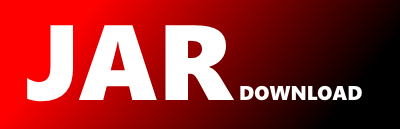
io.lettuce.core.codec.CompressionCodec Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lettuce-core Show documentation
Show all versions of lettuce-core Show documentation
Advanced and thread-safe Java Redis client for synchronous, asynchronous, and
reactive usage. Supports Cluster, Sentinel, Pipelining, Auto-Reconnect, Codecs
and much more.
The newest version!
package io.lettuce.core.codec;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.nio.ByteBuffer;
import java.util.zip.DeflaterOutputStream;
import java.util.zip.GZIPInputStream;
import java.util.zip.GZIPOutputStream;
import java.util.zip.InflaterInputStream;
import io.lettuce.core.internal.LettuceAssert;
/**
* A compressing/decompressing {@link RedisCodec} that wraps a typed {@link RedisCodec codec} and compresses values using GZIP
* or Deflate. See {@link io.lettuce.core.codec.CompressionCodec.CompressionType} for supported compression types.
*
* @author Mark Paluch
*/
public abstract class CompressionCodec {
private CompressionCodec() {
}
/**
* A {@link RedisCodec} that compresses values from a delegating {@link RedisCodec}.
*
* @param delegate codec used for key-value encoding/decoding, must not be {@code null}.
* @param compressionType the compression type, must not be {@code null}.
* @param Key type.
* @param Value type.
* @return Value-compressing codec.
*/
@SuppressWarnings({ "rawtypes", "unchecked" })
public static RedisCodec valueCompressor(RedisCodec delegate, CompressionType compressionType) {
LettuceAssert.notNull(delegate, "RedisCodec must not be null");
LettuceAssert.notNull(compressionType, "CompressionType must not be null");
return (RedisCodec) new CompressingValueCodecWrapper((RedisCodec) delegate, compressionType);
}
private static class CompressingValueCodecWrapper implements RedisCodec
© 2015 - 2025 Weber Informatics LLC | Privacy Policy