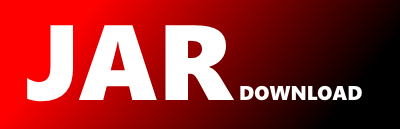
io.lettuce.core.masterslave.MasterSlave Maven / Gradle / Ivy
Show all versions of lettuce-core Show documentation
package io.lettuce.core.masterslave;
import java.util.concurrent.CompletableFuture;
import io.lettuce.core.RedisClient;
import io.lettuce.core.RedisURI;
import io.lettuce.core.codec.RedisCodec;
import io.lettuce.core.internal.LettuceAssert;
import io.lettuce.core.masterreplica.MasterReplica;
/**
* Master-Slave connection API.
*
* This API allows connections to Redis Master/Slave setups which run either in a static Master/Slave setup or are managed by
* Redis Sentinel. Master-Slave connections can discover topologies and select a source for read operations using
* {@link io.lettuce.core.ReadFrom}.
*
*
*
* Connections can be obtained by providing the {@link RedisClient}, a {@link RedisURI} and a {@link RedisCodec}.
*
*
* RedisClient client = RedisClient.create();
* StatefulRedisMasterSlaveConnection<String, String> connection = MasterSlave.connect(client,
* RedisURI.create("redis://localhost"), StringCodec.UTF8);
* // ...
*
* connection.close();
* client.shutdown();
*
*
*
* Topology Discovery
*
* Master-Slave topologies are either static or semi-static. Redis Standalone instances with attached slaves provide no
* failover/HA mechanism. Redis Sentinel managed instances are controlled by Redis Sentinel and allow failover (which include
* master promotion). The {@link MasterSlave} API supports both mechanisms. The topology is provided by a
* {@code TopologyProvider}:
*
*
* - {@code MasterReplicaTopologyProvider}: Dynamic topology lookup using the {@code INFO REPLICATION} output. Slaves are
* listed as {@code slaveN=...} entries. The initial connection can either point to a master or a replica and the topology
* provider will discover nodes. The connection needs to be re-established outside of lettuce in a case of Master/Slave failover
* or topology changes.
* - {@code StaticMasterReplicaTopologyProvider}: Topology is defined by the list of {@link RedisURI URIs} and the
* {@code ROLE} output. MasterSlave uses only the supplied nodes and won't discover additional nodes in the setup. The
* connection needs to be re-established outside of lettuce in a case of Master/Slave failover or topology changes.
* - {@code SentinelTopologyProvider}: Dynamic topology lookup using the Redis Sentinel API. In particular,
* {@code SENTINEL MASTER} and {@code SENTINEL SLAVES} output. Master/Slave failover is handled by lettuce.
*
*
* Topology Updates
*
* - Standalone Master/Slave: Performs a one-time topology lookup which remains static afterward
* - Redis Sentinel: Subscribes to all Sentinels and listens for Pub/Sub messages to trigger topology refreshing
*
*
* Connection Fault-Tolerance
Connecting to Master/Slave bears the possibility that individual nodes are not reachable.
* {@link MasterSlave} can still connect to a partially-available set of nodes.
*
*
* - Redis Sentinel: At least one Sentinel must be reachable, the masterId must be registered and at least one host must be
* available (master or slave). Allows for runtime-recovery based on Sentinel Events.
* - Static Setup (auto-discovery): The initial endpoint must be reachable. No recovery/reconfiguration during runtime.
* - Static Setup (provided hosts): All endpoints must be reachable. No recovery/reconfiguration during runtime.
*
*
* @author Mark Paluch
* @since 4.1
* @deprecated since 5.2, use {@link io.lettuce.core.masterreplica.MasterReplica}
*/
@Deprecated
public class MasterSlave {
/**
* Open a new connection to a Redis Master-Slave server/servers using the supplied {@link RedisURI} and the supplied
* {@link RedisCodec codec} to encode/decode keys.
*
* This {@link MasterSlave} performs auto-discovery of nodes using either Redis Sentinel or Master/Slave. A {@link RedisURI}
* can point to either a master or a replica host.
*
*
* @param redisClient the Redis client.
* @param codec Use this codec to encode/decode keys and values, must not be {@code null}.
* @param redisURI the Redis server to connect to, must not be {@code null}.
* @param Key type.
* @param Value type.
* @return a new connection.
*/
public static StatefulRedisMasterSlaveConnection connect(RedisClient redisClient, RedisCodec codec,
RedisURI redisURI) {
LettuceAssert.notNull(redisClient, "RedisClient must not be null");
LettuceAssert.notNull(codec, "RedisCodec must not be null");
LettuceAssert.notNull(redisURI, "RedisURI must not be null");
return new MasterSlaveConnectionWrapper<>(MasterReplica.connect(redisClient, codec, redisURI));
}
/**
* Open asynchronously a new connection to a Redis Master-Slave server/servers using the supplied {@link RedisURI} and the
* supplied {@link RedisCodec codec} to encode/decode keys.
*
* This {@link MasterSlave} performs auto-discovery of nodes using either Redis Sentinel or Master/Slave. A {@link RedisURI}
* can point to either a master or a replica host.
*
*
* @param redisClient the Redis client.
* @param codec Use this codec to encode/decode keys and values, must not be {@code null}.
* @param redisURI the Redis server to connect to, must not be {@code null}.
* @param Key type.
* @param Value type.
* @return {@link CompletableFuture} that is notified once the connect is finished.
* @since
*/
public static CompletableFuture> connectAsync(RedisClient redisClient,
RedisCodec codec, RedisURI redisURI) {
return MasterReplica.connectAsync(redisClient, codec, redisURI).thenApply(MasterSlaveConnectionWrapper::new);
}
/**
* Open a new connection to a Redis Master-Slave server/servers using the supplied {@link RedisURI} and the supplied
* {@link RedisCodec codec} to encode/decode keys.
*
* This {@link MasterSlave} performs auto-discovery of nodes if the URI is a Redis Sentinel URI. Master/Slave URIs will be
* treated as static topology and no additional hosts are discovered in such case. Redis Standalone Master/Slave will
* discover the roles of the supplied {@link RedisURI URIs} and issue commands to the appropriate node.
*
*
* When using Redis Sentinel, ensure that {@link Iterable redisURIs} contains only a single entry as only the first URI is
* considered. {@link RedisURI} pointing to multiple Sentinels can be configured through
* {@link RedisURI.Builder#withSentinel}.
*
*
* @param redisClient the Redis client.
* @param codec Use this codec to encode/decode keys and values, must not be {@code null}.
* @param redisURIs the Redis server(s) to connect to, must not be {@code null}.
* @param Key type.
* @param Value type.
* @return a new connection.
*/
public static StatefulRedisMasterSlaveConnection connect(RedisClient redisClient, RedisCodec codec,
Iterable redisURIs) {
return new MasterSlaveConnectionWrapper<>(MasterReplica.connect(redisClient, codec, redisURIs));
}
/**
* Open asynchronously a new connection to a Redis Master-Slave server/servers using the supplied {@link RedisURI} and the
* supplied {@link RedisCodec codec} to encode/decode keys.
*
* This {@link MasterSlave} performs auto-discovery of nodes if the URI is a Redis Sentinel URI. Master/Slave URIs will be
* treated as static topology and no additional hosts are discovered in such case. Redis Standalone Master/Slave will
* discover the roles of the supplied {@link RedisURI URIs} and issue commands to the appropriate node.
*
*
* When using Redis Sentinel, ensure that {@link Iterable redisURIs} contains only a single entry as only the first URI is
* considered. {@link RedisURI} pointing to multiple Sentinels can be configured through
* {@link RedisURI.Builder#withSentinel}.
*
*
* @param redisClient the Redis client.
* @param codec Use this codec to encode/decode keys and values, must not be {@code null}.
* @param redisURIs the Redis server(s) to connect to, must not be {@code null}.
* @param Key type.
* @param Value type.
* @return {@link CompletableFuture} that is notified once the connect is finished.
*/
public static CompletableFuture> connectAsync(RedisClient redisClient,
RedisCodec codec, Iterable redisURIs) {
return MasterReplica.connectAsync(redisClient, codec, redisURIs).thenApply(MasterSlaveConnectionWrapper::new);
}
}