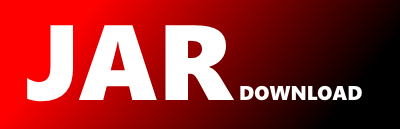
io.lettuce.core.json.DelegateJsonArray Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lettuce-core Show documentation
Show all versions of lettuce-core Show documentation
Advanced and thread-safe Java Redis client for synchronous, asynchronous, and
reactive usage. Supports Cluster, Sentinel, Pipelining, Auto-Reconnect, Codecs
and much more.
The newest version!
/*
* Copyright 2024, Redis Ltd. and Contributors
* All rights reserved.
*
* Licensed under the MIT License.
*/
package io.lettuce.core.json;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.node.ArrayNode;
import com.fasterxml.jackson.databind.node.JsonNodeFactory;
import io.lettuce.core.internal.LettuceAssert;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
/**
* Implementation of the {@link DelegateJsonArray} that delegates most of its' functionality to the Jackson {@link ArrayNode}.
*
* @author Tihomir Mateev
*/
class DelegateJsonArray extends DelegateJsonValue implements JsonArray {
DelegateJsonArray() {
super(new ArrayNode(JsonNodeFactory.instance));
}
DelegateJsonArray(JsonNode node) {
super(node);
}
@Override
public JsonArray add(JsonValue element) {
JsonNode newNode = null;
if (element != null) {
newNode = ((DelegateJsonValue) element).getNode();
}
((ArrayNode) node).add(newNode);
return this;
}
@Override
public void addAll(JsonArray element) {
LettuceAssert.notNull(element, "Element must not be null");
ArrayNode otherArray = (ArrayNode) ((DelegateJsonValue) element).getNode();
((ArrayNode) node).addAll(otherArray);
}
@Override
public List asList() {
List result = new ArrayList<>();
for (JsonNode jsonNode : node) {
result.add(new DelegateJsonValue(jsonNode));
}
return result;
}
@Override
public JsonValue get(int index) {
JsonNode jsonNode = node.get(index);
return jsonNode == null ? null : wrap(jsonNode);
}
@Override
public JsonValue getFirst() {
return get(0);
}
@Override
public Iterator iterator() {
return asList().iterator();
}
@Override
public JsonValue remove(int index) {
JsonNode jsonNode = ((ArrayNode) node).remove(index);
return wrap(jsonNode);
}
@Override
public JsonValue replace(int index, JsonValue newElement) {
JsonNode replaceWith = ((DelegateJsonValue) newElement).getNode();
JsonNode replaced = ((ArrayNode) node).set(index, replaceWith);
return wrap(replaced);
}
@Override
public int size() {
return node.size();
}
@Override
public JsonArray asJsonArray() {
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy