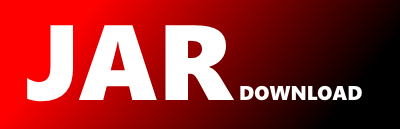
io.lettuce.core.codec.RedisCodec Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lettuce-core Show documentation
Show all versions of lettuce-core Show documentation
Advanced and thread-safe Java Redis client for synchronous, asynchronous, and
reactive usage. Supports Cluster, Sentinel, Pipelining, Auto-Reconnect, Codecs
and much more.
The newest version!
package io.lettuce.core.codec;
import java.nio.ByteBuffer;
/**
* A {@link RedisCodec} encodes keys and values sent to Redis, and decodes keys and values in the command output.
*
* The methods are called by multiple threads and must be thread-safe.
*
* @param Key type.
* @param Value type.
*
* @author Will Glozer
* @author Mark Paluch
* @author Dimitris Mandalidis
*/
public interface RedisCodec {
/**
* Returns a composite {@link RedisCodec} that uses {@code keyCodec} for keys and {@code valueCodec} for values.
*
* @param the type of the key
* @param the type of the value
* @param keyCodec the codec to encode/decode the keys.
* @param valueCodec the codec to encode/decode the values.
* @return a composite {@link RedisCodec}.
* @since 5.2
*/
static RedisCodec of(RedisCodec keyCodec, RedisCodec, V> valueCodec) {
return new ComposedRedisCodec<>(keyCodec, valueCodec);
}
/**
* Decode the key output by redis.
*
* @param bytes Raw bytes of the key, must not be {@code null}.
*
* @return The decoded key, may be {@code null}.
*/
K decodeKey(ByteBuffer bytes);
/**
* Decode the value output by redis.
*
* @param bytes Raw bytes of the value, must not be {@code null}.
*
* @return The decoded value, may be {@code null}.
*/
V decodeValue(ByteBuffer bytes);
/**
* Encode the key for output to redis.
*
* @param key the key, may be {@code null}.
*
* @return The encoded key, never {@code null}.
*/
ByteBuffer encodeKey(K key);
/**
* Encode the value for output to redis.
*
* @param value the value, may be {@code null}.
*
* @return The encoded value, never {@code null}.
*/
ByteBuffer encodeValue(V value);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy