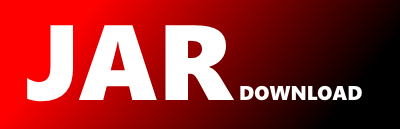
io.lettuce.core.dynamic.ParameterBinder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lettuce-core Show documentation
Show all versions of lettuce-core Show documentation
Advanced and thread-safe Java Redis client for synchronous, asynchronous, and
reactive usage. Supports Cluster, Sentinel, Pipelining, Auto-Reconnect, Codecs
and much more.
The newest version!
package io.lettuce.core.dynamic;
import static io.lettuce.core.protocol.CommandKeyword.LIMIT;
import java.lang.reflect.Array;
import java.nio.ByteBuffer;
import java.util.*;
import io.lettuce.core.*;
import io.lettuce.core.codec.RedisCodec;
import io.lettuce.core.dynamic.parameter.MethodParametersAccessor;
import io.lettuce.core.dynamic.segment.CommandSegment;
import io.lettuce.core.dynamic.segment.CommandSegments;
import io.lettuce.core.dynamic.segment.CommandSegment.ArgumentContribution;
import io.lettuce.core.protocol.CommandArgs;
import io.lettuce.core.protocol.ProtocolKeyword;
/**
* Parameter binder for {@link CommandSegments}-based Redis Commands.
*
* @author Mark Paluch
* @since 5.0
*/
class ParameterBinder {
private static final byte[] MINUS_BYTES = { '-' };
private static final byte[] PLUS_BYTES = { '+' };
/**
* Bind {@link CommandSegments} and method parameters to {@link CommandArgs}.
*
* @param args the command arguments.
* @param codec the codec.
* @param commandSegments the command segments.
* @param accessor the parameter accessor.
* @return
*/
CommandArgs bind(CommandArgs args, RedisCodec codec, CommandSegments commandSegments,
MethodParametersAccessor accessor) {
int parameterCount = accessor.getParameterCount();
BitSet set = new BitSet(parameterCount);
for (CommandSegment commandSegment : commandSegments) {
ArgumentContribution argumentContribution = commandSegment.contribute(accessor);
bind(args, codec, argumentContribution.getValue(), argumentContribution.getParameterIndex(), accessor);
if (argumentContribution.getParameterIndex() != -1) {
set.set(argumentContribution.getParameterIndex());
}
}
for (int i = 0; i < parameterCount; i++) {
if (set.get(i)) {
continue;
}
Object bindableValue = accessor.getBindableValue(i);
bind(args, codec, bindableValue, i, accessor);
set.set(i);
}
return args;
}
/*
* Bind key/value/byte[] arguments. Other arguments are unwound, if applicable, and bound according to their type.
*/
@SuppressWarnings("unchecked")
private void bind(CommandArgs args, RedisCodec codec, Object argument, int index,
MethodParametersAccessor accessor) {
if (argument == null) {
if (accessor.isBindableNullValue(index)) {
args.add(new byte[0]);
}
return;
}
if (argument instanceof byte[]) {
if (index != -1 && accessor.isKey(index)) {
args.addKey((K) argument);
} else {
args.add((byte[]) argument);
}
return;
}
if (argument.getClass().isArray()) {
argument = asIterable(argument);
}
if (index != -1) {
if (accessor.isKey(index)) {
if (argument instanceof Iterable) {
args.addKeys((Iterable) argument);
} else {
args.addKey((K) argument);
}
return;
}
if (accessor.isValue(index)) {
if (argument instanceof Range) {
bindValueRange(args, codec, (Range extends V>) argument);
return;
}
if (argument instanceof Iterable) {
args.addValues((Iterable) argument);
} else {
args.addValue((V) argument);
}
return;
}
}
if (argument instanceof Iterable) {
for (Object argumentElement : (Iterable
© 2015 - 2025 Weber Informatics LLC | Privacy Policy