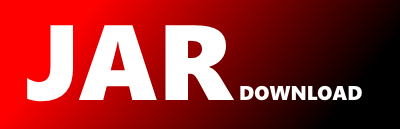
io.lettuce.core.dynamic.ConversionService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lettuce-core Show documentation
Show all versions of lettuce-core Show documentation
Advanced and thread-safe Java Redis client for synchronous, asynchronous, and
reactive usage. Supports Cluster, Sentinel, Pipelining, Auto-Reconnect, Codecs
and much more.
The newest version!
package io.lettuce.core.dynamic;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import java.util.function.Function;
import io.lettuce.core.dynamic.support.ClassTypeInformation;
import io.lettuce.core.dynamic.support.TypeInformation;
import io.lettuce.core.internal.LettuceAssert;
/**
* @author Mark Paluch
*/
class ConversionService {
private Map> converterMap = new HashMap<>(10);
/**
* Register a converter {@link Function}.
*
* @param converter the converter.
*/
@SuppressWarnings("rawtypes")
public void addConverter(Function, ?> converter) {
LettuceAssert.notNull(converter, "Converter must not be null");
ClassTypeInformation extends Function> classTypeInformation = ClassTypeInformation.from(converter.getClass());
TypeInformation> typeInformation = classTypeInformation.getSuperTypeInformation(Function.class);
List> typeArguments = typeInformation.getTypeArguments();
ConvertiblePair pair = new ConvertiblePair(typeArguments.get(0).getType(), typeArguments.get(1).getType());
converterMap.put(pair, converter);
}
@SuppressWarnings("unchecked")
public T convert(S source, Class targetType) {
LettuceAssert.notNull(source, "Source must not be null");
return (T) getConverter(source.getClass(), targetType).apply(source);
}
public boolean canConvert(Class sourceType, Class targetType) {
return findConverter(sourceType, targetType).isPresent();
}
@SuppressWarnings("unchecked")
Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy