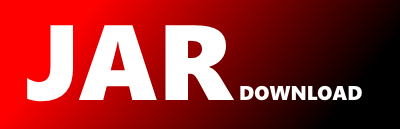
io.lettuce.core.json.JsonPath Maven / Gradle / Ivy
Show all versions of lettuce-core Show documentation
/*
* Copyright 2024, Redis Ltd. and Contributors
* All rights reserved.
*
* Licensed under the MIT License.
*/
package io.lettuce.core.json;
/**
* Describes a path to a certain {@link JsonValue} inside a JSON document.
*
*
* The Redis server implements its own JSONPath implementation, based on existing technologies. The generic rules to build a
* path string are:
*
* $
- The root (outermost JSON element), starts the path.
* . or []
- Selects a child element.
* ..
- Recursively descends through the JSON document.
* *
- Wildcard, returns all elements.
* []
- Subscript operator, accesses an array element.
* [,]]
- Union, selects multiple elements.
* [start:end:step]
- Array slice where start, end, and step are indexes.
* ?()
- Filters a JSON object or array. Supports comparison operators (==, !=, <, <=, >, >=, =~), logical
* operators (&&, ||), and parenthesis ((, )).
* ()
- Script expression.
* @
- The current element, used in filter or script expressions.
*
*
* For example, given the following JSON document:
*
*
* {
* "inventory": {
* "mountain_bikes": [
* {
* "id": "bike:1",
* "model": "Phoebe",
* "description": "This is a mid-travel trail slayer that is a fantastic daily...",
* "price": 1920,
* "specs": {"material": "carbon", "weight": 13.1},
* "colors": ["black", "silver"],
* },
* ...
* }
* }
*}
*
*
* To get a list of all the {@code mountain_bikes} inside the {@code inventory} you would write something like:
*
* {@code JSON.GET store '$.inventory["mountain_bikes"]' }
*
* @author Tihomir Mateev
* @since 6.5
* @see JSON Path in Redis docs
*/
public class JsonPath {
/**
* The root path {@code $} as defined by the second version of the RedisJSON implementation.
*
*
* @since 6.5
*/
public static final JsonPath ROOT_PATH = new JsonPath("$");
/**
* The legacy root path {@code .} as defined by the first version of the RedisJSON implementation.
*
* @deprecated since 6.5, use {@link #ROOT_PATH} instead.
*/
public static final JsonPath ROOT_PATH_LEGACY = new JsonPath(".");
private final String path;
/**
* Create a new {@link JsonPath} given a path string.
*
* @param pathString the path string, must not be {@literal null} or empty.
*/
public JsonPath(final String pathString) {
if (pathString == null) {
throw new IllegalArgumentException("Path cannot be null.");
}
if (pathString.isEmpty()) {
throw new IllegalArgumentException("Path cannot be empty.");
}
this.path = pathString;
}
@Override
public String toString() {
return path;
}
/**
* Create a new {@link JsonPath} given a path string.
*
* @param path the path string, must not be {@literal null} or empty.
* @return the {@link JsonPath}.
*/
public static JsonPath of(final String path) {
return new JsonPath(path);
}
@Override
public boolean equals(Object obj) {
return this.path.equals(obj);
}
@Override
public int hashCode() {
return path.hashCode();
}
public boolean isRootPath() {
return ROOT_PATH.toString().equals(path) || ROOT_PATH_LEGACY.toString().equals(path);
}
}