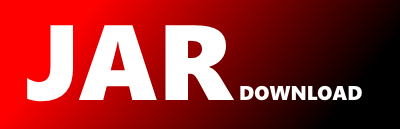
io.lettuce.core.output.ComplexData Maven / Gradle / Ivy
Show all versions of lettuce-core Show documentation
/*
* Copyright 2024, Redis Ltd. and Contributors
* All rights reserved.
*
* Licensed under the MIT License.
*/
package io.lettuce.core.output;
import java.util.List;
import java.util.Map;
import java.util.Set;
/**
* The base type of all complex data, collected by a {@link ComplexOutput}
*
* Commands typically result in simple types, however some of the commands could return complex nested structures. In these
* cases, and with the help of a {@link ComplexDataParser}, the data gathered by the {@link ComplexOutput} could be parsed to a
* domain object.
*
* An complex data object could only be an aggregate data type as per the
* RESP2 and
* RESP3 protocol definitions. Its
* contents, however, could be both the simple and aggregate data types.
*
* For RESP2 the only possible aggregation is an array. RESP2 commands could also return sets (obviously, by simply making sure
* the elements of the array are unique) or maps (by sending the keys as odd elements and their values as even elements in the
* right order one after another.
*
* For RESP3 all the three aggregate types are supported (and indicated with special characters when the result is returned by
* the server).
*
* Aggregate data types could also be nested by using the {@link ComplexData#storeObject(Object)} call.
*
* Implementations of this class override the {@link ComplexData#getDynamicSet()}, {@link ComplexData#getDynamicList()} and
* {@link ComplexData#getDynamicMap()} methods to return the data received in the server in a implementation of the Collections
* framework. If a given implementation could not do the conversion in a meaningful way an {@link UnsupportedOperationException}
* would be thrown.
*
* @see ComplexOutput
* @see ArrayComplexData
* @see SetComplexData
* @see MapComplexData
* @author Tihomir Mateev
* @since 6.5
*/
public abstract class ComplexData {
/**
* Store a long
value in the underlying data structure
*
* @param value the value to store
*/
public void store(long value) {
storeObject(value);
}
/**
* Store a double
value in the underlying data structure
*
* @param value the value to store
*/
public void store(double value) {
storeObject(value);
}
/**
* Store a boolean
value in the underlying data structure
*
* @param value the value to store
*/
public void store(boolean value) {
storeObject(value);
}
/**
* Store an {@link Object} value in the underlying data structure. This method should be used when nesting one instance of
* {@link ComplexData} inside another
*
* @param value the value to store
*/
public abstract void storeObject(Object value);
public void store(String value) {
storeObject(value);
}
/**
* Returns the aggregate data in a {@link List} form. If the underlying implementation could not convert the data structure
* to a {@link List} then an {@link UnsupportedOperationException} would be thrown.
*
* @return a {@link List} of {@link Object} values
*/
public List