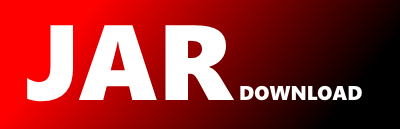
io.lettuce.core.dynamic.AsyncExecutableCommand Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lettuce-core Show documentation
Show all versions of lettuce-core Show documentation
Advanced and thread-safe Java Redis client for synchronous, asynchronous, and
reactive usage. Supports Cluster, Sentinel, Pipelining, Auto-Reconnect, Codecs
and much more.
The newest version!
package io.lettuce.core.dynamic;
import java.time.Duration;
import java.util.concurrent.ExecutionException;
import io.lettuce.core.api.StatefulConnection;
import io.lettuce.core.dynamic.domain.Timeout;
import io.lettuce.core.dynamic.parameter.ExecutionSpecificParameters;
import io.lettuce.core.internal.Futures;
import io.lettuce.core.protocol.AsyncCommand;
import io.lettuce.core.protocol.RedisCommand;
/**
* An {@link ExecutableCommand} that is executed asynchronously or synchronously.
*
* @author Mark Paluch
* @since 5.0
*/
class AsyncExecutableCommand implements ExecutableCommand {
private final CommandMethod commandMethod;
private final CommandFactory commandFactory;
private final StatefulConnection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy