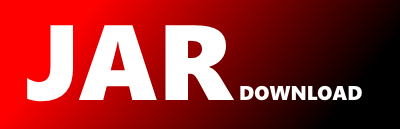
io.lettuce.core.dynamic.BatchExecutableCommandLookupStrategy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lettuce-core Show documentation
Show all versions of lettuce-core Show documentation
Advanced and thread-safe Java Redis client for synchronous, asynchronous, and
reactive usage. Supports Cluster, Sentinel, Pipelining, Auto-Reconnect, Codecs
and much more.
The newest version!
package io.lettuce.core.dynamic;
import java.util.Arrays;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import java.util.concurrent.ExecutionException;
import io.lettuce.core.api.StatefulConnection;
import io.lettuce.core.codec.RedisCodec;
import io.lettuce.core.dynamic.batch.BatchExecutor;
import io.lettuce.core.dynamic.output.CommandOutputFactoryResolver;
import io.lettuce.core.dynamic.parameter.ExecutionSpecificParameters;
import io.lettuce.core.internal.LettuceAssert;
/**
* @author Mark Paluch
* @since 5.0
*/
class BatchExecutableCommandLookupStrategy extends ExecutableCommandLookupStrategySupport {
private final Set> SYNCHRONOUS_RETURN_TYPES = new HashSet>(Arrays.asList(Void.class, Void.TYPE));
private final Batcher batcher;
private final StatefulConnection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy