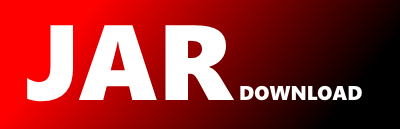
io.lettuce.core.cluster.ClusterReadOnlyCommands Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lettuce-core Show documentation
Show all versions of lettuce-core Show documentation
Advanced and thread-safe Java Redis client for synchronous, asynchronous, and
reactive usage. Supports Cluster, Sentinel, Pipelining, Auto-Reconnect, Codecs
and much more.
The newest version!
package io.lettuce.core.cluster;
import java.util.Collections;
import java.util.EnumSet;
import java.util.Set;
import io.lettuce.core.protocol.CommandType;
import io.lettuce.core.protocol.ProtocolKeyword;
import io.lettuce.core.protocol.ReadOnlyCommands;
/**
* Contains all command names that are read-only commands.
*
* @author Mark Paluch
* @since 6.2.5
*/
public class ClusterReadOnlyCommands {
private static final Set READ_ONLY_COMMANDS = EnumSet.noneOf(CommandType.class);
private static final ReadOnlyCommands.ReadOnlyPredicate PREDICATE = command -> isReadOnlyCommand(command.getType());
static {
READ_ONLY_COMMANDS.addAll(ReadOnlyCommands.getReadOnlyCommands());
for (CommandName commandNames : CommandName.values()) {
READ_ONLY_COMMANDS.add(CommandType.valueOf(commandNames.name()));
}
}
/**
* @param protocolKeyword must not be {@code null}.
* @return {@code true} if {@link ProtocolKeyword} is a read-only command.
*/
public static boolean isReadOnlyCommand(ProtocolKeyword protocolKeyword) {
return READ_ONLY_COMMANDS.contains(protocolKeyword);
}
/**
* @return an unmodifiable {@link Set} of {@link CommandType read-only} commands.
*/
public static Set getReadOnlyCommands() {
return Collections.unmodifiableSet(READ_ONLY_COMMANDS);
}
/**
* Return a {@link ReadOnlyCommands.ReadOnlyPredicate} to test against the underlying
* {@link #isReadOnlyCommand(ProtocolKeyword) known commands}.
*
* @return a {@link ReadOnlyCommands.ReadOnlyPredicate} to test against the underlying
* {@link #isReadOnlyCommand(ProtocolKeyword) known commands}.
*/
public static ReadOnlyCommands.ReadOnlyPredicate asPredicate() {
return PREDICATE;
}
enum CommandName {
// Pub/Sub commands are no key-space commands so they are safe to execute on replica nodes
PUBLISH, PUBSUB, PSUBSCRIBE, PUNSUBSCRIBE, SUBSCRIBE, UNSUBSCRIBE, SSUBSCRIBE
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy