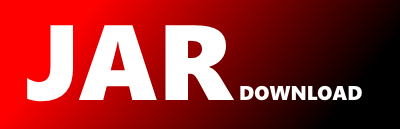
io.lettuce.core.json.JsonObject Maven / Gradle / Ivy
Show all versions of lettuce-core Show documentation
/*
* Copyright 2024, Redis Ltd. and Contributors
* All rights reserved.
*
* Licensed under the MIT License.
*/
package io.lettuce.core.json;
/**
* Representation of a JSON object as per RFC 8259 - The
* JavaScript Object Notation (JSON) Data Interchange Format, Section 4. Objects
*
*
* @see JsonValue
* @author Tihomir Mateev
* @since 6.5
*/
public interface JsonObject extends JsonValue {
/**
* Add (if there is no value with the same key already) or replace (if there is) a new {@link JsonValue} to the object under
* the provided key. Supports chaining of calls.
*
* @param key the key of the {@link JsonValue} to add or replace
* @param element the value to add or replace
* @return the updated {@link JsonObject} to allow call chaining
*/
JsonObject put(String key, JsonValue element);
/**
* Get the {@link JsonValue} under the provided key.
*
* @param key the key to get the value for
* @return the {@link JsonValue} under the provided key or {@code null} if no value is found
*/
JsonValue get(String key);
/**
* Remove the {@link JsonValue} under the provided key.
*
* @param key the key to remove the value for
* @return the removed {@link JsonValue} or {@code null} if no value is found
*/
JsonValue remove(String key);
/**
* @return the number of key-value pairs in this {@link JsonObject}
*/
int size();
}