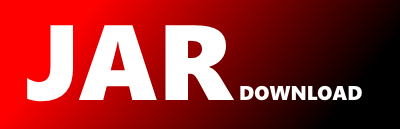
io.lettuce.core.cluster.RedisClusterPubSubReactiveCommandsImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lettuce-core Show documentation
Show all versions of lettuce-core Show documentation
Advanced and thread-safe Java Redis client for synchronous, asynchronous, and
reactive usage. Supports Cluster, Sentinel, Pipelining, Auto-Reconnect, Codecs
and much more.
The newest version!
/*
* Copyright 2016-Present, Redis Ltd. and Contributors
* All rights reserved.
*
* Licensed under the MIT License.
*
* This file contains contributions from third-party contributors
* licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.lettuce.core.cluster;
import static io.lettuce.core.cluster.NodeSelectionInvocationHandler.ExecutionModel.*;
import java.lang.reflect.Proxy;
import java.util.List;
import java.util.concurrent.CompletableFuture;
import java.util.function.Predicate;
import java.util.stream.Collectors;
import reactor.core.publisher.Flux;
import io.lettuce.core.GeoArgs;
import io.lettuce.core.GeoWithin;
import io.lettuce.core.RedisURI;
import io.lettuce.core.cluster.api.NodeSelectionSupport;
import io.lettuce.core.cluster.models.partitions.RedisClusterNode;
import io.lettuce.core.cluster.pubsub.StatefulRedisClusterPubSubConnection;
import io.lettuce.core.cluster.pubsub.api.reactive.NodeSelectionPubSubReactiveCommands;
import io.lettuce.core.cluster.pubsub.api.reactive.PubSubReactiveNodeSelection;
import io.lettuce.core.cluster.pubsub.api.reactive.RedisClusterPubSubReactiveCommands;
import io.lettuce.core.codec.RedisCodec;
import io.lettuce.core.protocol.ConnectionIntent;
import io.lettuce.core.pubsub.RedisPubSubReactiveCommandsImpl;
import io.lettuce.core.pubsub.StatefulRedisPubSubConnection;
import io.lettuce.core.pubsub.api.reactive.RedisPubSubReactiveCommands;
/**
* A reactive and thread-safe API for a Redis pub/sub connection.
*
* @param Key type.
* @param Value type.
* @author Mark Paluch
* @since 5.0
*/
public class RedisClusterPubSubReactiveCommandsImpl extends RedisPubSubReactiveCommandsImpl
implements RedisClusterPubSubReactiveCommands {
/**
* Initialize a new connection.
*
* @param connection the connection.
* @param codec Codec used to encode/decode keys and values.
*/
public RedisClusterPubSubReactiveCommandsImpl(StatefulRedisPubSubConnection connection, RedisCodec codec) {
super(connection, codec);
}
@Override
public Flux georadius(K key, double longitude, double latitude, double distance, GeoArgs.Unit unit) {
return super.georadius_ro(key, longitude, latitude, distance, unit);
}
@Override
public Flux> georadius(K key, double longitude, double latitude, double distance, GeoArgs.Unit unit,
GeoArgs geoArgs) {
return super.georadius_ro(key, longitude, latitude, distance, unit, geoArgs);
}
@Override
public Flux georadiusbymember(K key, V member, double distance, GeoArgs.Unit unit) {
return super.georadiusbymember_ro(key, member, distance, unit);
}
@Override
public Flux> georadiusbymember(K key, V member, double distance, GeoArgs.Unit unit, GeoArgs geoArgs) {
return super.georadiusbymember_ro(key, member, distance, unit, geoArgs);
}
@Override
public StatefulRedisClusterPubSubConnectionImpl getStatefulConnection() {
return (StatefulRedisClusterPubSubConnectionImpl) super.getStatefulConnection();
}
@SuppressWarnings("unchecked")
@Override
public PubSubReactiveNodeSelection nodes(Predicate predicate) {
PubSubReactiveNodeSelection selection = new StaticPubSubReactiveNodeSelection(getStatefulConnection(),
predicate);
NodeSelectionInvocationHandler h = new NodeSelectionInvocationHandler((AbstractNodeSelection, ?, ?, ?>) selection,
RedisPubSubReactiveCommands.class, REACTIVE);
return (PubSubReactiveNodeSelection) Proxy.newProxyInstance(NodeSelectionSupport.class.getClassLoader(),
new Class>[] { NodeSelectionPubSubReactiveCommands.class, PubSubReactiveNodeSelection.class }, h);
}
private static class StaticPubSubReactiveNodeSelection
extends AbstractNodeSelection, NodeSelectionPubSubReactiveCommands, K, V>
implements PubSubReactiveNodeSelection {
private final List redisClusterNodes;
private final ClusterDistributionChannelWriter writer;
@SuppressWarnings("unchecked")
public StaticPubSubReactiveNodeSelection(StatefulRedisClusterPubSubConnection globalConnection,
Predicate selector) {
this.redisClusterNodes = globalConnection.getPartitions().stream().filter(selector).collect(Collectors.toList());
writer = ((StatefulRedisClusterPubSubConnectionImpl) globalConnection).getClusterDistributionChannelWriter();
}
@Override
protected CompletableFuture> getApi(RedisClusterNode redisClusterNode) {
return getConnection(redisClusterNode).thenApply(StatefulRedisPubSubConnection::reactive);
}
protected List nodes() {
return redisClusterNodes;
}
@SuppressWarnings("unchecked")
protected CompletableFuture> getConnection(RedisClusterNode redisClusterNode) {
RedisURI uri = redisClusterNode.getUri();
AsyncClusterConnectionProvider async = (AsyncClusterConnectionProvider) writer.getClusterConnectionProvider();
return async.getConnectionAsync(ConnectionIntent.WRITE, uri.getHost(), uri.getPort())
.thenApply(it -> (StatefulRedisPubSubConnection) it);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy